AggregatedProcess¶
(Source code
, png
)
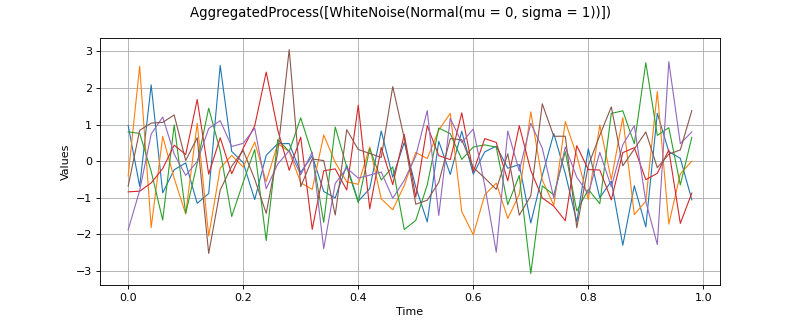
- class AggregatedProcess(*args)¶
Aggregation of several processes in one process.
- Parameters:
- collProcsequence of
Process
Collection of processes which all have the same input dimension.
- collProcsequence of
Notes
If we note
for
the collection of processes, where
for all
. Then the resulting aggregated process
where
. The mesh of the first process
has been assigned to the process
.
Examples
Create an aggregated process:
>>> import openturns as ot >>> myMesher = ot.IntervalMesher([5, 10]) >>> lowerbound = [0.0, 0.0] >>> upperBound = [2.0, 4.0] >>> myInterval = ot.Interval(lowerbound, upperBound) >>> myMesh = myMesher.build(myInterval) >>> myProcess1 = ot.WhiteNoise(ot.Normal(), myMesh) >>> myProcess2 = ot.WhiteNoise(ot.Triangular(), myMesh) >>> myAggregatedProcess = ot.AggregatedProcess([myProcess1, myProcess2])
Draw one realization:
>>> myGraph = myAggregatedProcess.getRealization().drawMarginal(0)
Methods
Accessor to the object's name.
Get a continuous realization.
Accessor to the covariance model.
Get the description of the process.
getFuture
(*args)Prediction of the
future iterations of the process.
Get the dimension of the domain
.
getMarginal
(*args)Accessor the marginal processes.
getMesh
()Get the mesh.
getName
()Accessor to the object's name.
Get the dimension of the domain
.
Get the collection of processes.
Get one realization of the aggregated process.
getSample
(size)Get
realizations of the process.
Get the time grid of observation of the process.
getTrend
()Accessor to the trend.
hasName
()Test if the object is named.
Test whether the process is composite or not.
isNormal
()Test whether the process is normal or not.
Test whether the process is stationary or not.
setDescription
(description)Set the description of the process.
setMesh
(mesh)Set the mesh.
setName
(name)Accessor to the object's name.
setProcessCollection
(coll)Set the collection of processes.
setTimeGrid
(timeGrid)Set the time grid of observation of the process.
- __init__(*args)¶
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getContinuousRealization()¶
Get a continuous realization.
- Returns:
- realization
Function
Each process of the collection is continuously realized on the common domain
.
- realization
- getCovarianceModel()¶
Accessor to the covariance model.
- Returns:
- cov_model
CovarianceModel
Covariance model, if any.
- cov_model
- getDescription()¶
Get the description of the process.
- Returns:
- description
Description
Description of the process.
- description
- getFuture(*args)¶
Prediction of the
future iterations of the process.
- Parameters:
- stepNumberint,
Number of future steps.
- sizeint,
, optional
Number of futures needed. Default is 1.
- stepNumberint,
- Returns:
- prediction
ProcessSample
orTimeSeries
future iterations of the process. If
, prediction is a
TimeSeries
. Otherwise, it is aProcessSample
.
- prediction
- getInputDimension()¶
Get the dimension of the domain
.
- Returns:
- nint
Dimension of the domain
:
.
- getMarginal(*args)¶
Accessor the marginal processes.
- Available usages:
getMarginal(index)
getMarginal(indices)
- Parameters:
- indexint
Index of the selected marginal process.
- indices
Indices
List of indices of the selected marginal processes.
Notes
The selected marginal processes are extracted if the list of indices does not mingle the processes of the initial collection: take care to extract all the marginal processes process by process. For example, if
,
and
then you can extract Indices([1,0,2,4,6]) but not Indices([1,2,0,4,6]).
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getOutputDimension()¶
Get the dimension of the domain
.
- Returns:
- dint
Dimension of the domain
.
- getProcessCollection()¶
Get the collection of processes.
- Returns:
- collProc
ProcessCollection
Collection of processes which all have the same input dimension.
- collProc
- getRealization()¶
Get one realization of the aggregated process.
- Returns:
- realization
Field
Each process of the collection is realized on the common mesh defined on
.
- realization
- getSample(size)¶
Get
realizations of the process.
- Parameters:
- nint,
Number of realizations of the process needed.
- nint,
- Returns:
- processSample
ProcessSample
realizations of the random process. A process sample is a collection of fields which share the same mesh
.
- processSample
- getTimeGrid()¶
Get the time grid of observation of the process.
- Returns:
- timeGrid
RegularGrid
Time grid of a process when the mesh associated to the process can be interpreted as a
RegularGrid
. We check if the vertices of the mesh are scalar and are regularly spaced inbut we don’t check if the connectivity of the mesh is conform to the one of a regular grid (without any hole and composed of ordered instants).
- timeGrid
- getTrend()¶
Accessor to the trend.
- Returns:
- trend
TrendTransform
Trend, if any.
- trend
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- isComposite()¶
Test whether the process is composite or not.
- Returns:
- isCompositebool
True if the process is composite (built upon a function and a process).
- isNormal()¶
Test whether the process is normal or not.
- Returns:
- isNormalbool
True if the process is normal.
Notes
A stochastic process is normal if all its finite dimensional joint distributions are normal, which means that for all
and
, with
, there is
and
such that:
where
,
and
and
is the symmetric matrix:
A Gaussian process is entirely defined by its mean function
and its covariance function
(or correlation function
).
- isStationary()¶
Test whether the process is stationary or not.
- Returns:
- isStationarybool
True if the process is stationary.
Notes
A process
is stationary if its distribution is invariant by translation:
,
,
, we have:
- setDescription(description)¶
Set the description of the process.
- Parameters:
- descriptionsequence of str
Description of the process.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
- setProcessCollection(coll)¶
Set the collection of processes.
- Parameters:
- collProcsequence of
Process
Collection of processes which all have the same input dimension.
- collProcsequence of
- setTimeGrid(timeGrid)¶
Set the time grid of observation of the process.
- Returns:
- timeGrid
RegularGrid
Time grid of observation of the process when the mesh associated to the process can be interpreted as a
RegularGrid
. We check if the vertices of the mesh are scalar and are regularly spaced inbut we don’t check if the connectivity of the mesh is conform to the one of a regular grid (without any hole and composed of ordered instants).
- timeGrid
Examples using the class¶
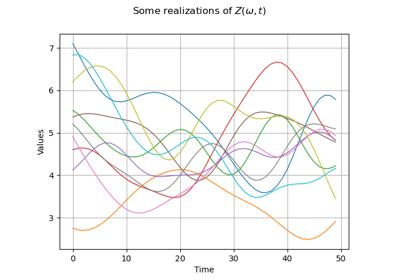
Create a process from random vectors and processes
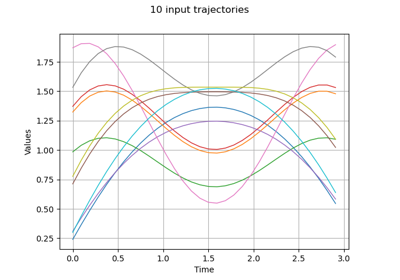
Estimate Sobol indices on a field to point function