KarhunenLoeveValidation¶
(Source code
, png
)
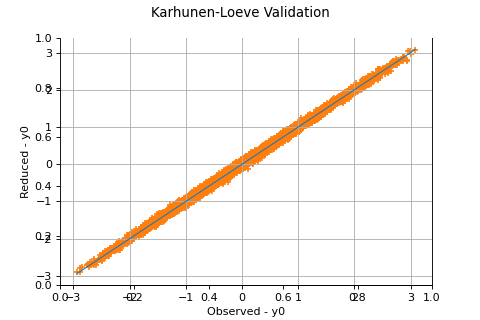
- class KarhunenLoeveValidation(*args)¶
Karhunen-Loeve decomposition validation services.
- Parameters:
- sample
ProcessSample
Observed (or learning) sample
- result
KarhunenLoeveResult
Decomposition result
- trend
TrendTransform
, optional Process trend, useful when the basis built using the covariance function from the space of trajectories is not well suited to approximate the mean function of the underlying process.
- sample
Examples
>>> import openturns as ot >>> N = 20 >>> interval = ot.Interval(-1.0, 1.0) >>> mesh = ot.IntervalMesher([N - 1]).build(interval) >>> covariance = ot.SquaredExponential() >>> process = ot.GaussianProcess(covariance, mesh) >>> sampleSize = 100 >>> processSample = process.getSample(sampleSize) >>> threshold = 1.0e-7 >>> algo = ot.KarhunenLoeveSVDAlgorithm(processSample, threshold) >>> algo.run() >>> klresult = algo.getResult() >>> validation = ot.KarhunenLoeveValidation(processSample, klresult)
Methods
Compute residual field.
Compute residual mean field.
Compute residual standard deviation field.
Plot the quality of representation of each observation.
Plot the weight of representation of each observation.
Plot a model vs metamodel graph for visual validation.
Accessor to the object's name.
getName
()Accessor to the object's name.
hasName
()Test if the object is named.
setName
(name)Accessor to the object's name.
- __init__(*args)¶
- computeResidual()¶
Compute residual field.
- Returns:
- processSampleResiduals
ProcessSample
The sample of residuals fields.
- processSampleResiduals
- computeResidualStandardDeviation()¶
Compute residual standard deviation field.
- Returns:
- stddev
Field
The residual standard deviation field.
- stddev
- drawObservationQuality()¶
Plot the quality of representation of each observation.
For each observation N we plot the quality of representation:
with
- Returns:
- graph
Graph
The visual validation graph.
- graph
- drawObservationWeight(k=0)¶
Plot the weight of representation of each observation.
For each observation we plot the weight according to the k-th mode using the projection of the observed sample:
- Parameters:
- kint,
, default=0
Mode index
- kint,
- Returns:
- graph
Graph
The visual validation graph.
- graph
- drawValidation()¶
Plot a model vs metamodel graph for visual validation.
For each marginal of the fields in the sample, we draw the value of the observed field depending on the value of the Karhunen-Loève reduced field (built from the observed field thanks to the projection and lift functions) at each node of the mesh. One graph is drawn for each marginal of the field.
- Returns:
- graph
GridLayout
The visual validation graph.
- graph
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
Examples using the class¶
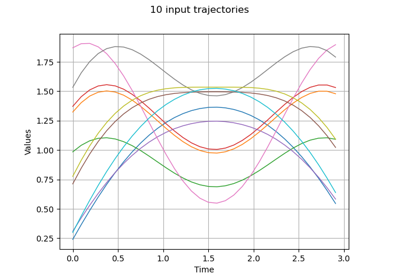
Estimate Sobol indices on a field to point function