SobolSimulationAlgorithm¶
(Source code
, png
)
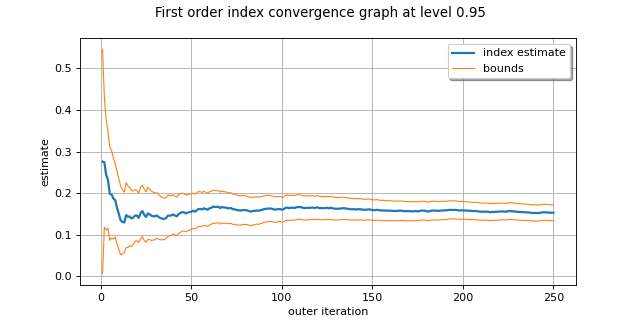
- class SobolSimulationAlgorithm(*args)¶
Sobol indices computation using iterative sampling.
The algorithm uses sampling of the distribution of the random vector
through the model
to iteratively estimate the Sobol indices.
At each iteration a fixed number
of replications inputs is generated. These inputs are evaluated by blocks of size
through the model
. Then the distribution of the indices (first and total order) is computed on this current replication sample. At the end of each iteration we update the global distribution of the indices.
- Parameters:
- X
Distribution
The random vector to study.
- f
Function
The function to study.
- estimator
SobolIndicesAlgorithm
The estimator of the indices.
- X
See also
Notes
The algorithm can operate on a multivariate model
, in this case it operates on aggregated indices.
Several estimators are available (Saltelli, Jansen, …).
Let us denote by
the number of input variables. For any
, let us denote by
(resp.
) the cumulative distribution function of the gaussian asymptotic distribution of the estimator of the Sobol’ first (resp. total) order indice. Let
be the level of the confidence interval and
the length of this confidence interval. The algorithms stops when, on all components, first and total order indices haved been estimated with enough precision.
The precision is said to be sufficient if the length of the
-level confidence interval is smaller than
:
for all
.
Examples
>>> import openturns as ot >>> ot.RandomGenerator.SetSeed(0) >>> distribution = ot.JointDistribution([ot.Uniform(-1.0, 1.0)] * 3) >>> model = ot.SymbolicFunction(['x1', 'x2', 'x3'], ['x1*x2+x3']) >>> estimator = ot.SaltelliSensitivityAlgorithm() >>> estimator.setUseAsymptoticDistribution(True) >>> algo = ot.SobolSimulationAlgorithm(distribution, model, estimator) >>> algo.setMaximumOuterSampling(25) # number of iterations >>> algo.setBlockSize(100) # size of Sobol experiment at each iteration >>> algo.setBatchSize(4) # number of points evaluated simultaneously >>> algo.setIndexQuantileLevel(0.05) # alpha >>> algo.setIndexQuantileEpsilon(1e-2) # epsilon >>> algo.run() >>> result = algo.getResult() >>> fo = result.getFirstOrderIndicesEstimate() >>> foDist = result.getFirstOrderIndicesDistribution()
Methods
Draw the first order Sobol index convergence at a given level.
Draw the total order Sobol index convergence at a given level.
Accessor to the batch size.
Accessor to the block size.
Accessor to the object's name.
Accessor to the convergence strategy.
Accessor to the batch size.
Sobol estimator accessor.
Accessor to the criterion operator.
Accessor to the quantile level.
Accessor to the maximum coefficient of variation.
Accessor to the maximum sample size.
Accessor to the maximum standard deviation.
Accessor to the maximum duration.
getName
()Accessor to the object's name.
Accessor to the result.
hasName
()Test if the object is named.
run
()Launch simulation.
setBatchSize
(replicationSize)Accessor to the batch size.
setBlockSize
(blockSize)Accessor to the block size.
setConvergenceStrategy
(convergenceStrategy)Accessor to the convergence strategy.
setEstimator
(estimator)Sobol estimator accessor.
setIndexQuantileEpsilon
(indexQuantileEpsilon)Accessor to the quantile tolerance.
setIndexQuantileLevel
(indexQuantileLevel)Accessor to the quantile level.
Accessor to the maximum coefficient of variation.
setMaximumOuterSampling
(maximumOuterSampling)Accessor to the maximum sample size.
Accessor to the maximum standard deviation.
setMaximumTimeDuration
(maximumTimeDuration)Accessor to the maximum duration.
setName
(name)Accessor to the object's name.
setProgressCallback
(*args)Set up a progress callback.
setStopCallback
(*args)Set up a stop callback.
- __init__(*args)¶
- drawFirstOrderIndexConvergence(*args)¶
Draw the first order Sobol index convergence at a given level.
- Parameters:
- marginalIndexint
Index of the random vector component to consider
- levelfloat, optional
The expectation convergence is drawn at this given confidence length level. By default level is 0.95.
- Returns:
- grapha
Graph
expectation convergence graph
- grapha
- drawTotalOrderIndexConvergence(*args)¶
Draw the total order Sobol index convergence at a given level.
- Parameters:
- marginalIndexint
Index of the random vector component to consider
- levelfloat, optional
The expectation convergence is drawn at this given confidence length level. By default level is 0.95.
- Returns:
- grapha
Graph
expectation convergence graph
- grapha
- getBatchSize()¶
Accessor to the batch size.
- Returns:
- batchSizeint
Number of points evaluated simultaneously.
- getBlockSize()¶
Accessor to the block size.
- Returns:
- blockSizeint
Number of terms in the probability simulation estimator grouped together. It is set by default to 1.
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getConvergenceStrategy()¶
Accessor to the convergence strategy.
- Returns:
- storage_strategy
HistoryStrategy
Storage strategy used to store the values of the probability estimator and its variance during the simulation algorithm.
- storage_strategy
- getDistribution()¶
Accessor to the batch size.
- Returns:
- distribution
Distribution
Distribution of the random variable.
- distribution
- getEstimator()¶
Sobol estimator accessor.
- Returns:
- estimator
SobolIndicesAlgorithm
The estimator of the indices.
- estimator
- getIndexQuantileEpsilon()¶
Accessor to the criterion operator.
- Returns:
- epsilonfloat
The quantile tolerance
- getIndexQuantileLevel()¶
Accessor to the quantile level.
- Returns:
- alphafloat
The quantile level.
- getMaximumCoefficientOfVariation()¶
Accessor to the maximum coefficient of variation.
- Returns:
- coefficientfloat
Maximum coefficient of variation of the simulated sample.
- getMaximumOuterSampling()¶
Accessor to the maximum sample size.
- Returns:
- outerSamplingint
Maximum number of groups of terms in the probability simulation estimator.
- getMaximumStandardDeviation()¶
Accessor to the maximum standard deviation.
- Returns:
- sigmafloat,
Maximum standard deviation of the estimator.
- sigmafloat,
- getMaximumTimeDuration()¶
Accessor to the maximum duration.
- Returns:
- maximumTimeDurationfloat
Maximum optimization duration in seconds.
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getResult()¶
Accessor to the result.
- Returns:
- result
SobolSimulationResult
The simulation result.
- result
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- run()¶
Launch simulation.
Notes
It launches the simulation on a sample of size at most outerSampling * blockSize, this sample being built by blocks of size blockSize. It allows one to use efficiently the distribution of the computation as well as it allows one to deal with a sample size
by a combination of blockSize and outerSampling.
- setBatchSize(replicationSize)¶
Accessor to the batch size.
- Parameters:
- batchSizeint
Number of points evaluated simultaneously.
- setBlockSize(blockSize)¶
Accessor to the block size.
- Parameters:
- blockSizeint,
Number of terms in the probability simulation estimator grouped together. It is set by default to 1.
- blockSizeint,
Notes
For Monte Carlo, LHS and Importance Sampling methods, this allows one to save space while allowing multithreading, when available we recommend to use the number of available CPUs; for the Directional Sampling, we recommend to set it to 1.
- setConvergenceStrategy(convergenceStrategy)¶
Accessor to the convergence strategy.
- Parameters:
- storage_strategy
HistoryStrategy
Storage strategy used to store the values of the probability estimator and its variance during the simulation algorithm.
- storage_strategy
- setEstimator(estimator)¶
Sobol estimator accessor.
- Parameters:
- estimator
SobolIndicesAlgorithm
The estimator of the indices.
- estimator
- setIndexQuantileEpsilon(indexQuantileEpsilon)¶
Accessor to the quantile tolerance.
- Parameters:
- epsilonfloat
The quantile tolerance
- setIndexQuantileLevel(indexQuantileLevel)¶
Accessor to the quantile level.
- Parameters:
- alphafloat
The quantile level.
- setMaximumCoefficientOfVariation(maximumCoefficientOfVariation)¶
Accessor to the maximum coefficient of variation.
- Parameters:
- coefficientfloat
Maximum coefficient of variation of the simulated sample.
- setMaximumOuterSampling(maximumOuterSampling)¶
Accessor to the maximum sample size.
- Parameters:
- outerSamplingint
Maximum number of groups of terms in the probability simulation estimator.
- setMaximumStandardDeviation(maximumStandardDeviation)¶
Accessor to the maximum standard deviation.
- Parameters:
- sigmafloat,
Maximum standard deviation of the estimator.
- sigmafloat,
- setMaximumTimeDuration(maximumTimeDuration)¶
Accessor to the maximum duration.
- Parameters:
- maximumTimeDurationfloat
Maximum optimization duration in seconds.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
- setProgressCallback(*args)¶
Set up a progress callback.
Can be used to programmatically report the progress of a simulation.
- Parameters:
- callbackcallable
Takes a float as argument as percentage of progress.
Examples
>>> import sys >>> import openturns as ot >>> experiment = ot.MonteCarloExperiment() >>> X = ot.RandomVector(ot.Normal()) >>> Y = ot.CompositeRandomVector(ot.SymbolicFunction(['X'], ['1.1*X']), X) >>> event = ot.ThresholdEvent(Y, ot.Less(), -2.0) >>> algo = ot.ProbabilitySimulationAlgorithm(event, experiment) >>> algo.setMaximumOuterSampling(100) >>> algo.setMaximumCoefficientOfVariation(-1.0) >>> def report_progress(progress): ... sys.stderr.write('-- progress=' + str(progress) + '%\n') >>> algo.setProgressCallback(report_progress) >>> algo.run()
- setStopCallback(*args)¶
Set up a stop callback.
Can be used to programmatically stop a simulation.
- Parameters:
- callbackcallable
Returns an int deciding whether to stop or continue.
Examples
Stop a Monte Carlo simulation algorithm using a time limit
>>> import openturns as ot >>> experiment = ot.MonteCarloExperiment() >>> X = ot.RandomVector(ot.Normal()) >>> Y = ot.CompositeRandomVector(ot.SymbolicFunction(['X'], ['1.1*X']), X) >>> event = ot.ThresholdEvent(Y, ot.Less(), -2.0) >>> algo = ot.ProbabilitySimulationAlgorithm(event, experiment) >>> algo.setMaximumOuterSampling(10000000) >>> algo.setMaximumCoefficientOfVariation(-1.0) >>> algo.setMaximumTimeDuration(0.1) >>> algo.run()