FloodModel¶
- class FloodModel(trueKs=30.0, trueZv=50.0, trueZm=55.0, distributionHdLow=True)¶
Data class for the flood model.
- Parameters:
- Lfloat, optional
Length of the river. The default is 5000.0.
- Bfloat, optional
Width of the river. The default is 300.0.
- trueKsfloat, optional
The true value of the Ks parameter. The default is 30.0.
- trueZvfloat, optional
The true value of the Zv parameter. The default is 50.0.
- trueZmfloat, optional
The true value of the Zm parameter. The default is 55.0.
- distributionHdLowbool, optional
If True, then the distribution of Hd is uniform in [2, 4] i.e the dyke is relatively low. Otherwise, the distribution of Hd is uniform in [7, 9] i.e the dyke is relatively high. The default is True.
- Attributes:
- dimint
The dimension of the problem: dim=4
- Q
TruncatedDistribution
ot.TruncatedDistribution(ot.Gumbel(558.0, 1013.0), 0.0, ot.TruncatedDistribution.LOWER)
- Ks
TruncatedDistribution
ot.TruncatedDistribution(ot.Normal(30.0, 7.5), 0.0, ot.TruncatedDistribution.LOWER)
- Zv
Uniform
ot.Uniform(49.0, 51.0)
- Zm
Uniform
ot.Uniform(54.0, 56.0)
- B
Uniform
Triangular(295.0, 300.0, 305.0)
- L
Uniform
ot.Triangular(4990.0, 5000.0, 5010.0)
- Hd
Uniform
ot.Uniform(54.0, 56.0)
- Zb
Uniform
The distribution depends on distributionHdLow.
- model
ParametricFunction
The flood model. The function has input dimension 4 and output dimension 1. More precisely, we have
and
. Its parameters are
.
- distribution
JointDistribution
The joint distribution of the input parameters.
- data
Sample
of size 10 and dimension 2 A data set which contains noisy observations of the flow rate (column 0) and the height (column 1).
Methods
getHeightModel
([L, B, Zb, Hd])Return the height model with corresponding input distribution
Examples
>>> from openturns.usecases import flood_model >>> # Load the flood model >>> fm = flood_model.FloodModel() >>> print(fm.data[:5]) [ Q ($m^3/s$) H (m) ] 0 : [ 130 0.59 ] 1 : [ 530 1.33 ] 2 : [ 960 2.03 ] 3 : [ 1400 2.72 ] 4 : [ 1830 2.83 ] >>> print("Inputs:", fm.model.getInputDescription()) Inputs: [Q, Ks, Zv, Zm, B, L, Zb, Hd] >>> print("Output:", fm.model.getOutputDescription()) Output: [H, S, C]
Get the height model.
>>> heightInputDistribution, heightModel = fm.getHeightModel() >>> print("Inputs:", heightModel.getInputDescription()) Inputs: [Q,Ks,Zv,Zm] >>> print("Outputs:", heightModel.getOutputDescription()) Outputs: [H]
Get the flooding model with high Hd scenario.
>>> fm = flood_model.FloodModel(distributionHdLow=False)
- __init__(trueKs=30.0, trueZv=50.0, trueZm=55.0, distributionHdLow=True)¶
- getHeightModel(L=5000.0, B=300.0, Zb=55.5, Hd=3.0)¶
Return the height model with corresponding input distribution
- Parameters:
- Lfloat, optional
The value of the river length. The default is 5000.0.
- Bfloat, optional
The value of the river width. The default is 300.0.
- Zbfloat, optional
The level (altitude) of the bank. The default is 55.5.
- Hdfloat, optional
The height of the dyke. The default is 3.0.
- Returns:
- heightInputDistribution
Distribution
The joint input distribution of (Q, Ks, Zv, Zm).
- heightModel
Function
The function with (Q, Ks, Zv, Zm) as input and (H) as output.
- heightInputDistribution
Examples using the class¶
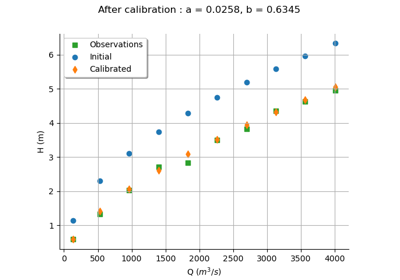
Calibrate a parametric model: a quick-start guide to calibration