Manipulate a time series
The objective here is to create and manipulate a time series.
A time series is a particular field where the mesh
1-d and regular, eg a time grid
.
It is possible to draw a time series, using interpolation between the values: see the use case on the Field.
A time series can be obtained as a realization of a multivariate stochastic process
of dimension
where
is discretized according to the regular grid
.
The values
of the time series are defined by:
A time series is stored in the TimeSeries
object that stores the regular time grid and the associated values.
import openturns as ot
import openturns.viewer as viewer
from matplotlib import pylab as plt
ot.Log.Show(ot.Log.NONE)
Create the RegularGrid
tMin = 0.0
timeStep = 0.1
N = 100
myTimeGrid = ot.RegularGrid(tMin, timeStep, N)
Case 1: Create a time series from a time grid and values.
Be careful that the number of steps of the time grid must correspond to the size of the values
myValues = ot.Normal(3).getSample(myTimeGrid.getVertices().getSize())
myTimeSeries = ot.TimeSeries(myTimeGrid, myValues)
myTimeSeries
class=TimeSeries name=Unnamed derived from=class=FieldImplementation name=Unnamed mesh=class=Mesh name=Unnamed dimension=1 vertices=class=Sample name=Unnamed implementation=class=SampleImplementation name=Unnamed size=100 dimension=1 description=[t] data=[[0],[0.1],[0.2],[0.3],[0.4],[0.5],[0.6],[0.7],[0.8],[0.9],[1],[1.1],[1.2],[1.3],[1.4],[1.5],[1.6],[1.7],[1.8],[1.9],[2],[2.1],[2.2],[2.3],[2.4],[2.5],[2.6],[2.7],[2.8],[2.9],[3],[3.1],[3.2],[3.3],[3.4],[3.5],[3.6],[3.7],[3.8],[3.9],[4],[4.1],[4.2],[4.3],[4.4],[4.5],[4.6],[4.7],[4.8],[4.9],[5],[5.1],[5.2],[5.3],[5.4],[5.5],[5.6],[5.7],[5.8],[5.9],[6],[6.1],[6.2],[6.3],[6.4],[6.5],[6.6],[6.7],[6.8],[6.9],[7],[7.1],[7.2],[7.3],[7.4],[7.5],[7.6],[7.7],[7.8],[7.9],[8],[8.1],[8.2],[8.3],[8.4],[8.5],[8.6],[8.7],[8.8],[8.9],[9],[9.1],[9.2],[9.3],[9.4],[9.5],[9.6],[9.7],[9.8],[9.9]] simplices=[[0,1],[1,2],[2,3],[3,4],[4,5],[5,6],[6,7],[7,8],[8,9],[9,10],[10,11],[11,12],[12,13],[13,14],[14,15],[15,16],[16,17],[17,18],[18,19],[19,20],[20,21],[21,22],[22,23],[23,24],[24,25],[25,26],[26,27],[27,28],[28,29],[29,30],[30,31],[31,32],[32,33],[33,34],[34,35],[35,36],[36,37],[37,38],[38,39],[39,40],[40,41],[41,42],[42,43],[43,44],[44,45],[45,46],[46,47],[47,48],[48,49],[49,50],[50,51],[51,52],[52,53],[53,54],[54,55],[55,56],[56,57],[57,58],[58,59],[59,60],[60,61],[61,62],[62,63],[63,64],[64,65],[65,66],[66,67],[67,68],[68,69],[69,70],[70,71],[71,72],[72,73],[73,74],[74,75],[75,76],[76,77],[77,78],[78,79],[79,80],[80,81],[81,82],[82,83],[83,84],[84,85],[85,86],[86,87],[87,88],[88,89],[89,90],[90,91],[91,92],[92,93],[93,94],[94,95],[95,96],[96,97],[97,98],[98,99]] values=class=Sample name=Normal implementation=class=SampleImplementation name=Normal size=100 dimension=3 description=[X0,X1,X2] data=[[0.52378,0.12611,0.393794],[-0.248139,-0.16677,0.214858],[0.0247934,0.138394,0.430279],[-1.61823,0.558478,-1.30886],[-0.139764,-0.546122,-0.567461],[0.205057,0.904138,0.547278],[0.788237,1.49744,-1.41748],[0.47696,-1.43288,2.02109],[1.23468,0.157697,0.940324],[0.117709,-1.14723,-1.32423],[0.498823,0.801346,0.900951],[-1.34579,-0.466627,0.246984],[-0.588016,0.250813,-0.859715],[-0.431791,-1.73008,-1.10509],[-1.22603,-0.70203,1.08883],[-0.700387,-1.66383,1.73256],[0.865208,0.270015,-0.27368],[-0.733251,0.521748,0.362264],[-0.123077,0.573721,-0.328373],[-0.10364,-0.52281,-1.68534],[0.195637,-0.0788806,-1.94656],[1.32949,-1.26065,-0.137471],[-0.349241,0.927261,1.02351],[-0.528963,1.2413,0.273859],[0.207583,1.16668,1.04363],[-2.38573,-1.65012,-0.416396],[2.13326,-0.43238,-0.483701],[-0.923412,-0.325859,-1.19977],[0.803572,0.524339,-0.0630568],[-0.835405,0.0402377,-0.452565],[0.0257213,0.368049,-0.841262],[-1.01146,-2.18025,-1.58033],[-0.449535,0.890842,0.0743256],[1.8438,-0.300223,-1.13582],[0.603846,-1.57444,-0.95201],[0.620044,-0.831148,0.179603],[-0.444416,0.959874,0.393199],[0.607432,-0.442113,0.170379],[0.296909,0.291782,0.977481],[-0.190001,0.250078,0.434109],[0.323441,1.75839,-0.023602],[-0.967543,-0.400737,-1.29578],[-0.312378,-0.944492,1.75581],[1.04865,-0.830397,-0.892176],[0.433775,-0.825645,-0.537977],[-0.102559,0.84464,-1.91496],[0.406466,0.321913,-0.305269],[0.488492,0.122734,0.305202],[-0.386326,0.0689214,0.313803],[0.677903,0.495358,-0.490069],[0.315441,-0.379322,0.785857],[-0.35019,0.264677,1.1554],[-0.455589,-0.559667,-0.662289],[-0.84981,-1.48909,-0.438836],[0.545473,-0.369104,0.962339],[0.770285,0.5617,-0.0453834],[-0.548109,-1.08238,0.471951],[0.50024,-1.40894,0.656795],[-1.05172,0.263069,-0.418894],[0.30767,-0.416239,1.35256],[-0.02062,1.30727,0.445701],[-0.428967,-2.15109,1.10333],[1.20183,-0.65679,-0.0034805],[-1.53118,1.56066,0.317831],[0.206523,1.45024,-1.4915],[-2.71262,-1.60122,-1.73553],[0.315804,-0.389312,-0.835347],[0.915355,-1.11271,0.586068],[0.57727,0.0729422,-0.441155],[-0.145206,1.00515,-1.07333],[1.89198,-0.216602,0.214305],[-0.503722,-0.345728,-1.40113],[0.921629,0.229499,0.777413],[-1.17868,0.132514,0.400826],[0.79873,0.175534,0.6878],[1.1929,1.95488,-0.475165],[-0.434939,-0.563952,2.23404],[-0.139733,-0.897931,0.198825],[-1.95284,-0.125675,1.59678],[1.05037,0.207933,-0.607134],[-0.652396,-0.666132,-1.07025],[0.225309,-1.13645,0.0128528],[0.681485,-0.0589669,0.62219],[-0.784616,-0.500505,-0.497468],[-0.59515,-0.597179,1.28317],[1.98506,0.0321232,0.469106],[0.0181719,-1.12972,0.70832],[0.309358,-0.161451,-0.0337435],[0.242744,-0.720621,0.605114],[-0.0771294,0.22276,-1.78651],[-0.52325,0.174664,-0.704638],[-0.988056,-0.361028,-1.76169],[0.313168,0.825269,0.432011],[1.20499,0.884502,0.117486],[-0.0337554,-0.092368,0.437182],[-0.0595837,-0.637973,-0.887564],[-0.337387,-0.0849012,-0.399771],[0.344743,-1.22959,0.656478],[-0.21913,-0.018154,0.365455],[0.793991,-1.21411,-1.73067]] start=0 timeStep=0.1 n=100
Case 2: Get a time series from a Process
myProcess = ot.WhiteNoise(ot.Normal(3), myTimeGrid)
myTimeSeries2 = myProcess.getRealization()
myTimeSeries2
| t | X0 | X1 | X2 |
0 | 0 | -1.670492 | -1.138823 | 1.317518 |
1 | 0.1 | 0.004916539 | 0.207453 | 0.5854116 |
2 | 0.2 | 0.6318718 | 0.3769744 | 1.288444 |
3 | 0.3 | -1.815059 | -1.064866 | -0.5376213 |
4 | 0.4 | 1.100027 | -1.608281 | 0.2590714 |
5 | 0.5 | 0.2505065 | -1.76158 | 0.6998717 |
6 | 0.6 | 0.9417183 | -0.488464 | -0.08899449 |
7 | 0.7 | 1.739529 | -0.8246544 | -0.0005616102 |
8 | 0.8 | 0.6088398 | 0.2800388 | 1.456377 |
9 | 0.9 | -0.2445882 | -0.9281551 | -0.8752327 |
10 | 1 | -0.9090887 | 0.09793622 | 0.5108138 |
11 | 1.1 | -0.2333036 | 2.977989 | -0.9998576 |
12 | 1.2 | -1.182387 | 1.048563 | 1.703911 |
13 | 1.3 | 0.8708819 | -1.284252 | 1.036737 |
14 | 1.4 | -0.5798028 | -1.859145 | 0.9250127 |
15 | 1.5 | 0.1757063 | 1.531778 | 0.5817077 |
16 | 1.6 | 1.024101 | 0.09670833 | 0.6195517 |
17 | 1.7 | 1.139809 | -0.4087134 | -0.2550477 |
18 | 1.8 | 0.5224197 | 0.3861234 | -2.075111 |
19 | 1.9 | 0.6156748 | 1.977506 | 0.06924619 |
20 | 2 | -1.367441 | -1.178709 | 1.05263 |
21 | 2.1 | 2.36118 | -1.022332 | 0.2285195 |
22 | 2.2 | -0.9436299 | 0.05570427 | 0.8479874 |
23 | 2.3 | 1.947942 | -1.768569 | 0.313241 |
24 | 2.4 | 1.393382 | -0.5917899 | -1.283321 |
25 | 2.5 | -0.4261665 | -2.275057 | -1.122716 |
26 | 2.6 | -0.9587 | 0.8365301 | -0.2694569 |
27 | 2.7 | 0.8057302 | 1.089693 | -0.6405182 |
28 | 2.8 | -2.792036 | -0.2587426 | -0.3865208 |
29 | 2.9 | 0.3161664 | 0.7604701 | -1.217716 |
30 | 3 | -0.1682398 | 0.5425786 | -1.366357 |
31 | 3.1 | 1.074819 | 0.01435018 | 0.1129411 |
32 | 3.2 | -0.755817 | -0.2352335 | -0.3526476 |
33 | 3.3 | 1.031261 | 0.1387743 | -0.1019698 |
34 | 3.4 | 0.1148558 | 1.307602 | 0.2750357 |
35 | 3.5 | 0.8908154 | -0.6283213 | 0.03249425 |
36 | 3.6 | 0.8022597 | -0.7750893 | 1.622367 |
37 | 3.7 | -0.8908726 | 0.1450516 | 1.288845 |
38 | 3.8 | 0.7490533 | -1.660132 | -0.2574172 |
39 | 3.9 | -0.6333344 | -0.01390061 | -0.5187067 |
40 | 4 | -0.7641469 | -0.8802884 | -1.04384 |
41 | 4.1 | 1.624155 | -0.08771599 | -0.2191296 |
42 | 4.2 | 1.651487 | 0.1178754 | -1.152759 |
43 | 4.3 | -0.6219183 | -1.12608 | 1.060989 |
44 | 4.4 | 1.310402 | -1.324693 | 2.232301 |
45 | 4.5 | -0.9766673 | -0.5351095 | -0.4692117 |
46 | 4.6 | 0.8752487 | -0.6614909 | 0.4248837 |
47 | 4.7 | 0.9250794 | 0.1116855 | -1.69762 |
48 | 4.8 | -1.71699 | 0.5622419 | -0.7709706 |
49 | 4.9 | -0.2774593 | -1.896383 | 2.738704 |
50 | 5 | 0.2583176 | 1.411942 | 0.5341219 |
51 | 5.1 | 0.813716 | 0.5418584 | -0.7792782 |
52 | 5.2 | -0.2821132 | 1.738024 | -0.3488722 |
53 | 5.3 | 0.5248662 | -0.4167478 | -0.4363704 |
54 | 5.4 | 1.231982 | -1.329614 | 0.4294014 |
55 | 5.5 | -0.1988925 | -0.5305423 | 0.1479477 |
56 | 5.6 | 1.087853 | -0.1887557 | 0.8494047 |
57 | 5.7 | 0.9088333 | 0.7830113 | 0.6364255 |
58 | 5.8 | 0.3660167 | 0.2228301 | 0.7101552 |
59 | 5.9 | 0.2647587 | -0.6881035 | -1.005743 |
60 | 6 | 0.2375102 | -1.817178 | -0.7518762 |
61 | 6.1 | -0.6579323 | 0.9981225 | -0.07624542 |
62 | 6.2 | -0.7847343 | -0.1382298 | -0.9096015 |
63 | 6.3 | -0.6038747 | -1.507997 | 1.923131 |
64 | 6.4 | -0.8878048 | -0.5890475 | 0.4536556 |
65 | 6.5 | 1.112655 | -0.9013951 | 0.7350295 |
66 | 6.6 | 0.1578329 | -1.229718 | -0.7125437 |
67 | 6.7 | 0.3872097 | -0.221006 | -0.6366688 |
68 | 6.8 | 0.1411499 | -1.382219 | -0.9600347 |
69 | 6.9 | -0.673238 | 1.35691 | -1.472505 |
70 | 7 | 0.290016 | -0.3173095 | 1.594927 |
71 | 7.1 | 0.427398 | 0.06673495 | 0.1377921 |
72 | 7.2 | 0.3715888 | -0.409588 | 0.4125253 |
73 | 7.3 | -0.7911313 | -0.3134569 | 0.683661 |
74 | 7.4 | 0.2432084 | 1.35115 | -1.572174 |
75 | 7.5 | 0.7591635 | -0.5428189 | 0.894484 |
76 | 7.6 | 1.496315 | 1.494005 | 0.07607672 |
77 | 7.7 | 3.032252 | -0.0697185 | 0.9362872 |
78 | 7.8 | 0.09534806 | 0.5547554 | -0.1597999 |
79 | 7.9 | -0.117537 | 0.8516132 | -2.280891 |
80 | 8 | 0.4156774 | 0.1686187 | -0.4769556 |
81 | 8.1 | -1.248859 | 0.5411939 | -2.166777 |
82 | 8.2 | 1.047143 | 0.4078612 | -1.554764 |
83 | 8.3 | -0.04557226 | 1.203224 | 0.9711234 |
84 | 8.4 | -0.9533762 | -0.5502569 | 0.7774226 |
85 | 8.5 | 0.4831444 | -0.1721776 | 1.008082 |
86 | 8.6 | 1.217844 | -0.4041445 | 1.793038 |
87 | 8.7 | -0.8329919 | 1.636185 | 0.6188379 |
88 | 8.8 | 0.9314921 | 0.3700222 | 1.658918 |
89 | 8.9 | -0.7589742 | -0.1231373 | -1.700695 |
90 | 9 | -0.8901343 | -0.8929079 | 0.1463011 |
91 | 9.1 | -0.01782797 | 0.1991584 | -1.521287 |
92 | 9.2 | 1.876469 | -1.655881 | 0.08516664 |
93 | 9.3 | 0.4022144 | -1.748718 | 0.5559068 |
94 | 9.4 | -1.22224 | 1.493979 | 1.512701 |
95 | 9.5 | -0.07158338 | 0.5902772 | 1.96161 |
96 | 9.6 | 0.1669381 | -1.754113 | 1.140951 |
97 | 9.7 | 0.6632621 | 1.00617 | -0.2992668 |
98 | 9.8 | -0.7329082 | -0.5431353 | 0.4164685 |
99 | 9.9 | 0.7069276 | 0.3009473 | 0.534359 |
Get the number of values of the time series
Get the dimension of the values observed at each time
myTimeSeries.getMesh().getDimension()
Get the value
at index 
i = 37
print("Xi = ", myTimeSeries.getValueAtIndex(i))
Xi = [0.607432,-0.442113,0.170379]
Get the time series at index
: 
i = 37
print("Xi = ", myTimeSeries[i])
Xi = [0.607432,-0.442113,0.170379]
Get a the marginal value at index
of the time series
i = 37
# get the time stamp:
print("ti = ", myTimeSeries.getTimeGrid().getValue(i))
# get the first component of the corresponding value :
print("Xi1 = ", myTimeSeries[i, 0])
ti = 3.7
Xi1 = 0.6074317802523269
Get all the values
of the time series
| X0 | X1 | X2 |
0 | 0.5237798 | 0.12611 | 0.3937941 |
1 | -0.2481388 | -0.1667702 | 0.2148585 |
2 | 0.02479338 | 0.1383943 | 0.430279 |
3 | -1.618226 | 0.5584776 | -1.30886 |
4 | -0.139764 | -0.5461219 | -0.5674607 |
5 | 0.2050574 | 0.9041375 | 0.5472782 |
6 | 0.7882369 | 1.49744 | -1.417477 |
7 | 0.4769603 | -1.432882 | 2.021089 |
8 | 1.23468 | 0.1576975 | 0.9403244 |
9 | 0.117709 | -1.147233 | -1.324232 |
10 | 0.4988229 | 0.801346 | 0.9009509 |
11 | -1.34579 | -0.466627 | 0.2469844 |
12 | -0.5880163 | 0.2508129 | -0.8597147 |
13 | -0.4317908 | -1.730083 | -1.105085 |
14 | -1.226028 | -0.7020297 | 1.088834 |
15 | -0.7003868 | -1.663834 | 1.732557 |
16 | 0.865208 | 0.2700146 | -0.2736801 |
17 | -0.7332508 | 0.5217478 | 0.3622642 |
18 | -0.1230767 | 0.5737211 | -0.3283734 |
19 | -0.1036404 | -0.5228103 | -1.685336 |
20 | 0.1956371 | -0.07888063 | -1.946558 |
21 | 1.329493 | -1.260652 | -0.1374706 |
22 | -0.3492414 | 0.9272612 | 1.023511 |
23 | -0.5289635 | 1.241303 | 0.2738588 |
24 | 0.2075833 | 1.166677 | 1.043628 |
25 | -2.385726 | -1.650125 | -0.4163961 |
26 | 2.133258 | -0.4323803 | -0.4837005 |
27 | -0.9234119 | -0.3258593 | -1.199766 |
28 | 0.8035719 | 0.5243393 | -0.06305676 |
29 | -0.835405 | 0.04023769 | -0.4525647 |
30 | 0.0257213 | 0.3680492 | -0.8412618 |
31 | -1.011461 | -2.180247 | -1.580328 |
32 | -0.4495348 | 0.8908425 | 0.07432561 |
33 | 1.843799 | -0.3002226 | -1.135818 |
34 | 0.6038459 | -1.574439 | -0.9520099 |
35 | 0.6200444 | -0.8311482 | 0.1796026 |
36 | -0.4444155 | 0.9598739 | 0.3931992 |
37 | 0.6074318 | -0.4421131 | 0.1703794 |
38 | 0.2969089 | 0.2917823 | 0.9774806 |
39 | -0.1900015 | 0.2500776 | 0.4341087 |
40 | 0.3234411 | 1.758392 | -0.02360201 |
41 | -0.9675434 | -0.4007368 | -1.295784 |
42 | -0.3123782 | -0.9444916 | 1.755809 |
43 | 1.048647 | -0.8303966 | -0.8921762 |
44 | 0.4337751 | -0.825645 | -0.5379767 |
45 | -0.1025594 | 0.8446397 | -1.914963 |
46 | 0.4064664 | 0.321913 | -0.3052688 |
47 | 0.4884916 | 0.1227336 | 0.3052021 |
48 | -0.3863256 | 0.06892144 | 0.3138032 |
49 | 0.6779029 | 0.4953577 | -0.490069 |
50 | 0.3154414 | -0.3793216 | 0.7858567 |
51 | -0.3501902 | 0.2646774 | 1.155399 |
52 | -0.4555892 | -0.5596673 | -0.6622893 |
53 | -0.8498098 | -1.489086 | -0.4388359 |
54 | 0.5454725 | -0.369104 | 0.9623389 |
55 | 0.7702848 | 0.5617002 | -0.04538344 |
56 | -0.5481091 | -1.082383 | 0.4719511 |
57 | 0.5002396 | -1.408936 | 0.6567949 |
58 | -1.051715 | 0.2630691 | -0.4188936 |
59 | 0.3076702 | -0.416239 | 1.352556 |
60 | -0.02062003 | 1.307273 | 0.4457012 |
61 | -0.4289673 | -2.151095 | 1.103334 |
62 | 1.201832 | -0.65679 | -0.003480504 |
63 | -1.531184 | 1.560664 | 0.3178313 |
64 | 0.2065234 | 1.45024 | -1.491504 |
65 | -2.712616 | -1.601219 | -1.735534 |
66 | 0.3158041 | -0.3893124 | -0.8353471 |
67 | 0.9153545 | -1.112714 | 0.5860682 |
68 | 0.5772697 | 0.07294216 | -0.4411553 |
69 | -0.1452064 | 1.005149 | -1.073327 |
70 | 1.891982 | -0.2166016 | 0.2143049 |
71 | -0.5037216 | -0.345728 | -1.401125 |
72 | 0.9216287 | 0.2294988 | 0.7774133 |
73 | -1.178678 | 0.1325139 | 0.4008258 |
74 | 0.7987302 | 0.1755338 | 0.6877997 |
75 | 1.1929 | 1.954883 | -0.4751648 |
76 | -0.434939 | -0.5639525 | 2.234044 |
77 | -0.1397326 | -0.8979314 | 0.1988251 |
78 | -1.952842 | -0.1256751 | 1.596785 |
79 | 1.050368 | 0.2079334 | -0.6071344 |
80 | -0.6523957 | -0.6661315 | -1.07025 |
81 | 0.2253092 | -1.136449 | 0.01285282 |
82 | 0.681485 | -0.05896693 | 0.62219 |
83 | -0.784616 | -0.5005054 | -0.4974685 |
84 | -0.5951495 | -0.5971793 | 1.283174 |
85 | 1.985056 | 0.03212315 | 0.4691064 |
86 | 0.01817188 | -1.129723 | 0.7083197 |
87 | 0.3093576 | -0.1614505 | -0.03374345 |
88 | 0.2427443 | -0.7206208 | 0.6051135 |
89 | -0.07712943 | 0.2227604 | -1.786512 |
90 | -0.5232505 | 0.1746638 | -0.7046384 |
91 | -0.9880562 | -0.3610281 | -1.761685 |
92 | 0.3131678 | 0.8252694 | 0.4320112 |
93 | 1.204991 | 0.8845017 | 0.1174865 |
94 | -0.03375537 | -0.09236796 | 0.4371824 |
95 | -0.05958368 | -0.6379726 | -0.8875642 |
96 | -0.3373875 | -0.08490124 | -0.3997714 |
97 | 0.3447429 | -1.229588 | 0.6564776 |
98 | -0.2191304 | -0.01815395 | 0.3654549 |
99 | 0.7939912 | -1.214108 | -1.730674 |
Compute the temporal Mean
It corresponds to the mean of the values of the time series
myTimeSeries.getInputMean()
class=Point name=Unnamed dimension=3 values=[0.00695924,-0.100262,-0.0396681]
Draw the marginal
of the time series using linear interpolation
graph = myTimeSeries.drawMarginal(0)
view = viewer.View(graph)
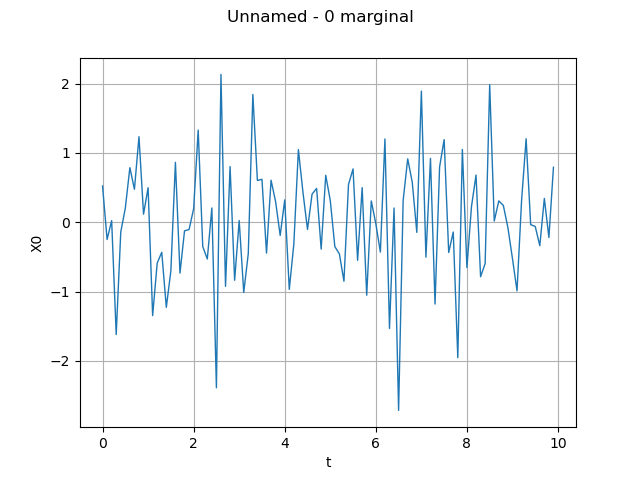
With no interpolation
graph = myTimeSeries.drawMarginal(0, False)
view = viewer.View(graph)
plt.show()