Note
Go to the end to download the full example code.
Subset Sampling¶
The objective is to evaluate a probability from the Subset sampling technique.
We consider the function defined by:
and the input random vector which follows a Normal distribution with independent components, and identical marginals with 0.25 mean and unit variance:
We want to evaluate the probability:
First, import the python modules:
import openturns as ot
from openturns.viewer import View
Create the probabilistic model
¶
Create the input random vector :
X = ot.RandomVector(ot.Normal([0.25] * 2, [1] * 2, ot.IdentityMatrix(2)))
Create the function :
g = ot.SymbolicFunction(["x1", "x2"], ["20-(x1-x2)^2-8*(x1+x2-4)^3"])
print("function g: ", g)
function g: [x1,x2]->[20-(x1-x2)^2-8*(x1+x2-4)^3]
Create the output random vector :
Y = ot.CompositeRandomVector(g, X)
Create the event
¶
myEvent = ot.ThresholdEvent(Y, ot.LessOrEqual(), 0.0)
Evaluate the probability with the subset sampling technique¶
algo = ot.SubsetSampling(myEvent)
In order to get all the inputs and outputs that realize the event, you have to mention it now:
algo.setKeepSample(True)
Now you can run the algorithm!
algo.run()
result = algo.getResult()
proba = result.getProbabilityEstimate()
print("Proba Subset = ", proba)
print("Current coefficient of variation = ", result.getCoefficientOfVariation())
Proba Subset = 0.0003932849999999996
Current coefficient of variation = 0.08730685574998505
The length of the confidence interval of level is:
length95 = result.getConfidenceLength()
print("Confidence length (0.95) = ", result.getConfidenceLength())
Confidence length (0.95) = 0.00013459651562543358
which enables to build the confidence interval:
print(
"Confidence interval (0.95) = [",
proba - length95 / 2,
", ",
proba + length95 / 2,
"]",
)
Confidence interval (0.95) = [ 0.00032598674218728283 , 0.0004605832578127164 ]
You can also get the successive thresholds used by the algorithm:
levels = algo.getThresholdPerStep()
print("Levels of g = ", levels)
Levels of g = [56.4629,18.3376,8.10199,0]
Draw the subset samples used by the algorithm¶
You can get the number of steps with:
Ns = algo.getStepsNumber()
print("Number of steps= ", Ns)
Number of steps= 4
Get all the inputs where was evaluated at each step
list_subSamples = list()
for step in range(Ns):
list_subSamples.append(algo.getInputSample(step))
The following graph draws each subset sample and the frontier where
is the threshold at the step
:
graph = ot.Graph()
graph.setAxes(True)
graph.setGrid(True)
graph.setTitle("Subset sampling: samples")
graph.setXTitle(r"$x_1$")
graph.setYTitle(r"$x_2$")
graph.setLegendPosition("lower left")
Add all the subset samples:
for i in range(Ns):
cloud = ot.Cloud(list_subSamples[i])
# cloud.setPointStyle("dot")
graph.add(cloud)
col = ot.Drawable().BuildDefaultPalette(Ns)
graph.setColors(col)
Add the frontiers where
is the threshold at the step
:
gIsoLines = g.draw([-3] * 2, [5] * 2, [128] * 2)
dr = gIsoLines.getDrawable(0)
for i in range(levels.getSize()):
dr.setLevels([levels[i]])
dr.setLineStyle("solid")
dr.setLegend(r"$g(X) = $" + str(round(levels[i], 2)))
dr.setLineWidth(3)
dr.setColor(col[i])
graph.add(dr)
_ = View(graph)

Draw the frontiers only¶
The following graph enables to understand the progresison of the algorithm:
graph = ot.Graph()
graph.setAxes(True)
graph.setGrid(True)
dr = gIsoLines.getDrawable(0)
for i in range(levels.getSize()):
dr.setLevels([levels[i]])
dr.setLineStyle("solid")
dr.setLegend(r"$g(X) = $" + str(round(levels[i], 2)))
dr.setLineWidth(3)
graph.add(dr)
graph.setColors(col)
graph.setLegendPosition("lower left")
graph.setTitle("Subset sampling: thresholds")
graph.setXTitle(r"$x_1$")
graph.setYTitle(r"$x_2$")
_ = View(graph)

Get all the input and output points that realized the event¶
The following lines are possible only if you have mentioned that you wanted to keep samples with the method algo.setKeepSample(True)
select = ot.SubsetSampling.EVENT1 # points that realize the event
step = Ns - 1 # get the working sample from last iteration
inputEventSample = algo.getInputSample(step, select)
outputEventSample = algo.getOutputSample(step, select)
print("Number of event realizations = ", inputEventSample.getSize())
Number of event realizations = 3925
Draw them! They are all in the event space.
graph = ot.Graph()
graph.setAxes(True)
graph.setGrid(True)
cloud = ot.Cloud(inputEventSample)
cloud.setPointStyle("bullet")
graph.add(cloud)
gIsoLines = g.draw([-3] * 2, [5] * 2, [1000] * 2)
dr = gIsoLines.getDrawable(0)
dr.setLevels([0.0])
dr.setColor("red")
graph.add(dr)
_ = View(graph)
View.ShowAll()
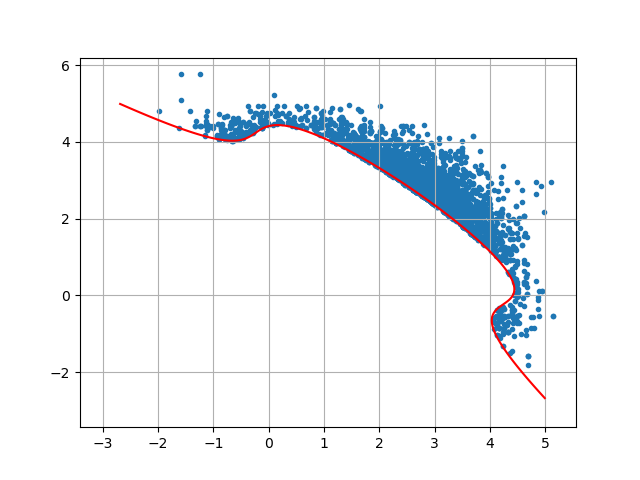