LaguerreFactory¶
(Source code
, png
)
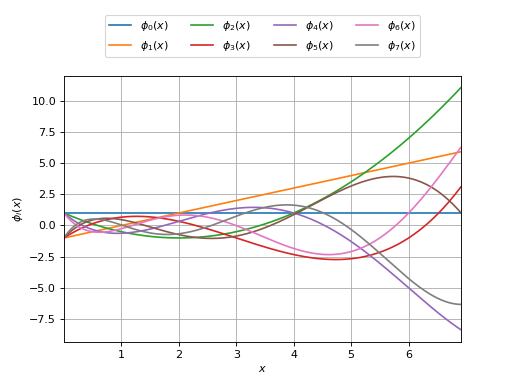
- class LaguerreFactory(*args)¶
Laguerre specific orthonormal univariate polynomial family.
For the
Gamma
distribution.- Parameters:
- kfloat
If parameters_set == ot.JacobiFactory.PROBABILITY: default shape parameter
of the
Gamma
distribution.If parameters_set == ot.JacobiFactory.ANALYSIS: alternative shape parameter
of the
Gamma
distribution.- parameters_setint, optional
Integer telling which parameters set is used for defining the distribution (amongst ot.LaguerreFactory.ANALYSIS, ot.LaguerreFactory.PROBABILITY).
Methods
build
(degree)Build the
-th order orthogonal univariate polynomial.
buildCoefficients
(degree)Build the
-th order orthogonal univariate polynomial coefficients.
Build the recurrence coefficients.
Accessor to the object's name.
getK
()Accessor to the alternative shape parameter
.
Accessor to the associated probability measure.
getName
()Accessor to the object's name.
Build the
-th order quadrature scheme.
Accessor to the recurrence coefficients of the
-th order.
getRoots
(n)Accessor to the recurrence coefficients of the
-th order.
hasName
()Test if the object is named.
setName
(name)Accessor to the object's name.
Notes
Any sequence of orthogonal polynomials has a recurrence formula relating any three consecutive polynomials as follows:
The recurrence coefficients for the Laguerre polynomials come analytically and read:
where
is the alternative shape parameter of the
Gamma
distribution, and:Examples
>>> import openturns as ot >>> polynomial_factory = ot.LaguerreFactory() >>> for i in range(3): ... print(polynomial_factory.build(i)) 1 -1 + X 1 - 2 * X + 0.5 * X^2
- __init__(*args)¶
- build(degree)¶
Build the
-th order orthogonal univariate polynomial.
- Parameters:
- kint,
Polynomial order.
- kint,
- Returns:
- polynomial
OrthogonalUniVariatePolynomial
Requested orthogonal univariate polynomial.
- polynomial
Examples
>>> import openturns as ot >>> polynomial_factory = ot.HermiteFactory() >>> print(polynomial_factory.build(2)) -0.707107 + 0.707107 * X^2
- buildCoefficients(degree)¶
Build the
-th order orthogonal univariate polynomial coefficients.
- Parameters:
- kint,
Polynomial order.
- kint,
- Returns:
- coefficients
Point
Coefficients of the requested orthogonal univariate polynomial.
- coefficients
Examples
>>> import openturns as ot >>> polynomial_factory = ot.HermiteFactory() >>> print(polynomial_factory.buildCoefficients(2)) [-0.707107,0,0.707107]
- buildRecurrenceCoefficientsCollection(degree)¶
Build the recurrence coefficients.
Build the recurrence coefficients of the orthogonal univariate polynomial family up to the
-th order.
- Parameters:
- kint,
Polynomial order.
- kint,
- Returns:
- recurrence_coefficientslist of
Point
All the tecurrence coefficients up to the requested order.
- recurrence_coefficientslist of
Examples
>>> import openturns as ot >>> polynomial_factory = ot.HermiteFactory() >>> print(polynomial_factory.buildRecurrenceCoefficientsCollection(2)) 0 : [ 1 0 0 ] 1 : [ 0.707107 0 -0.707107 ]
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getK()¶
Accessor to the alternative shape parameter
.
Of the
Gamma
distribution.- Returns:
- k_afloat
Alternative shape parameter
of the
Gamma
distribution.
- getMeasure()¶
Accessor to the associated probability measure.
- Returns:
- measure
Distribution
The associated probability measure (according to which the polynomials are orthogonal).
- measure
Notes
Two polynomials P and Q are orthogonal with respect to the probability measure
if and only if their dot product:
where
and
.
Examples
>>> import openturns as ot >>> polynomial_factory = ot.HermiteFactory() >>> print(polynomial_factory.getMeasure()) Normal(mu = 0, sigma = 1)
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getNodesAndWeights(n)¶
Build the
-th order quadrature scheme.
Associated with the orthogonal univariate polynomials family.
- Parameters:
- kint,
Polynomial order.
- kint,
- Returns:
Examples
>>> import openturns as ot >>> polynomial_factory = ot.HermiteFactory() >>> nodes, weights = polynomial_factory.getNodesAndWeights(3) >>> print(nodes) [-1.73205,...,1.73205] >>> print(weights) [0.166667,0.666667,0.166667]
- getRecurrenceCoefficients(n)¶
Accessor to the recurrence coefficients of the
-th order.
Of the orthogonal univariate polynomial.
- Parameters:
- kint,
Polynomial order.
- kint,
- Returns:
- recurrence_coefficients
Point
The recurrence coefficients of the
-th order orthogonal univariate polynomial.
- recurrence_coefficients
Notes
Any sequence of orthogonal polynomials has a recurrence formula relating any three consecutive polynomials as follows:
Examples
>>> import openturns as ot >>> polynomial_factory = ot.HermiteFactory() >>> print(polynomial_factory.getRecurrenceCoefficients(3)) [0.5,0,-0.866025]
- getRoots(n)¶
Accessor to the recurrence coefficients of the
-th order.
Of the orthogonal univariate polynomial.
- Parameters:
- kint,
Polynomial order.
- kint,
- Returns:
- roots
Point
The roots of the
-th order orthogonal univariate polynomial.
- roots
Examples
>>> import openturns as ot >>> polynomial_factory = ot.HermiteFactory() >>> print(polynomial_factory.getRoots(3)) [-1.73205,...,1.73205]
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.