LevelSetMesher¶
(Source code
, png
)
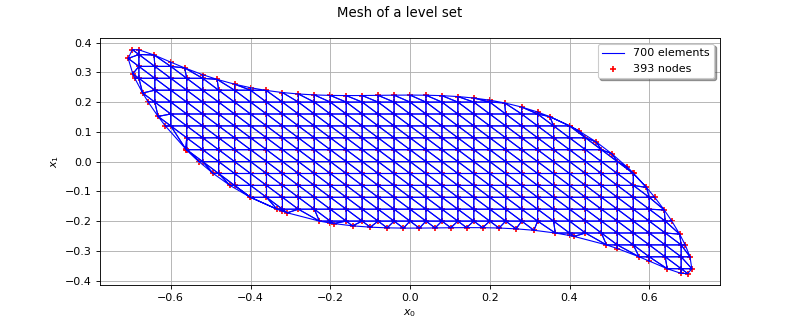
- class LevelSetMesher(*args)¶
Creation of mesh from a level set.
- Parameters:
- discretizationsequence of int
Discretization of the level set bounding box.
- solver
OptimizationAlgorithm
, optional Optimization solver used to project the vertices onto the level set. It must be able to solve nearest point problems. Default is
AbdoRackwitz
.
Methods
build
(*args)Build the mesh of level set type.
Accessor to the object's name.
Accessor to the discretization.
getName
()Accessor to the object's name.
Accessor to the optimization solver.
hasName
()Test if the object is named.
setDiscretization
(discretization)Accessor to the discretization.
setName
(name)Accessor to the object's name.
setOptimizationAlgorithm
(solver)Accessor to the optimization solver.
Notes
The meshing algorithm is based on the
IntervalMesher
class. First, the bounding box of the level set (provided by the user or automatically computed) is meshed. Then, all the simplices with all vertices outside of the level set are rejected, while the simplices with all vertices inside of the level set are kept. The remaining simplices are adapted the following way:The mean point of the vertices inside of the level set is computed
Each vertex outside of the level set is projected onto the level set using a linear interpolation
If the project flag is True, then the projection is refined using an optimization solver.
Examples
Create a mesh:
>>> import openturns as ot >>> mesher = ot.LevelSetMesher([5, 10]) >>> level = 1.0 >>> function = ot.SymbolicFunction(['x0', 'x1'], ['x0^2+x1^2']) >>> levelSet = ot.LevelSet(function, ot.LessOrEqual(), level) >>> mesh = mesher.build(levelSet, ot.Interval([-2.0]*2, [2.0]*2))
- __init__(*args)¶
- build(*args)¶
Build the mesh of level set type.
- Parameters:
- levelSet
LevelSet
The level set to be meshed, of dimension equal to the dimension of discretization.
- boundingBox
Interval
The bounding box used to mesh the level set.
- projectbool
Flag to tell if the vertices outside of the level set of a simplex partially included into the level set have to be projected onto the level set. Default is True.
- levelSet
- Returns:
- mesh
Mesh
The mesh built.
- mesh
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getDiscretization()¶
Accessor to the discretization.
- Returns:
- discretization
Indices
Discretization of the bounding box of the level sets.
- discretization
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getOptimizationAlgorithm()¶
Accessor to the optimization solver.
- Returns:
- solver
OptimizationAlgorithm
The optimization solver used to project vertices onto the level set.
- solver
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- setDiscretization(discretization)¶
Accessor to the discretization.
- Parameters:
- discretizationsequence of int
Discretization of the bounding box of the level sets.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
- setOptimizationAlgorithm(solver)¶
Accessor to the optimization solver.
- Parameters:
- solver
OptimizationAlgorithm
The optimization solver used to project vertices onto the level set.
- solver