PointToFieldFunction¶
- class PointToFieldFunction(*args)¶
Function mapping a point into a field.
- Parameters:
- inputDimint,
Dimension
of the input vector
- outputMesh
Mesh
The output mesh
- outputDimint,
Dimension
of the output field
- inputDimint,
Notes
Point to field functions act on points to produce fields:
with
a mesh of
.
A field is represented by a collection
of elements of
where
is a vertex of
and
the associated value in
.
The two first constructors build an object which evaluation operator is not defined (it throws a NotYetImplementedException). The instantiation of such an object is used to extract an actual
PointToFieldFunction
from aStudy
.Examples
>>> import openturns as ot
Use the class
OpenTURNSPythonPointToFieldFunction
to create a function that acts a vectorof dimension
and returns a field defined by:
the mesh that discretizes
into 10 regular intervals of length 0.1 (
)
the value associated to the vertex number
is
(
)
Using the class
OpenTURNSPythonFieldToPointFunction
allows one to define a persistent state between the evaluations of the function.>>> class FUNC(ot.OpenTURNSPythonPointToFieldFunction): ... def __init__(self): ... mesh = ot.RegularGrid(0.0, 0.1, 11) ... super(FUNC, self).__init__(2, mesh, 2) ... self.setInputDescription(['R', 'S']) ... self.setOutputDescription(['T', 'U']) ... def _exec(self, X): ... size = self.getOutputMesh().getVerticesNumber() ... Y = [ot.Point(X)*i for i in range(size)] ... return Y >>> F = FUNC()
Create the associated PointToFieldFunction:
>>> myFunc = ot.PointToFieldFunction(F)
It is also possible to create a PointToFieldFunction from a python function as follows:
>>> mesh = ot.RegularGrid(0.0, 0.1, 11) >>> def myPyFunc(X): ... size = 11 ... Y = [ot.Point(X)*i for i in range(size)] ... return Y >>> inputDim = 2 >>> outputDim = 2 >>> myFunc = ot.PythonPointToFieldFunction(inputDim, mesh, outputDim, myPyFunc)
Evaluation the function on a vector:
>>> Yfield = myFunc([1.1, 2.2])
Methods
Get the number of calls of the function.
Accessor to the object's name.
getId
()Accessor to the object's id.
Accessor to the underlying implementation.
Get the description of the input vector.
Get the dimension of the input vector.
getMarginal
(*args)Get the marginal(s) at given indice(s).
getName
()Accessor to the object's name.
Get the description of the output field values.
Get the dimension of the output field values.
Get the output mesh.
setInputDescription
(inputDescription)Set the description of the input vector.
setName
(name)Accessor to the object's name.
setOutputDescription
(outputDescription)Set the description of the output field values.
- __init__(*args)¶
- getCallsNumber()¶
Get the number of calls of the function.
- Returns:
- callsNumberint
Counts the number of times the function has been called since its creation.
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getId()¶
Accessor to the object’s id.
- Returns:
- idint
Internal unique identifier.
- getImplementation()¶
Accessor to the underlying implementation.
- Returns:
- implImplementation
A copy of the underlying implementation object.
- getInputDescription()¶
Get the description of the input vector.
- Returns:
- inputDescription
Description
Description of the input vector.
- inputDescription
- getInputDimension()¶
Get the dimension of the input vector.
- Returns:
- dint
Dimension
of the input vector.
- getMarginal(*args)¶
Get the marginal(s) at given indice(s).
- Parameters:
- iint or list of ints,
Indice(s) of the marginal(s) to be extracted.
- iint or list of ints,
- Returns:
- function
PointToFieldFunction
The initial function restricted to the concerned marginal(s) at the indice(s)
.
- function
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getOutputDescription()¶
Get the description of the output field values.
- Returns:
- outputDescription
Description
Description of the output field values.
- outputDescription
- getOutputDimension()¶
Get the dimension of the output field values.
- Returns:
- d’int
Dimension
of the output field values.
- setInputDescription(inputDescription)¶
Set the description of the input vector.
- Parameters:
- inputDescriptionsequence of str
Description of the input vector.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
- setOutputDescription(outputDescription)¶
Set the description of the output field values.
- Parameters:
- outputDescriptionsequence of str
Description of the output field values.
Examples using the class¶
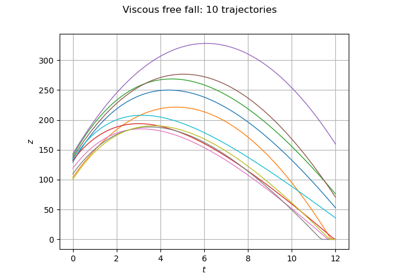
Define a function with a field output: the viscous free fall example