TNC¶
- class TNC(*args)¶
Truncated Newton Constrained solver.
Truncated-Newton method Non-linear optimizer. This solver uses no derivative information and only supports bound constraints.
- Available constructors:
TNC(problem)
TNC(problem, scale, offset, maxCGit, eta, stepmx, accuracy, fmin, rescale)
- Parameters:
- problem
OptimizationProblem
Optimization problem to solve.
- scalesequence of float
Scaling factors to apply to each variable.
- offsetsequence of float
Constant to subtract to each variable.
- maxCGitint
Maximum number of hessian*vector evaluation per main iteration.
- etafloat
Severity of the line search.
- stepmxfloat
Maximum step for the line search, may be increased during call.
- accuracyfloat
Relative precision for finite difference calculations.
- fminfloat
Minimum function value estimate.
- rescalefloat
f scaling factor (in log10) used to trigger f value rescaling.
- problem
Methods
Accessor to accuracy parameter.
Accessor to check status flag.
Accessor to the object's name.
getEta
()Accessor to eta parameter.
getFmin
()Accessor to fmin parameter.
Accessor to maxCGit parameter.
Accessor to maximum allowed absolute error.
Accessor to maximum allowed number of calls.
Accessor to maximum allowed constraint error.
Accessor to maximum allowed number of iterations.
Accessor to maximum allowed relative error.
Accessor to maximum allowed residual error.
Accessor to the maximum duration.
getName
()Accessor to the object's name.
Accessor to offset parameter.
Accessor to optimization problem.
Accessor to rescale parameter.
Accessor to optimization result.
getScale
()Accessor to scale parameter.
Accessor to starting point.
Accessor to stepmx parameter.
hasName
()Test if the object is named.
run
()Launch the optimization.
setAccuracy
(accuracy)Accessor to accuracy parameter.
setCheckStatus
(checkStatus)Accessor to check status flag.
setEta
(eta)Accessor to eta parameter.
setFmin
(fmin)Accessor to fmin parameter.
setMaxCGit
(maxCGit)Accessor to maxCGit parameter.
setMaximumAbsoluteError
(maximumAbsoluteError)Accessor to maximum allowed absolute error.
setMaximumCallsNumber
(maximumCallsNumber)Accessor to maximum allowed number of calls
setMaximumConstraintError
(maximumConstraintError)Accessor to maximum allowed constraint error.
setMaximumIterationNumber
(maximumIterationNumber)Accessor to maximum allowed number of iterations.
setMaximumRelativeError
(maximumRelativeError)Accessor to maximum allowed relative error.
setMaximumResidualError
(maximumResidualError)Accessor to maximum allowed residual error.
setMaximumTimeDuration
(maximumTime)Accessor to the maximum duration.
setName
(name)Accessor to the object's name.
setOffset
(offset)Accessor to offset parameter.
setProblem
(problem)Accessor to optimization problem.
setProgressCallback
(*args)Set up a progress callback.
setRescale
(rescale)Accessor to rescale parameter.
setResult
(result)Accessor to optimization result.
setScale
(scale)Accessor to scale parameter.
setStartingPoint
(startingPoint)Accessor to starting point.
setStepmx
(stepmx)Accessor to stepmx parameter.
setStopCallback
(*args)Set up a stop callback.
See also
Examples
>>> import openturns as ot >>> model = ot.SymbolicFunction(['E', 'F', 'L', 'I'], ['-F*L^3/(3*E*I)']) >>> bounds = ot.Interval([1.0]*4, [2.0]*4) >>> problem = ot.OptimizationProblem(model, ot.Function(), ot.Function(), bounds) >>> algo = ot.TNC(problem) >>> algo.setStartingPoint([1.0] * 4) >>> algo.run() >>> result = algo.getResult()
- __init__(*args)¶
- getAccuracy()¶
Accessor to accuracy parameter.
- Returns:
- accuracyfloat
Relative precision for finite difference calculations
if <= machine_precision, set to sqrt(machine_precision).
- getCheckStatus()¶
Accessor to check status flag.
- Returns:
- checkStatusbool
Whether to check the termination status. If set to False,
run()
will not throw an exception if the algorithm does not fully converge and will allow one to still find a feasible candidate.
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getEta()¶
Accessor to eta parameter.
- Returns:
- etafloat
Severity of the line search.
if < 0 or > 1, set to 0.25.
- getFmin()¶
Accessor to fmin parameter.
- Returns:
- fminfloat
Minimum function value estimate.
- getMaxCGit()¶
Accessor to maxCGit parameter.
- Returns:
- maxCGitint
Maximum number of hessian*vector evaluation per main iteration
if maxCGit = 0, the direction chosen is -gradient
if maxCGit < 0, maxCGit is set to max(1,min(50,n/2)).
- getMaximumAbsoluteError()¶
Accessor to maximum allowed absolute error.
- Returns:
- maximumAbsoluteErrorfloat
Maximum allowed absolute error, where the absolute error is defined by
where
and
are two consecutive approximations of the optimum.
- getMaximumCallsNumber()¶
Accessor to maximum allowed number of calls.
- Returns:
- maximumEvaluationNumberint
Maximum allowed number of direct objective function calls through the () operator. Does not take into account eventual indirect calls through finite difference gradient calls.
- getMaximumConstraintError()¶
Accessor to maximum allowed constraint error.
- Returns:
- maximumConstraintErrorfloat
Maximum allowed constraint error, where the constraint error is defined by
where
is the current approximation of the optimum and
is the function that gathers all the equality and inequality constraints (violated values only)
- getMaximumIterationNumber()¶
Accessor to maximum allowed number of iterations.
- Returns:
- maximumIterationNumberint
Maximum allowed number of iterations.
- getMaximumRelativeError()¶
Accessor to maximum allowed relative error.
- Returns:
- maximumRelativeErrorfloat
Maximum allowed relative error, where the relative error is defined by
if
, else
.
- getMaximumResidualError()¶
Accessor to maximum allowed residual error.
- Returns:
- maximumResidualErrorfloat
Maximum allowed residual error, where the residual error is defined by
if
, else
.
- getMaximumTimeDuration()¶
Accessor to the maximum duration.
- Returns:
- maximumTimefloat
Maximum optimization duration in seconds.
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getOffset()¶
Accessor to offset parameter.
- Returns:
- offset
Point
Constant to subtract to each variable
if empty, the constant are (min-max)/2 for interval bounded
variables and x for the others.
- offset
- getProblem()¶
Accessor to optimization problem.
- Returns:
- problem
OptimizationProblem
Optimization problem.
- problem
- getRescale()¶
Accessor to rescale parameter.
- Returns:
- rescalefloat
f scaling factor (in log10) used to trigger f value rescaling
if 0, rescale at each iteration
if a big value, never rescale
if < 0, rescale is set to 1.3.
- getResult()¶
Accessor to optimization result.
- Returns:
- result
OptimizationResult
Result class.
- result
- getScale()¶
Accessor to scale parameter.
- Returns:
- scale
Point
Scaling factors to apply to each variable
if empty, the factors are min-max for interval bounded variables
and 1+|x] for the others.
- scale
- getStepmx()¶
Accessor to stepmx parameter.
- Returns:
- stepmxfloat
Maximum step for the line search. may be increased during call
if too small, will be set to 10.0.
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- run()¶
Launch the optimization.
- setAccuracy(accuracy)¶
Accessor to accuracy parameter.
- Parameters:
- accuracyfloat
Relative precision for finite difference calculations
if <= machine_precision, set to sqrt(machine_precision).
- setCheckStatus(checkStatus)¶
Accessor to check status flag.
- Parameters:
- checkStatusbool
Whether to check the termination status. If set to False,
run()
will not throw an exception if the algorithm does not fully converge and will allow one to still find a feasible candidate.
- setEta(eta)¶
Accessor to eta parameter.
- Parameters:
- etafloat
Severity of the line search.
if < 0 or > 1, set to 0.25.
- setFmin(fmin)¶
Accessor to fmin parameter.
- Parameters:
- fminfloat
Minimum function value estimate.
- setMaxCGit(maxCGit)¶
Accessor to maxCGit parameter.
- Parameters:
- maxCGitint
Maximum number of hessian*vector evaluation per main iteration
if maxCGit = 0, the direction chosen is -gradient
if maxCGit < 0, maxCGit is set to max(1,min(50,n/2)).
- setMaximumAbsoluteError(maximumAbsoluteError)¶
Accessor to maximum allowed absolute error.
- Parameters:
- maximumAbsoluteErrorfloat
Maximum allowed absolute error, where the absolute error is defined by
where
and
are two consecutive approximations of the optimum.
- setMaximumCallsNumber(maximumCallsNumber)¶
Accessor to maximum allowed number of calls
- Parameters:
- maximumEvaluationNumberint
Maximum allowed number of direct objective function calls through the () operator. Does not take into account eventual indirect calls through finite difference gradient calls.
- setMaximumConstraintError(maximumConstraintError)¶
Accessor to maximum allowed constraint error.
- Parameters:
- maximumConstraintErrorfloat
Maximum allowed constraint error, where the constraint error is defined by
where
is the current approximation of the optimum and
is the function that gathers all the equality and inequality constraints (violated values only)
- setMaximumIterationNumber(maximumIterationNumber)¶
Accessor to maximum allowed number of iterations.
- Parameters:
- maximumIterationNumberint
Maximum allowed number of iterations.
- setMaximumRelativeError(maximumRelativeError)¶
Accessor to maximum allowed relative error.
- Parameters:
- maximumRelativeErrorfloat
Maximum allowed relative error, where the relative error is defined by
if
, else
.
- setMaximumResidualError(maximumResidualError)¶
Accessor to maximum allowed residual error.
- Parameters:
- maximumResidualErrorfloat
Maximum allowed residual error, where the residual error is defined by
if
, else
.
- setMaximumTimeDuration(maximumTime)¶
Accessor to the maximum duration.
- Parameters:
- maximumTimefloat
Maximum optimization duration in seconds.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
- setOffset(offset)¶
Accessor to offset parameter.
- Parameters:
- offsetsequence of float
Constant to subtract to each variable
if empty, the constant are (min-max)/2 for interval bounded
variables and x for the others.
- setProblem(problem)¶
Accessor to optimization problem.
- Parameters:
- problem
OptimizationProblem
Optimization problem.
- problem
- setProgressCallback(*args)¶
Set up a progress callback.
Can be used to programmatically report the progress of an optimization.
- Parameters:
- callbackcallable
Takes a float as argument as percentage of progress.
Examples
>>> import sys >>> import openturns as ot >>> rosenbrock = ot.SymbolicFunction(['x1', 'x2'], ['(1-x1)^2+100*(x2-x1^2)^2']) >>> problem = ot.OptimizationProblem(rosenbrock) >>> solver = ot.OptimizationAlgorithm(problem) >>> solver.setStartingPoint([0, 0]) >>> solver.setMaximumResidualError(1.e-3) >>> solver.setMaximumCallsNumber(10000) >>> def report_progress(progress): ... sys.stderr.write('-- progress=' + str(progress) + '%\n') >>> solver.setProgressCallback(report_progress) >>> solver.run()
- setRescale(rescale)¶
Accessor to rescale parameter.
- Parameters:
- rescalefloat
f scaling factor (in log10) used to trigger f value rescaling
if 0, rescale at each iteration
if a big value, never rescale
if < 0, rescale is set to 1.3.
- setResult(result)¶
Accessor to optimization result.
- Parameters:
- result
OptimizationResult
Result class.
- result
- setScale(scale)¶
Accessor to scale parameter.
- Parameters:
- scalesequence of float
Scaling factors to apply to each variable
if empty, the factors are min-max for interval bounded variables
and 1+|x] for the others.
- setStartingPoint(startingPoint)¶
Accessor to starting point.
- Parameters:
- startingPoint
Point
Starting point.
- startingPoint
- setStepmx(stepmx)¶
Accessor to stepmx parameter.
- Parameters:
- stepmxfloat
Maximum step for the line search. may be increased during call
if too small, will be set to 10.0.
- setStopCallback(*args)¶
Set up a stop callback.
Can be used to programmatically stop an optimization.
- Parameters:
- callbackcallable
Returns an int deciding whether to stop or continue.
Examples
>>> import openturns as ot >>> rosenbrock = ot.SymbolicFunction(['x1', 'x2'], ['(1-x1)^2+100*(x2-x1^2)^2']) >>> problem = ot.OptimizationProblem(rosenbrock) >>> solver = ot.OptimizationAlgorithm(problem) >>> solver.setStartingPoint([0, 0]) >>> solver.setMaximumResidualError(1.e-3) >>> solver.setMaximumCallsNumber(10000) >>> def ask_stop(): ... return True >>> solver.setStopCallback(ask_stop) >>> solver.run()
Examples using the class¶
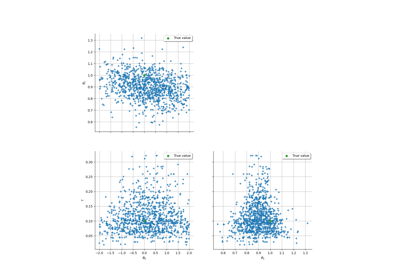
Linear Regression with interval-censored observations