FieldFunctionalChaosSobolIndices¶
- class FieldFunctionalChaosSobolIndices(*args)¶
Sobol indices from a functional decomposition.
Warning
This class is experimental and likely to be modified in future releases. To use it, import the
openturns.experimental
submodule.This class allows one to perform sensitivity analysis from field chaos decomposition. The process decomposition is done by Karhunen-Loeve and the modes interpolation is performed by functional chaos:
Let us expand the multi indices notation:
with
see
FunctionalChaosAlgorithm
for details.Sobol indices of the input field component
can be computed from the coefficients of the chaos decomposition that involve the matching Karhunen-Loeve coefficients.
For the first order Sobol indices we sum over the multi-indices
that are non-zero on the
indices corresponding to the Karhunen-Loeve decomposition of j-th input and zero on the other
indices (noted
):
For the total order Sobol indices we sum over the multi-indices
that are non-zero on the
indices corresponding to the Karhunen-Loeve decomposition of the j-th input (noted
):
This generalizes to higher order indices.
- Parameters:
- result
FieldFunctionalChaosResult
Result.
- result
See also
Examples
>>> import openturns as ot >>> import openturns.experimental as otexp >>> ot.RandomGenerator.SetSeed(0) >>> mesh = ot.RegularGrid(0.0, 0.1, 20) >>> cov = ot.KroneckerCovarianceModel(ot.MaternModel([2.0], 1.5), ot.CovarianceMatrix(4)) >>> X = ot.GaussianProcess(cov, mesh) >>> x = X.getSample(500) >>> y = [] >>> for xi in x: ... m = xi.computeMean() ... y.append([m[0] + m[1] + m[2] - m[3] + m[0] * m[1] - m[2] * m[3] - 0.1 * m[0] * m[1] * m[2]]) >>> algo = otexp.FieldToPointFunctionalChaosAlgorithm(x, y) >>> algo.setThreshold(4e-2) >>> # Temporarily lower the basis size for the sake of this example. >>> # We need to store the original size. >>> bs = ot.ResourceMap.GetAsUnsignedInteger('FunctionalChaosAlgorithm-BasisSize') >>> ot.ResourceMap.SetAsUnsignedInteger('FunctionalChaosAlgorithm-BasisSize', 100) >>> algo.run() >>> # The algorithm has been run with the lower basis size: >>> # we can now restore the original value. >>> ot.ResourceMap.SetAsUnsignedInteger('FunctionalChaosAlgorithm-BasisSize', bs) >>> result = algo.getResult() >>> sensitivity = otexp.FieldFunctionalChaosSobolIndices(result) >>> sobol_1 = sensitivity.getFirstOrderIndices() >>> sobol_t = sensitivity.getTotalOrderIndices()
Methods
draw
([marginalIndex])Draw sensitivity indices.
Accessor to the object's name.
getFirstOrderIndices
([marginalIndex])Get the first order Sobol indices.
getId
()Accessor to the object's id.
getName
()Accessor to the object's name.
Accessor to the object's shadowed id.
getSobolIndex
(*args)Get a single Sobol index.
getSobolTotalIndex
(*args)Get a single Sobol index.
getTotalOrderIndices
([marginalIndex])Get the total order Sobol indices.
Accessor to the object's visibility state.
hasName
()Test if the object is named.
Test if the object has a distinguishable name.
setName
(name)Accessor to the object's name.
setShadowedId
(id)Accessor to the object's shadowed id.
setVisibility
(visible)Accessor to the object's visibility state.
- __init__(*args)¶
- draw(marginalIndex=0)¶
Draw sensitivity indices.
- Parameters:
- marginalIndexint, default=0
Marginal index
- Returns:
- graph
Graph
A graph showing the first and total order indices per input.
- graph
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getFirstOrderIndices(marginalIndex=0)¶
Get the first order Sobol indices.
- Parameters:
- jint, default=0
Output index
- Returns:
- indices
Point
First order Sobol indices
- indices
- getId()¶
Accessor to the object’s id.
- Returns:
- idint
Internal unique identifier.
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getShadowedId()¶
Accessor to the object’s shadowed id.
- Returns:
- idint
Internal unique identifier.
- getSobolIndex(*args)¶
Get a single Sobol index.
- Parameters:
- iint or list of int
Input index
- jint, default=0
Output index
- Returns:
- sfloat
Sobol index
- getSobolTotalIndex(*args)¶
Get a single Sobol index.
- Parameters:
- iint or list of int
Input index
- jint, default=0
Output index
- Returns:
- sfloat
Sobol index
- getTotalOrderIndices(marginalIndex=0)¶
Get the total order Sobol indices.
- Parameters:
- jint, default=0
Output index
- Returns:
- indices
Point
Total order Sobol indices
- indices
- getVisibility()¶
Accessor to the object’s visibility state.
- Returns:
- visiblebool
Visibility flag.
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- hasVisibleName()¶
Test if the object has a distinguishable name.
- Returns:
- hasVisibleNamebool
True if the name is not empty and not the default one.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
- setShadowedId(id)¶
Accessor to the object’s shadowed id.
- Parameters:
- idint
Internal unique identifier.
- setVisibility(visible)¶
Accessor to the object’s visibility state.
- Parameters:
- visiblebool
Visibility flag.
Examples using the class¶
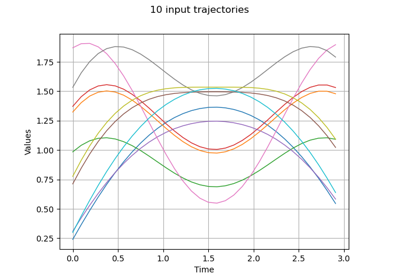
Estimate Sobol indices on a field to point function