Secant¶
(Source code
, png
)
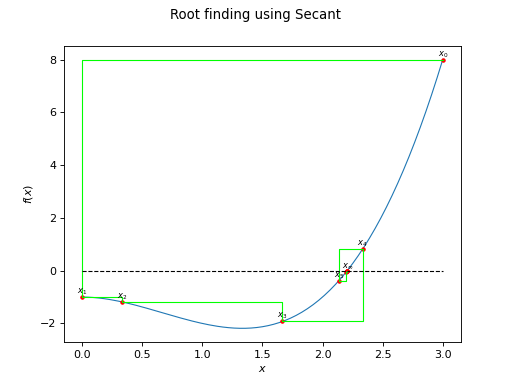
- class Secant(*args)¶
Secant algorithm solver for 1D non linear equations.
- Parameters:
- absErrorpositive float, optional
Absolute error: distance between two successive iterates at the end point. Default is
.
- relErrorpositive float, optional
Relative error: distance between the two last successive iterates with regards to the last iterate. Default is
.
- resErrorpositive float, optional
Residual error: difference between the last iterate value and the expected value. Default is
.
- maximumFunctionEvaluationint, optional
The maximum number of evaluations of the function. Default is
.
Notes
The Secant solver is based on the evaluation of a segment between the two last iterated points. Secant might fail and not converge.
Methods
Accessor to the absolute error.
Accessor to the number of function calls.
Accessor to the object's name.
Accessor to the maximum number of function calls.
getName
()Accessor to the object's name.
Accessor to the relative error.
Accessor to the residual error.
hasName
()Test if the object is named.
setAbsoluteError
(absoluteError)Accessor to the absolute error.
setMaximumCallsNumber
(maximumFunctionEvaluation)Accessor to the maximum number of function calls.
setName
(name)Accessor to the object's name.
setRelativeError
(relativeError)Accessor to the relative error.
setResidualError
(residualError)Accessor to the residual error.
solve
(*args)Solve an equation.
getMaximumFunctionEvaluation
getUsedFunctionEvaluation
setMaximumFunctionEvaluation
- __init__(*args)¶
- getAbsoluteError()¶
Accessor to the absolute error.
- Returns:
- absErrorfloat
The absolute error: distance between two successive iterates at the end point.
- getCallsNumber()¶
Accessor to the number of function calls.
- Returns:
- nEvalint
The number of function calls.
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getMaximumCallsNumber()¶
Accessor to the maximum number of function calls.
- Returns:
- maxEvalint
The maximum number of function calls.
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getRelativeError()¶
Accessor to the relative error.
- Returns:
- relErrorfloat
The relative error: distance between the two last successive iterates with regards to the last iterate.
- getResidualError()¶
Accessor to the residual error.
- Returns:
- resErrorfloat
The residual errors: difference between the last iterate value and the expected value.
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- setAbsoluteError(absoluteError)¶
Accessor to the absolute error.
- Parameters:
- absErrorfloat
The absolute error: distance between two successive iterates at the end point.
- setMaximumCallsNumber(maximumFunctionEvaluation)¶
Accessor to the maximum number of function calls.
- Parameters:
- maxEvalint
The maximum number of function calls.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
- setRelativeError(relativeError)¶
Accessor to the relative error.
- Parameters:
- relErrorfloat
The relative error: distance between the two last successive iterates with regards to the last iterate.
- setResidualError(residualError)¶
Accessor to the residual error.
- Parameters:
- resErrorfloat
The residual errors: difference between the last iterate value and the expected value.
- solve(*args)¶
Solve an equation.
- Parameters:
- function
Function
The function of the equation
to be solved in the interval
.
- valuefloat
The value to which the function must be equal.
- infPoint, supPointfloat
Lower and upper bounds of the variable
range.
- infValue, supValuefloat, optional
The values such that
, and
.
must be of opposite sign of
.
- function
- Returns:
- resultfloat
The result of the root research.