KrigingResult¶
- class KrigingResult(*args)¶
Kriging result.
- Available constructors:
KrigingResult(inputSample, outputSample, metaModel, residuals, relativeErrors, basis, trendCoefficients, covarianceModel, covarianceCoefficients)
KrigingResult(inputSample, outputSample, metaModel, residuals, relativeErrors, basis, trendCoefficients, covarianceModel, covarianceCoefficients, covarianceCholeskyFactor, covarianceHMatrix)
- Parameters:
- inputSample, outputSample2-d sequence of float
The samples
and
.
- metaModel
Function
The meta model:
, defined in (3).
- residuals
Point
The residual errors.
- relativeErrors
Point
The relative errors.
- basis
Basis
Functional basis of size
:
for each
. Its size should be equal to zero if the trend is not estimated.
- trendCoefficients
Point
The trend coefficients vectors
stored as a Point.
- covarianceModel
CovarianceModel
Covariance function of the Gaussian process.
- covarianceCoefficients2-d sequence of float
The
defined in (2).
- covarianceCholeskyFactor
TriangularMatrix
The Cholesky factor
of
.
- covarianceHMatrix
HMatrix
The hmat implementation of
.
Notes
The Kriging meta model
is defined by:
(1)¶
where
is the condition
for each
.
Equation (1) writes:
where
and
(2)¶
At the end, the meta model writes:
(3)¶
Examples
Create the model
and the samples:
>>> import openturns as ot >>> f = ot.SymbolicFunction(['x'], ['x * sin(x)']) >>> sampleX = [[1.0], [2.0], [3.0], [4.0], [5.0], [6.0]] >>> sampleY = f(sampleX)
Create the algorithm:
>>> basis = ot.Basis([ot.SymbolicFunction(['x'], ['x']), ot.SymbolicFunction(['x'], ['x^2'])]) >>> covarianceModel = ot.GeneralizedExponential([2.0], 2.0) >>> algoKriging = ot.KrigingAlgorithm(sampleX, sampleY, covarianceModel, basis) >>> algoKriging.run()
Get the result:
>>> resKriging = algoKriging.getResult()
Get the meta model:
>>> metaModel = resKriging.getMetaModel()
Methods
getBasis
()Accessor to the functional basis.
Accessor to the object's name.
getConditionalCovariance
(*args)Compute the conditional covariance of the Gaussian process on a point (or several points).
Compute the conditional covariance of the Gaussian process on a point (or several points).
Compute the conditional variance of the Gaussian process on a point (or several points).
getConditionalMean
(*args)Compute the conditional mean of the Gaussian process on a point or a sample of points.
Accessor to the covariance coefficients.
Accessor to the covariance model.
Accessor to the input sample.
Accessor to the metamodel.
getName
()Accessor to the object's name.
Accessor to the output sample.
Accessor to the relative errors.
Accessor to the residuals.
Accessor to the trend coefficients.
hasName
()Test if the object is named.
setInputSample
(sampleX)Accessor to the input sample.
setMetaModel
(metaModel)Accessor to the metamodel.
setName
(name)Accessor to the object's name.
setOutputSample
(sampleY)Accessor to the output sample.
setRelativeErrors
(relativeErrors)Accessor to the relative errors.
setResiduals
(residuals)Accessor to the residuals.
- __init__(*args)¶
- getBasis()¶
Accessor to the functional basis.
- Returns:
- basis
Basis
Functional basis of size
:
for each
.
- basis
Notes
If the trend is not estimated, the collection is empty.
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getConditionalCovariance(*args)¶
Compute the conditional covariance of the Gaussian process on a point (or several points).
- Available usages:
getConditionalCovariance(x)
getConditionalCovariance(sampleX)
- Parameters:
- xsequence of float
The point
where the conditional covariance of the output has to be evaluated.
- sampleX2-d sequence of float
The sample
where the conditional covariance of the output has to be evaluated (M can be equal to 1).
- Returns:
- condCov
CovarianceMatrix
The conditional covariance
at point
. Or the conditional covariance matrix at the sample
:
where
.
- condCov
- getConditionalMarginalCovariance(*args)¶
Compute the conditional covariance of the Gaussian process on a point (or several points).
- Available usages:
getConditionalMarginalCovariance(x)
getConditionalMarginalCovariance(sampleX)
- Parameters:
- xsequence of float
The point
where the conditional marginal covariance of the output has to be evaluated.
- sampleX2-d sequence of float
The sample
where the conditional marginal covariance of the output has to be evaluated (M can be equal to 1).
- Returns:
- condCov
CovarianceMatrix
The conditional covariance
at point
.
- condCov
CovarianceMatrixCollection
The collection of conditional covariance matrices
at each point of the sample
:
- condCov
Notes
In case input parameter is a of type
Sample
, each element of the collection corresponds to the conditional covariance with respect to the input learning set (pointwise evaluation of the getConditionalCovariance).
- getConditionalMarginalVariance(*args)¶
Compute the conditional variance of the Gaussian process on a point (or several points).
- Available usages:
getConditionalMarginalVariance(x, marginalIndex)
getConditionalMarginalVariance(sampleX, marginalIndex)
getConditionalMarginalVariance(x, marginalIndices)
getConditionalMarginalVariance(sampleX, marginalIndices)
- Parameters:
- xsequence of float
The point
where the conditional variance of the output has to be evaluated.
- sampleX2-d sequence of float
The sample
where the conditional variance of the output has to be evaluated (M can be equal to 1).
- marginalIndexint
Marginal of interest (for multiple outputs). Default value is 0
- marginalIndicessequence of int
Marginals of interest (for multiple outputs).
- Returns:
- varfloat
Variance of interest. float if one point (x) and one marginal of interest (x, marginalIndex)
- varPointsequence of float
The marginal variances
Notes
In case of fourth usage, the sequence of float is given as the concatenation of marginal variances for each point in sampleX.
- getConditionalMean(*args)¶
Compute the conditional mean of the Gaussian process on a point or a sample of points.
- Available usages:
getConditionalMean(x)
getConditionalMean(sampleX)
- Parameters:
- xsequence of float
The point
where the conditional mean of the output has to be evaluated.
- sampleX2-d sequence of float
The sample
where the conditional mean of the output has to be evaluated (M can be equal to 1).
- Returns:
- condMean
Point
The conditional mean
at point
. Or the conditional mean matrix at the sample
:
- condMean
- getCovarianceCoefficients()¶
Accessor to the covariance coefficients.
- getCovarianceModel()¶
Accessor to the covariance model.
- Returns:
- covModel
CovarianceModel
The covariance model of the Gaussian process W with its optimized parameters.
- covModel
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getRelativeErrors()¶
Accessor to the relative errors.
- Returns:
- relativeErrors
Point
The relative errors defined as follows for each output of the model:
with
the vector of the
model’s values
and
the metamodel’s values.
- relativeErrors
- getResiduals()¶
Accessor to the residuals.
- Returns:
- residuals
Point
The residual values defined as follows for each output of the model:
with
the
model’s values and
the metamodel’s values.
- residuals
- getTrendCoefficients()¶
Accessor to the trend coefficients.
- Returns:
- trendCoef
Point
The trend coefficients vectors
stored as a Point.
- trendCoef
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- setInputSample(sampleX)¶
Accessor to the input sample.
- Parameters:
- inputSample
Sample
The input sample.
- inputSample
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
- setOutputSample(sampleY)¶
Accessor to the output sample.
- Parameters:
- outputSample
Sample
The output sample.
- outputSample
- setRelativeErrors(relativeErrors)¶
Accessor to the relative errors.
- Parameters:
- relativeErrorssequence of float
The relative errors defined as follows for each output of the model:
with
the vector of the
model’s values
and
the metamodel’s values.
- setResiduals(residuals)¶
Accessor to the residuals.
- Parameters:
- residualssequence of float
The residual values defined as follows for each output of the model:
with
the
model’s values and
the metamodel’s values.
Examples using the class¶
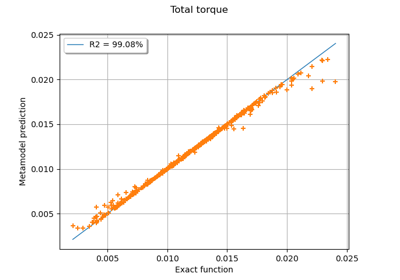
Example of multi output Kriging on the fire satellite model
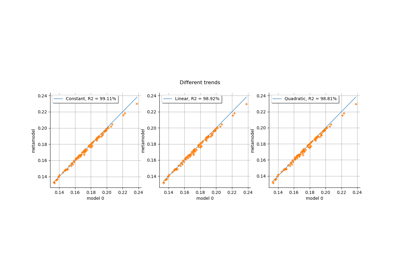
Kriging: choose a polynomial trend on the beam model
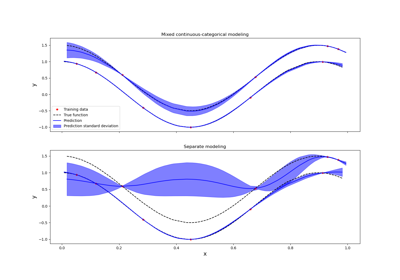
Kriging: metamodel with continuous and categorical variables