QuadraticTaylor¶
- class QuadraticTaylor(*args)¶
Second-order Taylor expansion.
- Parameters:
- centersequence of float
Point
.
- function
Function
Function
to be approximated at the point
.
See also
Notes
The response surface is the second-order Taylor expansion of the function
at the point
. Refer to Taylor Expansion for details.
Examples
>>> import openturns as ot >>> formulas = ['x1 * sin(x2)', 'cos(x1 + x2)', '(x2 + 1) * exp(x1 - 2 * x2)'] >>> myFunc = ot.SymbolicFunction(['x1', 'x2'], formulas) >>> myTaylor = ot.QuadraticTaylor([1, 2], myFunc) >>> myTaylor.run() >>> responseSurface = myTaylor.getMetaModel() >>> print(responseSurface([1.2,1.9])) [1.13655,-0.999155,0.214084]
Methods
Get the center.
Accessor to the object's name.
Get the constant vector of the approximation.
Get the function.
Get the gradient of the function at
.
Get the polynomial approximation of the function.
getName
()Accessor to the object's name.
Get the hessian of the function at
.
hasName
()Test if the object is named.
run
()Perform the second-order Taylor expansion around
.
setName
(name)Accessor to the object's name.
- __init__(*args)¶
- getCenter()¶
Get the center.
- Returns:
- center
Point
Point
where the Taylor expansion of the function is performed.
- center
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getMetaModel()¶
Get the polynomial approximation of the function.
- Returns:
- approximation
Function
The second-order Taylor expansion of
at
.
- approximation
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getQuadratic()¶
Get the hessian of the function at
.
- Returns:
- tensor
SymmetricTensor
The tensor
.
- tensor
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- run()¶
Perform the second-order Taylor expansion around
.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
Examples using the class¶
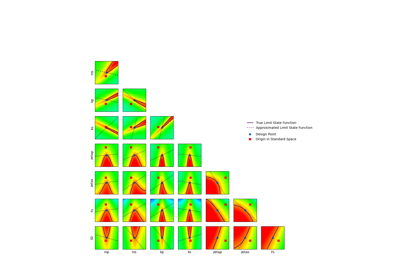
Using the FORM - SORM algorithms on a nonlinear function