Create a general linear model metamodelΒΆ
In this example we are going to create a global approximation of a model response using a general linear model. We show how to use the GeneralLinearModelAlgorithm
class, which estimates the parameters of the model.
[1]:
from __future__ import print_function
import openturns as ot
We create a model and a sample from this model.
[2]:
ot.RandomGenerator.SetSeed(0)
dimension = 2
input_names = ['x1', 'x2']
formulas = ['cos(x1 + x2)']
model = ot.SymbolicFunction(input_names, formulas)
distribution = ot.Normal(dimension)
x = distribution.getSample(100)
y = model(x)
We create a GeneralLinearModelAlgorithm
based on a linear basis. The run
method estimats the coefficients of the trend and the hyperparameters of the covariance model.
[3]:
basis = ot.LinearBasisFactory(dimension).build()
covarianceModel = ot.SquaredExponential([1]*dimension, [1.0])
algo = ot.GeneralLinearModelAlgorithm(x, y, covarianceModel, basis)
algo.run()
result = algo.getResult()
We see that the trend coefficients have been estimated.
[4]:
result.getTrendCoefficients()
[4]:
[class=Point name=Unnamed dimension=3 values=[-0.111515,-0.0142378,-0.0129734]]
The parameters of the covariance models also have been estimated.
[5]:
result.getCovarianceModel()
[5]:
SquaredExponential(scale=[0.810148,1.52486], amplitude=[0.404099])
The getMetaModel
method returns the metamodel where the parameters have been estimated.
[6]:
responseSurface = result.getMetaModel()
Plot the output of our model depending on x2
with x1=0.5
.
[7]:
xmin = -5.
xmax = 5.
x1value = 0.5
parametricModelGraph = ot.ParametricFunction(model, [0], [x1value]).draw(xmin,xmax)
parametricModelGraph.setColors(["red"])
graphMetamodel = ot.ParametricFunction(responseSurface, [0], [x1value]).draw(xmin,xmax)
graphMetamodel.setColors(["blue"])
parametricModelGraph.add(graphMetamodel)
parametricModelGraph.setLegends(["Model","Meta-Model"])
parametricModelGraph.setLegendPosition("topright")
parametricModelGraph
[7]:
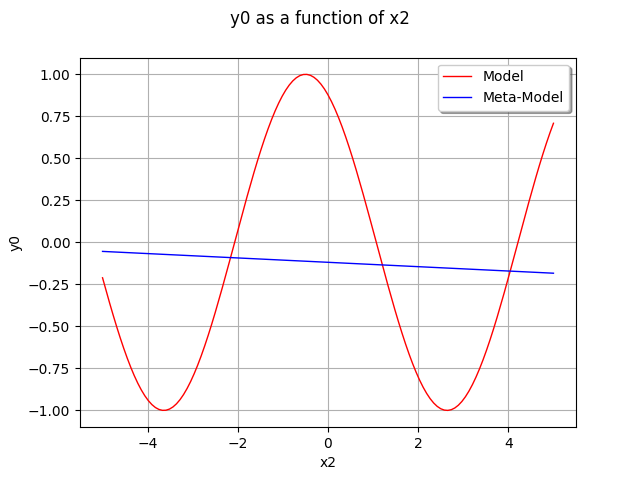
We see that the metamodel is equal to the trend because it takes into account the fact that the mean of the gaussian process is zero.
This GeneralLinearModelAlgorithm
class is the main building block of the KrigingAlgorithm
. This is why most basic use cases are based on the KrigingAlgorithm
instead of the GeneralLinearModelAlgorithm
, because this allows to condition the gaussian process.