Create a random walk processΒΆ
This example details first how to create and manipulate a random walk.
A random walk is a process where
discretized on the time grid
such that:
where and
is a white noise of dimension
.
The library proposes to model it through the object RandomWalk defined thanks to the origin, the distribution of the white noise and the time grid.
[1]:
from __future__ import print_function
import openturns as ot
import math as m
[2]:
# Define the origin
origin = [0.0]
[3]:
# Define an 1-d mesh
tgrid = ot.RegularGrid(0.0, 1.0, 500)
[4]:
# 1-d random walk and discrete distribution
dist = ot.UserDefined([[-1], [10]],[0.9, 0.1] )
process = ot.RandomWalk(origin, dist, tgrid)
sample = process.getSample(5)
graph = sample.drawMarginal(0)
graph.setTitle('1D Random Walk with discrete steps')
graph
[4]:
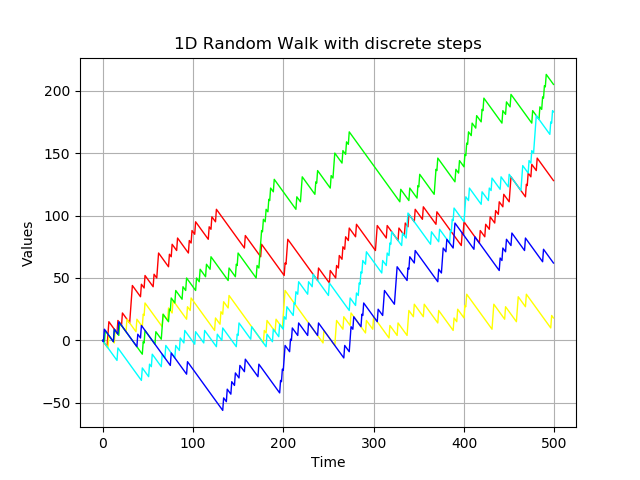
[5]:
# 1-d random walk and continuous distribution
dist = ot.Normal(0.0, 1.0)
process = ot.RandomWalk(origin, dist, tgrid)
sample = process.getSample(5)
graph = sample.drawMarginal(0)
graph.setTitle('1D Random Walk with continuous steps')
graph
[5]:
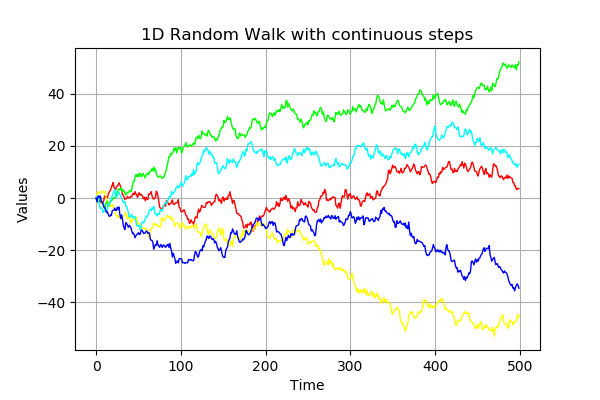
[6]:
# Define the origin
origin = [0.0]*2
[7]:
# color palette
pal = ['red', 'cyan', 'blue', 'yellow', 'green']
[8]:
# 2-d random walk and discrete distribution
dist = ot.UserDefined([[-1., -2.], [1., 3.]], [0.5, 0.5])
process = ot.RandomWalk(origin, dist, tgrid)
sample = process.getSample(5)
graph = ot.Graph('2D Random Walk with discrete steps', 'X1', 'X2', True)
for i in range(5) :
graph.add(ot.Curve(sample[i], pal[i % len(pal)], 'solid'))
graph
[8]:
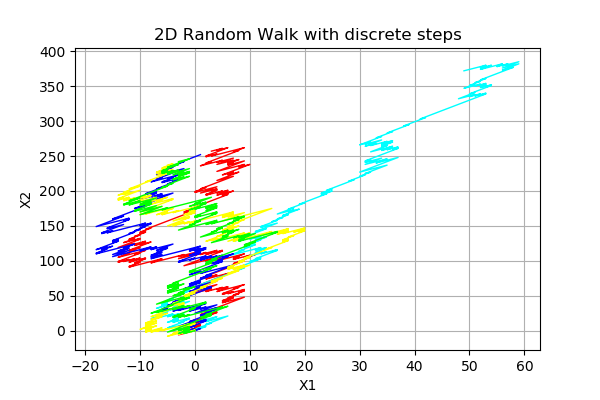
[9]:
# 2-d random walk and continuous distribution
dist = ot.Normal(2)
process = ot.RandomWalk(origin, dist, tgrid)
sample = process.getSample(5)
graph = ot.Graph('2D Random Walk with continuous steps', 'X1', 'X2', True)
for i in range(5) :
graph.add(ot.Curve(sample[i], pal[i % len(pal)], 'solid'))
graph
[9]:
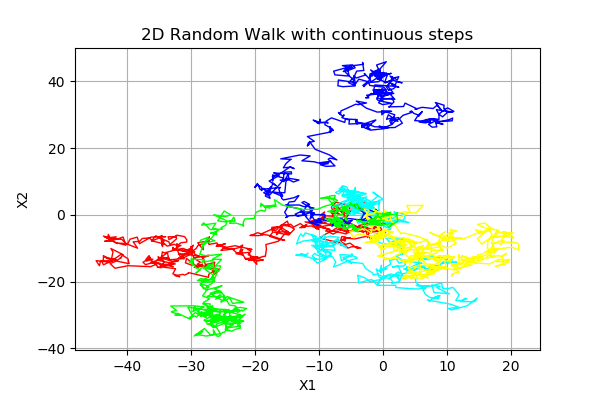