CompositeProcess¶
(Source code, png, hires.png, pdf)
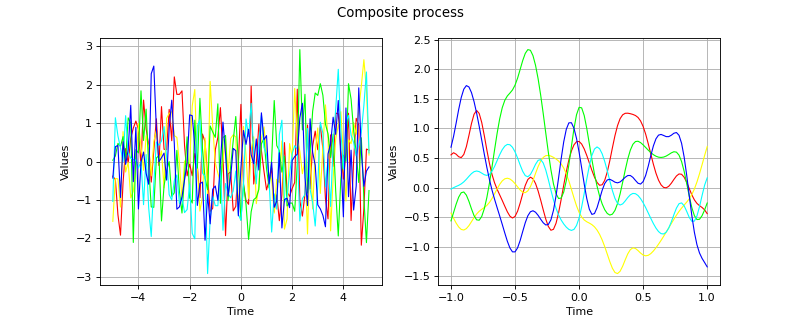
-
class
CompositeProcess
(*args)¶ Process obtained by transformation.
- Parameters
- fdyn
FieldFunction
A field function.
- inputProc
Process
The input process.
- fdyn
Notes
A composite process is the image of process
by the field function
:
where
and
, defined by:
with
and
.
The process
is defined on the domain
associated to the mesh
.
Examples
Create the process X:
>>> import openturns as ot >>> amplitude = [1.0, 1.0] >>> scale = [0.2, 0.3] >>> myCovModel = ot.ExponentialModel(scale, amplitude) >>> myMesh = ot.IntervalMesher([100]*2).build(ot.Interval([0.0]*2, [1.0]*2)) >>> myXProcess = ot.GaussianProcess(myCovModel, myMesh)
Create a spatial field function
associated to
where
:
>>> g = ot.SymbolicFunction(['x1', 'x2'], ['x1^2', 'x1+x2']) >>> nSpat = 2 >>> gdyn = ot.ValueFunction(g, myMesh)
Create the Y process
:
>>> myYProcess = ot.CompositeProcess(gdyn, myXProcess)
Add the trend
where
:
>>> f = ot.SymbolicFunction(['x1', 'x2'], ['1+2*x1', '1+3*x2^2']) >>> fTrend = ot.TrendTransform(f, myMesh)
Create the process
:
>>> myYProcess2 = ot.CompositeProcess(fTrend, myXProcess)
Apply the Box Cox transformation
where
:
>>> h = ot.BoxCoxTransform([3.0, 0.0]) >>> hBoxCox = ot.ValueFunction(h, myMesh)
Create the Y process
:
>>> myYProcess3 = ot.CompositeProcess(hBoxCox, myXProcess)
Methods
getAntecedent
(self)Get the antecedent process.
getClassName
(self)Accessor to the object’s name.
getContinuousRealization
(self)Get a continuous realization.
getCovarianceModel
(self)Accessor to the covariance model.
getDescription
(self)Get the description of the process.
getFunction
(self)Get the field function.
getFuture
(self, \*args)Prediction of the
future iterations of the process.
getId
(self)Accessor to the object’s id.
getInputDimension
(self)Get the dimension of the domain
.
getMarginal
(self, indices)Get the
marginal of the random process.
getMesh
(self)Get the mesh.
getName
(self)Accessor to the object’s name.
getOutputDimension
(self)Get the dimension of the domain
.
getRealization
(self)Get a realization of the process.
getSample
(self, size)Get
realizations of the process.
getShadowedId
(self)Accessor to the object’s shadowed id.
getTimeGrid
(self)Get the time grid of observation of the process.
getTrend
(self)Accessor to the trend.
getVisibility
(self)Accessor to the object’s visibility state.
hasName
(self)Test if the object is named.
hasVisibleName
(self)Test if the object has a distinguishable name.
isComposite
(self)Test whether the process is composite or not.
isNormal
(self)Test whether the process is normal or not.
isStationary
(self)Test whether the process is stationary or not.
setDescription
(self, description)Set the description of the process.
setMesh
(self, mesh)Set the mesh.
setName
(self, name)Accessor to the object’s name.
setShadowedId
(self, id)Accessor to the object’s shadowed id.
setTimeGrid
(self, timeGrid)Set the time grid of observation of the process.
setVisibility
(self, visible)Accessor to the object’s visibility state.
-
__init__
(self, \*args)¶ Initialize self. See help(type(self)) for accurate signature.
-
getClassName
(self)¶ Accessor to the object’s name.
- Returns
- class_namestr
The object class name (object.__class__.__name__).
-
getContinuousRealization
(self)¶ Get a continuous realization.
- Returns
- realization
Function
According to the process, the continuous realizations are built:
either using a dedicated functional model if it exists: e.g. a functional basis process.
or using an interpolation from a discrete realization of the process on
: in dimension
, a linear interpolation and in dimension
, a piecewise constant function (the value at a given position is equal to the value at the nearest vertex of the mesh of the process).
- realization
-
getCovarianceModel
(self)¶ Accessor to the covariance model.
- Returns
- cov_model
CovarianceModel
Covariance model, if any.
- cov_model
-
getDescription
(self)¶ Get the description of the process.
- Returns
- description
Description
Description of the process.
- description
-
getFunction
(self)¶ Get the field function.
- Returns
- fdyn
FieldFunction
The field function
.
- fdyn
-
getFuture
(self, \*args)¶ Prediction of the
future iterations of the process.
- Parameters
- stepNumberint,
Number of future steps.
- sizeint,
, optional
Number of futures needed. Default is 1.
- stepNumberint,
- Returns
- prediction
ProcessSample
orTimeSeries
future iterations of the process. If
, prediction is a
TimeSeries
. Otherwise, it is aProcessSample
.
- prediction
-
getId
(self)¶ Accessor to the object’s id.
- Returns
- idint
Internal unique identifier.
-
getInputDimension
(self)¶ Get the dimension of the domain
.
- Returns
- nint
Dimension of the domain
:
.
-
getMarginal
(self, indices)¶ Get the
marginal of the random process.
- Parameters
- kint or list of ints
Index of the marginal(s) needed.
- kint or list of ints
- Returns
- marginals
Process
Process defined with marginal(s) of the random process.
- marginals
-
getName
(self)¶ Accessor to the object’s name.
- Returns
- namestr
The name of the object.
-
getOutputDimension
(self)¶ Get the dimension of the domain
.
- Returns
- dint
Dimension of the domain
.
-
getRealization
(self)¶ Get a realization of the process.
- Returns
- realization
Field
Contains a mesh over which the process is discretized and the values of the process at the vertices of the mesh.
- realization
-
getSample
(self, size)¶ Get
realizations of the process.
- Parameters
- nint,
Number of realizations of the process needed.
- nint,
- Returns
- processSample
ProcessSample
realizations of the random process. A process sample is a collection of fields which share the same mesh
.
- processSample
-
getShadowedId
(self)¶ Accessor to the object’s shadowed id.
- Returns
- idint
Internal unique identifier.
-
getTimeGrid
(self)¶ Get the time grid of observation of the process.
- Returns
- timeGrid
RegularGrid
Time grid of a process when the mesh associated to the process can be interpreted as a
RegularGrid
. We check if the vertices of the mesh are scalar and are regularly spaced inbut we don’t check if the connectivity of the mesh is conform to the one of a regular grid (without any hole and composed of ordered instants).
- timeGrid
-
getTrend
(self)¶ Accessor to the trend.
- Returns
- trend
TrendTransform
Trend, if any.
- trend
-
getVisibility
(self)¶ Accessor to the object’s visibility state.
- Returns
- visiblebool
Visibility flag.
-
hasName
(self)¶ Test if the object is named.
- Returns
- hasNamebool
True if the name is not empty.
-
hasVisibleName
(self)¶ Test if the object has a distinguishable name.
- Returns
- hasVisibleNamebool
True if the name is not empty and not the default one.
-
isComposite
(self)¶ Test whether the process is composite or not.
- Returns
- isCompositebool
True if the process is composite (built upon a function and a process).
-
isNormal
(self)¶ Test whether the process is normal or not.
- Returns
- isNormalbool
True if the process is normal.
Notes
A stochastic process is normal if all its finite dimensional joint distributions are normal, which means that for all
and
, with
, there is
and
such that:
where
,
and
and
is the symmetric matrix:
A Gaussian process is entirely defined by its mean function
and its covariance function
(or correlation function
).
-
isStationary
(self)¶ Test whether the process is stationary or not.
- Returns
- isStationarybool
True if the process is stationary.
Notes
A process
is stationary if its distribution is invariant by translation:
,
,
, we have:
-
setDescription
(self, description)¶ Set the description of the process.
- Parameters
- descriptionsequence of str
Description of the process.
-
setName
(self, name)¶ Accessor to the object’s name.
- Parameters
- namestr
The name of the object.
-
setShadowedId
(self, id)¶ Accessor to the object’s shadowed id.
- Parameters
- idint
Internal unique identifier.
-
setTimeGrid
(self, timeGrid)¶ Set the time grid of observation of the process.
- Returns
- timeGrid
RegularGrid
Time grid of observation of the process when the mesh associated to the process can be interpreted as a
RegularGrid
. We check if the vertices of the mesh are scalar and are regularly spaced inbut we don’t check if the connectivity of the mesh is conform to the one of a regular grid (without any hole and composed of ordered instants).
- timeGrid
-
setVisibility
(self, visible)¶ Accessor to the object’s visibility state.
- Parameters
- visiblebool
Visibility flag.