HaselgroveSequence¶
(Source code, png, hires.png, pdf)
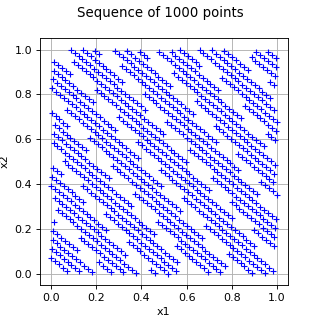
-
class
HaselgroveSequence
(*args)¶ Haselgrove sequence.
- Available constructors:
HaselgroveSequence(dimension=1)
HaselgroveSequence(base)
- Parameters
- dimensionpositive int
Dimension of the points.
- basesequence of positive float
Sequence of positive real values linearly independent over the integer ring, i.e. no linear combination with integer coefficients of these values can be zero excepted if all the coefficients are zero. The dimension of the sequence is given by the dimension of the base.
Examples
>>> import openturns as ot >>> sequence = ot.HaselgroveSequence(2) >>> print(sequence.generate(5)) 0 : [ 0.414214 0.732051 ] 1 : [ 0.828427 0.464102 ] 2 : [ 0.242641 0.196152 ] 3 : [ 0.656854 0.928203 ] 4 : [ 0.0710678 0.660254 ]
Methods
ComputeStarDiscrepancy
(sample)Compute the star discrepancy of a sample uniformly distributed over [0, 1).
generate
(self, \*args)Generate a sample of pseudo-random vectors of numbers uniformly distributed over [0, 1).
getClassName
(self)Accessor to the object’s name.
getDimension
(self)Accessor to the dimension of the points of the low discrepancy sequence.
getId
(self)Accessor to the object’s id.
getName
(self)Accessor to the object’s name.
getShadowedId
(self)Accessor to the object’s shadowed id.
getVisibility
(self)Accessor to the object’s visibility state.
hasName
(self)Test if the object is named.
hasVisibleName
(self)Test if the object has a distinguishable name.
initialize
(self, dimension)Initialize the sequence.
setName
(self, name)Accessor to the object’s name.
setShadowedId
(self, id)Accessor to the object’s shadowed id.
setVisibility
(self, visible)Accessor to the object’s visibility state.
-
__init__
(self, \*args)¶ Initialize self. See help(type(self)) for accurate signature.
-
static
ComputeStarDiscrepancy
(sample)¶ Compute the star discrepancy of a sample uniformly distributed over [0, 1).
- Parameters
- sample2-d sequence of float
- Returns
- starDiscrepancyfloat
Star discrepancy of a sample uniformly distributed over [0, 1).
Examples
>>> import openturns as ot >>> # Create a sequence of 3 points of 2 dimensions >>> sequence = ot.LowDiscrepancySequence(ot.SobolSequence(2)) >>> sample = sequence.generate(16) >>> print(sequence.computeStarDiscrepancy(sample)) 0.12890625 >>> sample = sequence.generate(64) >>> print(sequence.computeStarDiscrepancy(sample)) 0.0537109375
-
generate
(self, \*args)¶ Generate a sample of pseudo-random vectors of numbers uniformly distributed over [0, 1).
- Parameters
- sizeint
Number of points to be generated. Default is 1.
- Returns
- sample
Sample
Sample of pseudo-random vectors of numbers uniformly distributed over [0, 1).
- sample
Examples
>>> import openturns as ot >>> # Create a sequence of 3 points of 2 dimensions >>> sequence = ot.LowDiscrepancySequence(ot.SobolSequence(2)) >>> print(sequence.generate(3)) 0 : [ 0.5 0.5 ] 1 : [ 0.75 0.25 ] 2 : [ 0.25 0.75 ]
-
getClassName
(self)¶ Accessor to the object’s name.
- Returns
- class_namestr
The object class name (object.__class__.__name__).
-
getDimension
(self)¶ Accessor to the dimension of the points of the low discrepancy sequence.
- Returns
- dimensionint
Dimension of the points of the low discrepancy sequence.
-
getId
(self)¶ Accessor to the object’s id.
- Returns
- idint
Internal unique identifier.
-
getName
(self)¶ Accessor to the object’s name.
- Returns
- namestr
The name of the object.
-
getShadowedId
(self)¶ Accessor to the object’s shadowed id.
- Returns
- idint
Internal unique identifier.
-
getVisibility
(self)¶ Accessor to the object’s visibility state.
- Returns
- visiblebool
Visibility flag.
-
hasName
(self)¶ Test if the object is named.
- Returns
- hasNamebool
True if the name is not empty.
-
hasVisibleName
(self)¶ Test if the object has a distinguishable name.
- Returns
- hasVisibleNamebool
True if the name is not empty and not the default one.
-
initialize
(self, dimension)¶ Initialize the sequence.
- Parameters
- dimensionint
Dimension of the points of the low discrepancy sequence.
Examples
>>> import openturns as ot >>> # Create a sequence of 3 points of 2 dimensions >>> sequence = ot.LowDiscrepancySequence(ot.SobolSequence(2)) >>> print(sequence.generate(3)) 0 : [ 0.5 0.5 ] 1 : [ 0.75 0.25 ] 2 : [ 0.25 0.75 ] >>> print(sequence.generate(3)) 0 : [ 0.375 0.375 ] 1 : [ 0.875 0.875 ] 2 : [ 0.625 0.125 ] >>> sequence.initialize(2) >>> print(sequence.generate(3)) 0 : [ 0.5 0.5 ] 1 : [ 0.75 0.25 ] 2 : [ 0.25 0.75 ]
-
setName
(self, name)¶ Accessor to the object’s name.
- Parameters
- namestr
The name of the object.
-
setShadowedId
(self, id)¶ Accessor to the object’s shadowed id.
- Parameters
- idint
Internal unique identifier.
-
setVisibility
(self, visible)¶ Accessor to the object’s visibility state.
- Parameters
- visiblebool
Visibility flag.