Field¶
(Source code, png, hires.png, pdf)
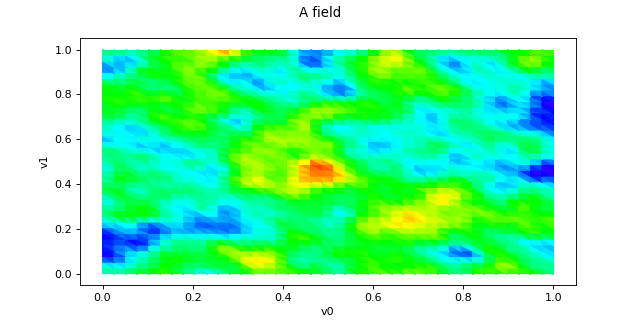
-
class
Field
(*args)¶ Base class for Fields.
- Available constructors:
Field(mesh, dim)
Field(mesh, values)
- Parameters
- mesh
Mesh
Each vertice of the mesh is in
a domain of
.
- dimint
Dimension
of the values.
- values2-d sequence of float of dimension
The values associated to the vertices of the mesh. The size of values is equal to the number of vertices in the associated mesh. So we must have the equality between values.getSize() and mesh.getVerticesNumber().
- mesh
Notes
A field
is an association between vertices and values:
where the
are the vertices of a mesh of the domain
and
are the associated values.
Mathematically speaking,
is an element
where
is the number of vertices of the mesh
of the domain
.
Examples
Create a field:
>>> import openturns as ot >>> myVertices = [[0.0, 0.0], [1.0, 0.0], [1.0, 1.0], [1.5, 1.0], [2.0, 1.5], [0.5, 1.5]] >>> mySimplicies = [[0,1,2], [1,2,3], [2,3,4], [2,4,5], [0,2,5]] >>> myMesh = ot.Mesh(myVertices, mySimplicies) >>> myValues = [[2.0],[2.5],[4.0], [4.5], [6.0], [7.0]] >>> myField = ot.Field(myMesh, myValues)
Draw the field:
>>> myGraph = myField.draw()
Methods
asDeformedMesh
(*args)Get the mesh deformed according to the values of the field.
draw
()Draw the first marginal of the field if the input dimension is less than 2.
drawMarginal
([index, interpolate])Draw one marginal field if the input dimension is less than 2.
exportToVTKFile
(fileName)Create the VTK format file of the field.
Accessor to the object’s name.
Get the description of the field values.
getId
()Accessor to the object’s id.
Accessor to the underlying implementation.
Get the dimension of the domain
.
Get the input weighted mean of the values of the field.
getMarginal
(*args)Marginal accessor.
getMesh
()Get the mesh on which the field is defined.
getName
()Accessor to the object’s name.
Get the dimension
of the values.
getSize
()Get the number of values inside the field.
Get the mesh as a time grid if it is 1D and regular.
getValueAtIndex
(index)Get the value of the field at the vertex of the given index.
Get the values of the field.
setDescription
(description)Set the description of the vertices and values of the field.
setName
(name)Accessor to the object’s name.
setValueAtIndex
(index, val)Assign the value of the field to the vertex at the given index.
setValues
(values)Assign values to a field.
-
__init__
(*args)¶ Initialize self. See help(type(self)) for accurate signature.
-
asDeformedMesh
(*args)¶ Get the mesh deformed according to the values of the field.
- Parameters
- verticesPaddingsequence of int
The positions at which the coordinates of vertices are set to zero when extending the vertices dimension. By default the sequence is empty.
- valuesPaddingsequence of int
The positions at which the components of values are set to zero when extending the values dimension. By default the sequence is empty.
- Returns
- deformedMesh
Mesh
The initial mesh is deformed as follows: each vertex of the mesh is translated by the value of the field at this vertex. Only works when the input dimension
: is equal to the dimension of the field
after extension.
- deformedMesh
-
draw
()¶ Draw the first marginal of the field if the input dimension is less than 2.
- Returns
- graph
Graph
Calls drawMarginal(0, False).
- graph
See also
-
drawMarginal
(index=0, interpolate=True)¶ Draw one marginal field if the input dimension is less than 2.
- Parameters
- indexint
The selected marginal.
- interpolatebool
Indicates whether the values at the vertices are linearly interpolated.
- Returns
- graph
Graph
If the dimension of the mesh is
and interpolate=True: it draws the graph of the piecewise linear function based on the selected marginal values of the field and the vertices coordinates (in
).
If the dimension of the mesh is
and interpolate=False: it draws the cloud of points which coordinates are (vertex, value of the marginal index).
If the dimension of the mesh is
and interpolate=True: it draws several iso-values curves of the selected marginal, based on a piecewise linear interpolation within the simplices (triangles) of the mesh. You get an empty graph if the vertices are not connected through simplicies.
If the dimension of the mesh is
and interpolate=False: if the vertices are connected through simplicies, each simplex is drawn with a color defined by the mean of the values of the vertices of the simplex. In the other case, it draws each vertex colored by its value.
- graph
-
exportToVTKFile
(fileName)¶ Create the VTK format file of the field.
- Parameters
- myVTKFilestr
Name of the output file. No extension is append to the filename.
Notes
Creates the VTK format file that contains the mesh and the associated values that can be visualised with the open source software Paraview .
-
getClassName
()¶ Accessor to the object’s name.
- Returns
- class_namestr
The object class name (object.__class__.__name__).
-
getDescription
()¶ Get the description of the field values.
- Returns
- description
Description
Description of the vertices and values of the field, size
.
- description
-
getId
()¶ Accessor to the object’s id.
- Returns
- idint
Internal unique identifier.
-
getImplementation
()¶ Accessor to the underlying implementation.
- Returns
- implImplementation
The implementation class.
-
getInputDimension
()¶ Get the dimension of the domain
.
- Returns
- nint
Dimension of the domain
:
.
-
getInputMean
()¶ Get the input weighted mean of the values of the field.
- Returns
- inputMean
Point
Weighted mean of the values of the field, weighted by the volume of each simplex.
- inputMean
Notes
The input mean of the field is defined by:
where
is the simplex of index
of the mesh,
its volume and
the values of the field associated to the vertices of
, and
.
-
getMarginal
(*args)¶ Marginal accessor.
- Parameters
- iint or sequence of int
Index of the marginal.
- Returns
- value
Field
. Marginal field.
- value
-
getMesh
()¶ Get the mesh on which the field is defined.
- Returns
- mesh
Mesh
Mesh over which the domain
is discretized.
- mesh
-
getName
()¶ Accessor to the object’s name.
- Returns
- namestr
The name of the object.
-
getOutputDimension
()¶ Get the dimension
of the values.
- Returns
- dint
Dimension of the field values:
.
-
getSize
()¶ Get the number of values inside the field.
- Returns
- sizeint
Number
of vertices in the mesh.
-
getTimeGrid
()¶ Get the mesh as a time grid if it is 1D and regular.
- Returns
- timeGrid
RegularGrid
Mesh of the field when it can be interpreted as a
RegularGrid
. We check if the vertices of the mesh are scalar and are regularly spaced inbut we don’t check if the connectivity of the mesh is conform to the one of a regular grid (without any hole and composed of ordered instants).
- timeGrid
-
getValueAtIndex
(index)¶ Get the value of the field at the vertex of the given index.
- Parameters
- indexint
Vertex of the mesh of index index.
- Returns
- value
Point
The value of the field associated to the selected vertex, in
.
- value
-
getValues
()¶ Get the values of the field.
- Returns
- values
Sample
Values associated to the mesh. The size of the sample is the number of vertices of the mesh and the dimension is the dimension of the values (
). Identical to
getSample()
.
- values
-
setDescription
(description)¶ Set the description of the vertices and values of the field.
- Parameters
- myDescription
Description
Description of the field values. Must be of size
and give the description of the vertices and the values.
- myDescription
-
setName
(name)¶ Accessor to the object’s name.
- Parameters
- namestr
The name of the object.
-
setValueAtIndex
(index, val)¶ Assign the value of the field to the vertex at the given index.
- Parameters
- indexint
Index that characterizes one vertex of the mesh.
- value
Point
in.
New value assigned to the selected vertex.
-
setValues
(values)¶ Assign values to a field.
- Parameters
- values2-d sequence of float
Values assigned to the mesh. The size of the values is the number of vertices of the mesh and the dimension is
.