CalibrationResult¶
(Source code
, png
)
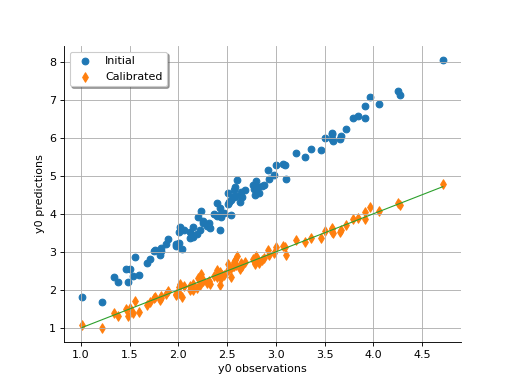
- class CalibrationResult(*args)¶
Calibration result.
Returned by calibration algorithms, see
CalibrationAlgorithm
.- Parameters:
- parameterPrior
Distribution
The prior distribution of the parameter.
- parameterPosterior
Distribution
The posterior distribution of the parameter.
- parameterMapsequence of float
The maximum a posteriori estimate of the parameter.
- observationsError
Distribution
The distribution of the observations error.
- inputObservations
Sample
The sample of input observations.
- outputObservations
Sample
The sample of output observations.
- residualFunction
Function
The residual function.
- parameterPrior
Notes
The residual function returns model(inputObservations) - outputObservations.
Examples
>>> import openturns as ot
# assume we obtained a result from CalibrationAlgorithm
>>> result = ot.CalibrationResult() >>> pmap = result.getParameterMAP() >>> prior = result.getParameterPrior() >>> posterior = result.getParameterPosterior() >>> graph1 = result.drawParameterDistributions() >>> graph2 = result.drawResiduals() >>> graph3 = result.drawObservationsVsInputs() >>> graph4 = result.drawObservationsVsPredictions()
Methods
Draw observations/inputs.
Draw observations/predictions.
Draw parameter prior/posterior.
Draw residuals.
Draw residuals normal plot.
Accessor to the object's name.
Accessor to the input observations.
getName
()Accessor to the object's name.
Accessor to the observations error distribution.
Accessor to the output observations.
Accessor to the output observations.
Accessor to the output observations.
Accessor to the maximum a posteriori parameter estimate.
Accessor to the parameter posterior distribution.
Accessor to the parameter prior distribution.
Accessor to the residual function.
hasName
()Test if the object is named.
setInputObservations
(inputObservations)Accessor to the input observations.
setName
(name)Accessor to the object's name.
setObservationsError
(observationsError)Accessor to the observations error distribution.
Accessor to the output at prior/posterior mean.
setOutputObservations
(outputObservations)Accessor to the output observations.
setParameterMAP
(parameterMAP)Accessor to the maximum a posteriori parameter estimate.
setParameterPosterior
(parameterPosterior)Accessor to the parameter posterior distribution.
setParameterPrior
(parameterPrior)Accessor to the parameter prior distribution.
setResidualFunction
(residualFunction)Accessor to the residual function.
- __init__(*args)¶
- drawObservationsVsInputs()¶
Draw observations/inputs.
Plot the observed output of the model depending on the observed input before and after calibration.
- Returns:
- grid
GridLayout
Graph array.
- grid
- drawObservationsVsPredictions()¶
Draw observations/predictions.
Plots the output of the model depending on the output observations before and after calibration.
- Returns:
- grid
GridLayout
Graph array.
- grid
- drawParameterDistributions()¶
Draw parameter prior/posterior.
Plots the prior and posterior distribution of the calibrated parameter theta.
- Returns:
- grid
GridLayout
Graph array.
- grid
- drawResiduals()¶
Draw residuals.
Plot the distribution of the sample residuals before and after calibration using kernel smoothing and the distribution of the observation errors.
- Returns:
- grid
GridLayout
Graph array.
- grid
- drawResidualsNormalPlot()¶
Draw residuals normal plot.
Plots the quantile-quantile graphs of the empirical residual after calibration vs the Gaussian distribution.
- Returns:
- grid
GridLayout
Graph array.
- grid
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getInputObservations()¶
Accessor to the input observations.
- Returns:
- inputObservations
Sample
The sample of input observations.
- inputObservations
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getObservationsError()¶
Accessor to the observations error distribution.
- Returns:
- observationsError
Distribution
The observations error distribution.
- observationsError
- getOutputAtPosteriorMean()¶
Accessor to the output observations.
- Returns:
- outputAtPosterior
Sample
Output at posterior mean.
- outputAtPosterior
- getOutputAtPriorMean()¶
Accessor to the output observations.
- Returns:
- outputAtPrior
Sample
Output at prior mean.
- outputAtPrior
- getOutputObservations()¶
Accessor to the output observations.
- Returns:
- outputObservations
Sample
The sample of output observations.
- outputObservations
- getParameterMAP()¶
Accessor to the maximum a posteriori parameter estimate.
- Returns:
- parameterPosterior
Point
The maximum a posteriori parameter estimate.
- parameterPosterior
- getParameterPosterior()¶
Accessor to the parameter posterior distribution.
- Returns:
- parameterPosterior
Distribution
The posterior distribution of the parameter.
- parameterPosterior
- getParameterPrior()¶
Accessor to the parameter prior distribution.
- Returns:
- parameterPrior
Distribution
The prior distribution of the parameter.
- parameterPrior
- getResidualFunction()¶
Accessor to the residual function.
- Returns:
- residualFunction
Function
The residual function.
- residualFunction
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- setInputObservations(inputObservations)¶
Accessor to the input observations.
- Parameters:
- inputObservations
Sample
The sample of input observations.
- inputObservations
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
- setObservationsError(observationsError)¶
Accessor to the observations error distribution.
- Parameters:
- observationsError
Distribution
The observations error distribution.
- observationsError
- setOutputAtPriorAndPosteriorMean(outputAtPriorMean, outputAtPosteriorMean)¶
Accessor to the output at prior/posterior mean.
- setOutputObservations(outputObservations)¶
Accessor to the output observations.
- Parameters:
- outputObservations
Sample
The sample of output observations.
- outputObservations
- setParameterMAP(parameterMAP)¶
Accessor to the maximum a posteriori parameter estimate.
- Parameters:
- parameterPosteriorsequence of float
The maximum a posteriori parameter estimate.
- setParameterPosterior(parameterPosterior)¶
Accessor to the parameter posterior distribution.
- Parameters:
- parameterPosterior:
Distribution
The posterior distribution of the parameter.
- parameterPosterior:
- setParameterPrior(parameterPrior)¶
Accessor to the parameter prior distribution.
- Parameters:
- parameterPrior:
Distribution
The prior distribution of the parameter.
- parameterPrior:
Examples using the class¶
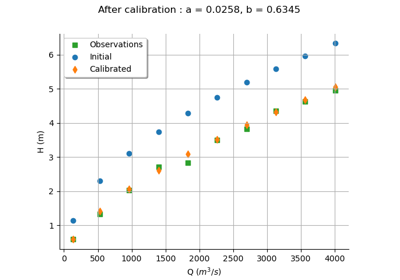
Calibrate a parametric model: a quick-start guide to calibration