KarhunenLoeveReduction¶
(Source code
, png
)
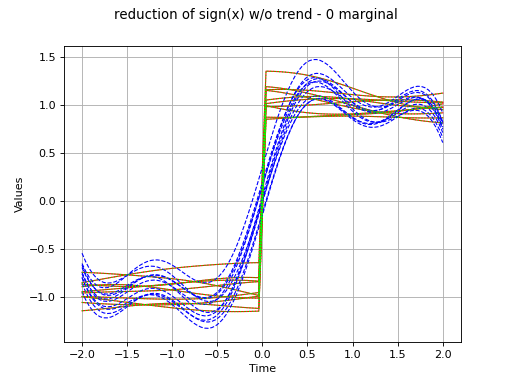
- class KarhunenLoeveReduction(*args)¶
Perform the reduction of a field.
This object projects a field on the Karhunen-Loeve basis by computing the coefficients, lifts the coefficients, combines them with the value of the modes on the mesh which creates the reduced field.
- Parameters:
- result
KarhunenLoeveResult
Decomposition result.
- trend
TrendTransform
, optional Process trend, useful when the basis built using the covariance function from the space of trajectories is not well suited to approximate the mean function of the underlying process.
- result
Methods
Get the number of calls of the function.
Accessor to the object's name.
Get the description of the input field values.
Get the dimension of the input field values.
Get the mesh associated to the input domain.
getMarginal
(*args)Get the marginal(s) at given indice(s).
getName
()Accessor to the object's name.
Get the description of the output field values.
Get the dimension of the output field values.
Get the mesh associated to the output domain.
hasName
()Test if the object is named.
Whether the function acts point-wise.
setInputDescription
(inputDescription)Set the description of the input field values.
setInputMesh
(inputMesh)Set the mesh associated to the input domain.
setName
(name)Accessor to the object's name.
setOutputDescription
(outputDescription)Set the description of the output field values.
setOutputMesh
(outputMesh)Set the mesh associated to the output domain.
setTrend
(trend)Trend accessor.
See also
Examples
Create a KL decomposition of a Gaussian process:
>>> import openturns as ot >>> numberOfVertices = 10 >>> interval = ot.Interval(-1.0, 1.0) >>> mesh = ot.IntervalMesher([numberOfVertices - 1]).build(interval) >>> covariance = ot.SquaredExponential() >>> process = ot.GaussianProcess(covariance, mesh) >>> sampleSize = 10 >>> sample = process.getSample(sampleSize) >>> threshold = 0.0 >>> algo = ot.KarhunenLoeveSVDAlgorithm(sample, threshold) >>> algo.run() >>> klresult = algo.getResult()
Generate some trajectories and reduce them:
>>> sample2 = process.getSample(5) >>> reduction = ot.KarhunenLoeveReduction(klresult) >>> reduced = reduction(sample2)
Same, but into account the trend:
>>> trend = ot.TrendTransform(ot.P1LagrangeEvaluation(sample.computeMean()), mesh) >>> reduction = ot.KarhunenLoeveReduction(klresult, trend) >>> reduced = reduction(sample2)
- __init__(*args)¶
- getCallsNumber()¶
Get the number of calls of the function.
- Returns:
- callsNumberint
Counts the number of times the function has been called since its creation.
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getInputDescription()¶
Get the description of the input field values.
- Returns:
- inputDescription
Description
Description of the input field values.
- inputDescription
- getInputDimension()¶
Get the dimension of the input field values.
- Returns:
- dint
Dimension
of the input field values.
- getMarginal(*args)¶
Get the marginal(s) at given indice(s).
- Parameters:
- iint or list of ints,
Indice(s) of the marginal(s) to be extracted.
- iint or list of ints,
- Returns:
- fieldFunction
FieldFunction
The initial function restricted to the concerned marginal(s) at the indice(s)
.
- fieldFunction
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getOutputDescription()¶
Get the description of the output field values.
- Returns:
- outputDescription
Description
Description of the output field values.
- outputDescription
- getOutputDimension()¶
Get the dimension of the output field values.
- Returns:
- d’int
Dimension
of the output field values.
- getOutputMesh()¶
Get the mesh associated to the output domain.
- Returns:
- outputMesh
Mesh
The output mesh
.
- outputMesh
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- isActingPointwise()¶
Whether the function acts point-wise.
- Returns:
- pointWisebool
Returns true if the function evaluation at each vertex depends only on the vertex or the value at the vertex.
- setInputDescription(inputDescription)¶
Set the description of the input field values.
- Parameters:
- inputDescriptionsequence of str
Description of the input field values.
- setInputMesh(inputMesh)¶
Set the mesh associated to the input domain.
- Parameters:
- inputMesh
Mesh
The input mesh
.
- inputMesh
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
- setOutputDescription(outputDescription)¶
Set the description of the output field values.
- Parameters:
- outputDescriptionsequence of str
Describes the outputs of the output field values.
- setOutputMesh(outputMesh)¶
Set the mesh associated to the output domain.
- Parameters:
- outputMesh
Mesh
The output mesh
.
- outputMesh
- setTrend(trend)¶
Trend accessor.
- Parameters:
- trend
TrendTransform
, optional Process trend, useful when the basis built using the covariance function from the space of trajectories is not well suited to approximate the mean function of the underlying process.
- trend