CauchyModel¶
(Source code
, png
)
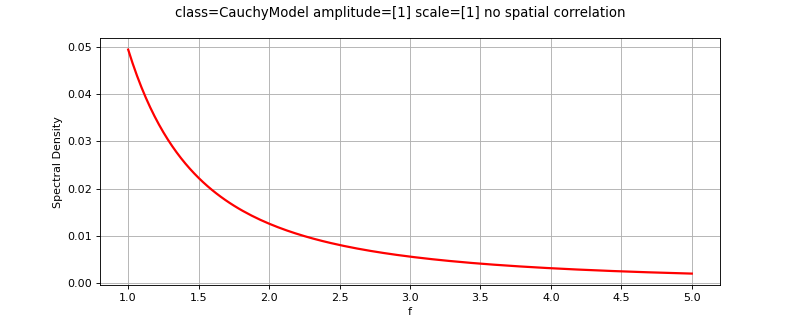
- class CauchyModel(*args)¶
Cauchy spectral model.
Refer to Parametric spectral density functions.
- Parameters:
- thetasequence of float
Scale coefficients
of the spectral density function. Vector of size n
- sigmasequence of float
Amplitude coefficients
of the spectral density function. Vector of size p
Methods
computeStandardRepresentative
(frequency)Compute the standard representant of the spectral density function.
draw
(*args)Draw a specific component of the spectral density function.
Get the amplitude parameter of the spectral density function.
Accessor to the object's name.
Get the input dimension of the spectral density function.
getName
()Accessor to the object's name.
Get the spatial correlation matrix of the spectral density function.
Get the dimension of the SpectralModel.
getScale
()Get the scale parameter of the spectral density function.
hasName
()Test if the object is named.
setAmplitude
(amplitude)Set the amplitude parameter of the spectral density function.
setName
(name)Accessor to the object's name.
setScale
(scale)Set the scale parameter of the spectral density function.
Notes
The spectral density function of input dimension n and output dimension p writes:
Examples
>>> import openturns as ot >>> spectralModel = ot.CauchyModel([3.0, 2.0], [2.0]) >>> f = 0.3 >>> print(spectralModel(f)) [[ (0.191364,0) ]] >>> f = 10 >>> print(spectralModel(f)) [[ (1.71084e-07,0) ]]
- __init__(*args)¶
- computeStandardRepresentative(frequency)¶
Compute the standard representant of the spectral density function.
- Parameters:
- taufloat
Frequency value.
- Returns:
- rhoComplex
Standard representant factor of the spectral density function.
Notes
Using definitions in
SpectralModel
: the standard representative function writes:where
- draw(*args)¶
Draw a specific component of the spectral density function.
- Parameters:
- rowIndexint,
The row index of the component to draw. Default value is 0.
- columnIndex: int, :math:`0 leq columnIndex < dimension`
The column index of the component to draw. Default value is 0.
- minimumFrequencyfloat
The lower bound of the frequency range over which the model is plotted. Default value is SpectralModel-DefaultMinimumFrequency in
ResourceMap
.- maximumFrequencyfloat
The upper bound of the frequency range over which the model is plotted. Default value is SpectralModel-DefaultMaximumFrequency in
ResourceMap
.- frequencyNumberint,
The discretization of the frequency range
over which the model is plotted. Default value is SpectralModel-DefaultFrequencyNumber in class:~openturns.ResourceMap.
- modulebool
Flag to tell if module has to be drawn (True) or if it is the argument to be drawn (False). Default value is True.
- rowIndexint,
- Returns:
- graphGraph
Graphic of the specified component
- getAmplitude()¶
Get the amplitude parameter of the spectral density function.
- Returns:
- amplitude
Point
The used amplitude parameter.
- amplitude
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getInputDimension()¶
Get the input dimension of the spectral density function.
- Returns:
- inputDimensionint
SpatialDimension of the SpectralModel.
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getOutputCorrelation()¶
Get the spatial correlation matrix of the spectral density function.
- Returns:
- spatialCorrelation
CorrelationMatrix
Correlation matrix
.
- spatialCorrelation
- getOutputDimension()¶
Get the dimension of the SpectralModel.
- Returns:
- dimensionint
Dimension of the SpectralModel.
- getScale()¶
Get the scale parameter of the spectral density function.
- Returns:
- scale
Point
The used scale parameter.
- scale
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- setAmplitude(amplitude)¶
Set the amplitude parameter of the spectral density function.
- Parameters:
- amplitude
Point
The amplitude parameter to be used in the spectral density function.
- amplitude
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.