Indices¶
- class Indices(*args)¶
Collection of unsigned integers.
- Available constructors:
Indices(size=0, value=0)
Indices(sequence)
- Parameters:
- sizeint,
Size of the collection.
- valuepositive int
Value set to the size elements.
- sequencesequence of int
Components of the vector.
- sizeint,
Examples
>>> import openturns as ot
Use the first constructor:
>>> ot.Indices(3) [0,0,0] >>> ot.Indices(3, 4) [4,4,4]
Use the second constructor:
>>> vector = ot.Indices([100, 30, 70]) >>> vector [100,30,70]
Use some functionalities:
>>> vector[1] = 20 >>> vector [100,20,70] >>> vector.add(50) >>> vector [100,20,70,50]
The Indices class has a method that can search for an element and return True if the element is in the indices, and False otherwise. We can access to it using the in keyword.
>>> indices = ot.Indices([3, 5, 7, 9]) >>> print(0 in indices) False >>> print(3 in indices) True
Methods
add
(*args)Append a component (in-place).
at
(*args)Access to an element of the collection.
check
(bound)Check that no value is repeated and no value exceeds the given bound.
clear
()Reset the collection to zero dimension.
complement
(n)Build the complement of the current indices wrt
.
fill
([initialValue, stepSize])Fill the indices with a linear progression.
find
(val)Find the index of a given value.
Accessor to the object's name.
getName
()Accessor to the object's name.
getSize
()Get the collection's dimension (or size).
hasName
()Test if the object is named.
isEmpty
()Tell if the collection is empty.
Check if the indices are increasing.
norm1
()Compute the 1-norm of the indices.
normInf
()Compute the infinite norm of the indices.
resize
(newSize)Change the size of the collection.
select
(marginalIndices)Selection from indices.
setName
(name)Accessor to the object's name.
- __init__(*args)¶
- add(*args)¶
Append a component (in-place).
- Parameters:
- valuetype depends on the type of the collection.
The component to append.
Examples
>>> import openturns as ot >>> x = ot.Point(2) >>> x.add(1.) >>> print(x) [0,0,1]
- at(*args)¶
Access to an element of the collection.
- Parameters:
- indexpositive int
Position of the element to access.
- Returns:
- elementtype depends on the type of the collection
Element of the collection at the position index.
- check(bound)¶
Check that no value is repeated and no value exceeds the given bound.
- Parameters:
- boundpositive int
The bound value.
- Returns:
- checkbool
True if no value is repeated and all values are < bound.
- clear()¶
Reset the collection to zero dimension.
Examples
>>> import openturns as ot >>> x = ot.Point(2) >>> x.clear() >>> x class=Point name=Unnamed dimension=0 values=[]
- complement(n)¶
Build the complement of the current indices wrt
.
- Parameters:
- boundpositive int
The value of
.
- Returns:
- complement
Indices
The increasing collection of integers in
not in the current indices.
- complement
Examples
>>> import openturns as ot >>> indices = ot.Indices([1, 3, 4]) >>> print(indices.complement(7)) [0,2,5,6]
- fill(initialValue=0, stepSize=1)¶
Fill the indices with a linear progression.
Starting from the start value initialValue by step stepSize.
- Parameters:
- initialValuepositive int
Initial value. By default it is equal to 0.
- stepSizepositive int
Step size. By default it is equal to 1.
Examples
>>> import openturns as ot >>> indices = ot.Indices(3) >>> indices.fill() >>> print(indices) [0,1,2] >>> indices = ot.Indices(3) >>> indices.fill(2, 4) >>> print(indices) [2,6,10]
- find(val)¶
Find the index of a given value.
- Parameters:
- valcollection value type
The value to find
- Returns:
- indexint
The index of the first occurrence of the value, or the size of the container if not found. When several values match, only the first index is returned.
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getSize()¶
Get the collection’s dimension (or size).
- Returns:
- nint
The number of components in the collection.
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- isEmpty()¶
Tell if the collection is empty.
- Returns:
- isEmptybool
True if there is no element in the collection.
Examples
>>> import openturns as ot >>> x = ot.Point(2) >>> x.isEmpty() False >>> x.clear() >>> x.isEmpty() True
- isIncreasing()¶
Check if the indices are increasing.
- Returns:
- isIncreasingbool
True if the indices are increasing.
Examples
>>> import openturns as ot >>> indices = ot.Indices(3) >>> indices.fill() >>> indices.isIncreasing() True
- norm1()¶
Compute the 1-norm of the indices.
- Returns:
- norm: int
The sum of the indices.
Examples
>>> import openturns as ot >>> indices = ot.Indices([3, 5, 7, 9]) >>> print(indices.norm1()) 24
- normInf()¶
Compute the infinite norm of the indices.
- Returns:
- norm: int
The maximum of the indices.
Examples
>>> import openturns as ot >>> indices = ot.Indices([3, 5, 7, 9]) >>> print(indices.normInf()) 9
- resize(newSize)¶
Change the size of the collection.
- Parameters:
- newSizepositive int
New size of the collection.
Notes
If the new size is smaller than the older one, the last elements are thrown away, else the new elements are set to the default value of the element type.
Examples
>>> import openturns as ot >>> x = ot.Point(2, 4) >>> print(x) [4,4] >>> x.resize(1) >>> print(x) [4] >>> x.resize(4) >>> print(x) [4,0,0,0]
- select(marginalIndices)¶
Selection from indices.
- Parameters:
- indicessequence of int
Indices to select
- Returns:
- collsequence
Sub-collection of values at the selection indices.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
Examples using the class¶
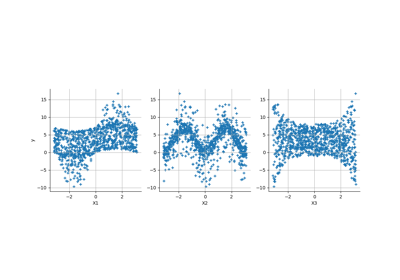
Create a polynomial chaos for the Ishigami function: a quick start guide to polynomial chaos