FieldToPointFunctionalChaosAlgorithm¶
- class FieldToPointFunctionalChaosAlgorithm(*args)¶
Functional metamodel algorithm based on chaos decomposition.
Warning
This class is experimental and likely to be modified in future releases. To use it, import the
openturns.experimental
submodule.The present algorithm allows one to build a response surface of the application
of the form:
The application
is known from
fields
of the associated input process
and
vectors
that fully characterize the output vector
The linear projection function
of the Karhunen-Loeve decomposition by SVD is used to project the input fields, see
KarhunenLoeveSVDAlgorithm
for the notations.The Karhunen-Loeve algorithm allows one to replace this integral by a specific weighted and finite sum and to write the projections of the j-th marginal of i-th input field
by multiplication with the projection matrix
:
with
the retained number of modes in the decomposition of the j-th input. The projections of all the
components of
fields are assembled in the
matrix:
with
the total number of modes across input components.
Then a functional chaos decomposition is built between the projected modes sample
and the output samples
, see
FunctionalChaosAlgorithm
for details.with
the number of terms in the chaos decomposition.
The final metamodel consists in the composition of the Karhunen-Loeve projections and the functional chaos metamodel.
A limitation of this approach is that the projected modes sample has a dimension
so the dimension of the input fields
and the associated number of modes must remain modest (curse of dimensionality).
- Parameters:
- x
ProcessSample
Input process sample.
- y
Sample
Output sample.
- x
Methods
BuildDistribution
(modesSample)Build the distribution of Karhunen-Loeve coefficients.
Accessor to the block indices.
Accessor to centered sample flag.
Accessor to the object's name.
Accessor to the input sample.
getName
()Accessor to the object's name.
Accessor to the maximum number of modes to compute.
Accessor to the output sample.
Accessor to the recompression flag.
Result accessor.
Accessor to the eigenvalues cutoff ratio.
hasName
()Test if the object is named.
run
()Compute the response surfaces.
setBlockIndices
(blockIndices)Accessor to the block indices.
setCenteredSample
(centered)Accessor to centered sample flag.
setName
(name)Accessor to the object's name.
setNbModes
(nbModes)Accessor to the maximum number of modes to compute.
setRecompress
(recompress)Accessor to the recompression flag.
setThreshold
(threshold)Accessor to the eigenvalues cutoff ratio.
See also
Notes
As the input process decomposition is done with the values decomposition approach, it is possible to control the number of modes retained per input, the idea being to avoid a large input dimension for the chaos decomposition step. As done in
KarhunenLoeveSVDAlgorithm
, thesetThreshold()
andsetNbModes()
methods allow one to control the spectrum ratio and maximum count.By default the input process data is assumed to not be centered, and the method
setCenteredSample()
allows one to skip centering of the data for both the computation of modes coefficients and inside the metamodel.In the case of homogenous data (if variables have the same unit or scale), it is also possible to recompress the modes at the global level with
setRecompress()
. When enabled, the eigenvalues are gathered and sorted so as to find a global spectrum cut-off value by which the spectrum of each input is truncated. The default value can be set through theResourceMap
key FieldToPointFunctionalChaosAlgorithm-DefaultRecompress.For the chaos metamodel step, it is possible to specify the basis size with the
ResourceMap
key FunctionalChaosAlgorithm-BasisSize.It is possible to group input variables by independent blocks with
setBlockIndices()
, this way Karhunen-Loeve process decompositions are done on each block rather than on each variable thus reducing the total number of modes and help reduce the chaos input dimension.Examples
>>> import openturns as ot >>> import openturns.experimental as otexp >>> ot.RandomGenerator.SetSeed(0) >>> mesh = ot.RegularGrid(0.0, 0.1, 20) >>> cov = ot.KroneckerCovarianceModel(ot.MaternModel([2.0], 1.5), ot.CovarianceMatrix(4)) >>> X = ot.GaussianProcess(cov, mesh) >>> x = X.getSample(500) >>> y = [[m[0] + m[1] + m[2] - m[3] + m[0] * m[1] - m[2] * m[3] - 0.1 * m[0] * m[1] * m[2]] for m in [xi.computeMean() for xi in x]] >>> algo = otexp.FieldToPointFunctionalChaosAlgorithm(x, y) >>> algo.setThreshold(4e-2) >>> # Temporarily lower the basis size for the sake of this example. >>> ot.ResourceMap.SetAsUnsignedInteger('FunctionalChaosAlgorithm-BasisSize', 100) >>> algo.run() >>> result = algo.getResult() >>> metamodel = result.getFieldToPointMetaModel() >>> y0hat = metamodel(x[0]) >>> ot.ResourceMap.Reload()
- __init__(*args)¶
- static BuildDistribution(modesSample)¶
Build the distribution of Karhunen-Loeve coefficients.
This method aims at building a possibly parametric representation of Karhunen-Loeve coefficients based on their Gaussian or independent theorical properties in order to build efficient metamodels. When this is not possible it falls back to non-parametric representations for the marginals and the dependence structure.
- Parameters:
- modesSample
Sample
Karhunen-Loeve modes sample.
- modesSample
- Returns:
- distribution
Distribution
Distribution of coefficients.
- distribution
Notes
The strategy for marginals is to test for a
Normal
hypothesis via theCramerVonMisesNormal()
test, else fallback toHistogram
.For the dependence structure it tests for an
IndependentCopula
thanks to theSpearman()
test, else fallback to the Normal or Beta copula (seeEmpiricalBernsteinCopula
) according to theResourceMap
key FieldToPointFunctionalChaosAlgorithm-CopulaType.
- getBlockIndices()¶
Accessor to the block indices.
- Returns:
- blockIndices
IndicesCollection
Independent components indices.
- blockIndices
- getCenteredSample()¶
Accessor to centered sample flag.
- Parameters:
- centeredbool
Whether the input sample is centered. When set to True, it allows one to skip centering of the input process data.
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getInputProcessSample()¶
Accessor to the input sample.
- Returns:
- inputSample
ProcessSample
Input sample.
- inputSample
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getNbModes()¶
Accessor to the maximum number of modes to compute.
- Returns:
- nint
The maximum number of modes to compute. The actual number of modes also depends on the threshold criterion.
- getRecompress()¶
Accessor to the recompression flag.
- Returns:
- recompressbool
Whether to recompress the input Karhunen-Loeve decompositions. This can only be enabled if the scale of the input variable blocks is the same.
- getResult()¶
Result accessor.
- Returns:
- result
openturns.experimental.FieldFunctionalChaosResult
Result class.
- result
- getThreshold()¶
Accessor to the eigenvalues cutoff ratio.
- Returns:
- sfloat,
The threshold
.
- sfloat,
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- run()¶
Compute the response surfaces.
Notes
It computes the response surfaces and creates a
MetaModelResult
structure containing all the results.
- setBlockIndices(blockIndices)¶
Accessor to the block indices.
- Parameters:
- blockIndices2-d sequence of int
Independent components indices.
- setCenteredSample(centered)¶
Accessor to centered sample flag.
- Parameters:
- centeredbool
Whether the input sample is centered. When set to True, it allows one to skip centering of the input process data.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
- setNbModes(nbModes)¶
Accessor to the maximum number of modes to compute.
- Parameters:
- nint
The maximum number of modes to compute. The actual number of modes also depends on the threshold criterion.
- setRecompress(recompress)¶
Accessor to the recompression flag.
- Parameters:
- recompressbool
Whether to recompress the input Karhunen-Loeve decompositions. The modes are truncated a second time according to a global eigen value bound across input decompositions. This can only be enabled if the scale of the input variable blocks is the same.
- setThreshold(threshold)¶
Accessor to the eigenvalues cutoff ratio.
- Parameters:
- sfloat,
The threshold
.
- sfloat,
Examples using the class¶
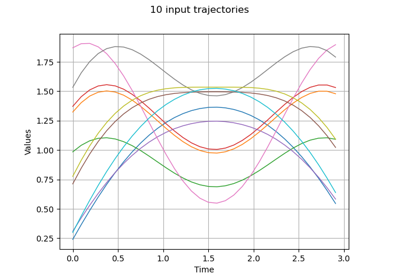
Estimate Sobol indices on a field to point function