Note
Go to the end to download the full example code.
Simulate an FMU
The Otfmi simulate
function instanciates, initializes and simulates the
FMU.
First, retrieve and load the FMU deviation.fmu.
import openturns as ot
import otfmi.example.utility
import matplotlib.pyplot as plt
path_fmu = otfmi.example.utility.get_path_fmu("deviation")
model = otfmi.fmi.load_fmu(path_fmu)
Note
model is a PyFMI object, loaded with Otfmi’s overlay.
As such,
model.simulate()
is a pure PyFMI method.Use
otfmi.fmi.simulate(model)
to benefit from Otfmi’s overlay.Otfmi simulate
function notably eases initializing a FMU, see
Initialize an FMU with non-default values.
On top of the initialization keywords, PyFMI simulation keywords can be employed:
result = otfmi.fmi.simulate(
model,
start_time=0, # PyFMI keyword
final_time=1, # PyFMI keyword
initialization_parameters=(["L"], [300]),
) # Otfmi keyword
print("y = %g" % result.final("y"))
Simulation interval : 0 - 1.0 seconds.
Elapsed simulation time: 0.013243385000009766 seconds.
y = 22.5
We can use these keyword to plot the deviation Y as function of the beam length L:
inputSample = ot.RegularGrid(1.0, 10.0, 10).getValues()
list_output = []
for length in inputSample:
result = otfmi.fmi.simulate(model, initialization_parameters=(["L"], [length]))
list_output.append(result.final("y"))
outputSample = ot.Sample([[xx] for xx in list_output])
plt.figure()
plt.plot(inputSample, outputSample)
plt.show()
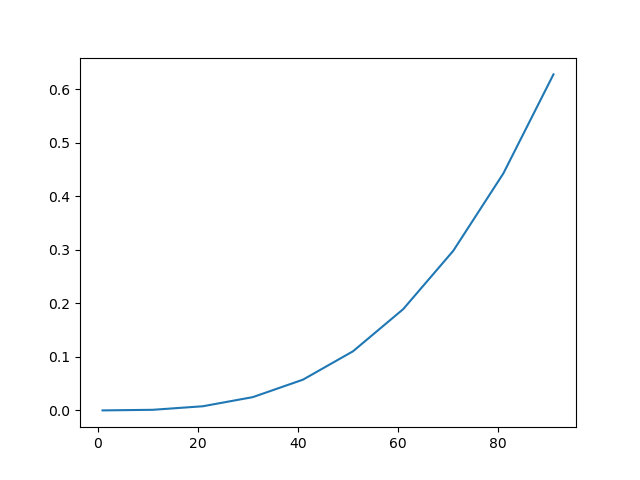
Simulation interval : 0.0 - 1.0 seconds.
Elapsed simulation time: 0.013004206999994494 seconds.
Simulation interval : 0.0 - 1.0 seconds.
Elapsed simulation time: 0.013082754999970803 seconds.
Simulation interval : 0.0 - 1.0 seconds.
Elapsed simulation time: 0.01317126100002497 seconds.
Simulation interval : 0.0 - 1.0 seconds.
Elapsed simulation time: 0.013007023000000117 seconds.
Simulation interval : 0.0 - 1.0 seconds.
Elapsed simulation time: 0.013051877000009426 seconds.
Simulation interval : 0.0 - 1.0 seconds.
Elapsed simulation time: 0.016068996000001334 seconds.
Simulation interval : 0.0 - 1.0 seconds.
Elapsed simulation time: 0.01298415000002251 seconds.
Simulation interval : 0.0 - 1.0 seconds.
Elapsed simulation time: 0.013103293999961352 seconds.
Simulation interval : 0.0 - 1.0 seconds.
Elapsed simulation time: 0.013034564000008686 seconds.
Simulation interval : 0.0 - 1.0 seconds.
Elapsed simulation time: 0.013171101000011731 seconds.
The interest of the higher-level functions are:
- avoid the for loop on the points of the design of experiment,
- automatic formatting of the simulation outputs.
Total running time of the script: (0 minutes 0.238 seconds)