Time series manipulationΒΆ
The objective here is to create and manipulate a time series. A time series is a particular field where the mesh 1-d and regular, eg a time grid
.
It is possible to draw a time series, using interpolation between the values: see the use case on the Field.
A time series can be obtained as a realization of a multivariate stochastic process of dimension
where
is discretized according to the regular grid
. The values
of the time series are defined by:
A time series is stored in the TimeSeries object that stores the regular time grid and the associated values.
[1]:
from __future__ import print_function
import openturns as ot
import math as m
[2]:
# Create the RegularGrid
tMin = 0.
timeStep = 0.1
N = 100
myTimeGrid = ot.RegularGrid(tMin, timeStep, N)
[15]:
# Case 1: Create a time series from a time grid and values
# Care! The number of steps of the time grid must correspond to the size of the values
myValues = ot.Normal(3).getSample(myTimeGrid.getVertices().getSize())
myTimeSeries = ot.TimeSeries(myTimeGrid, myValues)
myTimeSeries
[15]:
[ t X0 X1 X2 ]
0 : [ 0 2.31733 -0.469879 -0.801703 ]
1 : [ 0.1 -0.0108486 -0.0553915 -1.7548 ]
2 : [ 0.2 -0.894538 -0.360249 -0.770978 ]
3 : [ 0.3 0.55883 1.62925 1.34049 ]
4 : [ 0.4 0.494815 -0.0180664 -0.0365295 ]
5 : [ 0.5 -1.01369 1.03588 -0.12276 ]
6 : [ 0.6 -0.177026 1.4058 0.0981434 ]
7 : [ 0.7 1.01776 0.833219 2.12309 ]
8 : [ 0.8 -1.65891 1.91107 -0.499354 ]
9 : [ 0.9 -0.707992 -0.836693 -0.08448 ]
10 : [ 1 -0.0772068 -0.754153 1.35719 ]
11 : [ 1.1 -0.262538 0.43282 -1.88328 ]
12 : [ 1.2 1.1694 -0.0711754 -1.57923 ]
13 : [ 1.3 1.07961 0.818312 -0.769237 ]
14 : [ 1.4 -1.63959 -2.49198 0.392813 ]
15 : [ 1.5 1.54502 -0.592087 2.36899 ]
16 : [ 1.6 -0.81979 -0.103824 -0.98551 ]
17 : [ 1.7 0.987314 -0.346144 -1.34504 ]
18 : [ 1.8 1.07011 0.927288 -0.155148 ]
19 : [ 1.9 -0.461614 1.68461 0.712465 ]
20 : [ 2 0.929992 -0.483778 -0.174459 ]
21 : [ 2.1 0.662006 -0.698763 -0.0269453 ]
22 : [ 2.2 -1.28184 0.386923 0.152988 ]
23 : [ 2.3 -0.467685 -0.514194 0.384462 ]
24 : [ 2.4 0.261137 -0.578211 -1.33245 ]
25 : [ 2.5 0.864973 0.941687 0.329529 ]
26 : [ 2.6 0.0412185 0.141166 -0.0393573 ]
27 : [ 2.7 0.27118 -0.383442 0.760954 ]
28 : [ 2.8 0.0351336 -0.295452 0.13985 ]
29 : [ 2.9 -0.863809 1.23659 1.40946 ]
30 : [ 3 0.681355 -0.220565 1.25437 ]
31 : [ 3.1 -0.583736 -1.26391 -0.235933 ]
32 : [ 3.2 -0.633479 2.00508 -1.06159 ]
33 : [ 3.3 -0.973466 0.182045 0.466031 ]
34 : [ 3.4 -2.404 -0.675804 -0.43258 ]
35 : [ 3.5 0.615584 0.946263 0.254775 ]
36 : [ 3.6 -0.173574 0.741881 -0.0834884 ]
37 : [ 3.7 -1.23036 -1.46178 -0.519229 ]
38 : [ 3.8 1.90887 -1.15193 1.02327 ]
39 : [ 3.9 0.169987 -0.0977635 -0.423342 ]
40 : [ 4 -0.638673 -0.260154 -1.43577 ]
41 : [ 4.1 1.15734 -1.18448 -0.242118 ]
42 : [ 4.2 -1.75983 0.224354 -0.497826 ]
43 : [ 4.3 0.894821 0.595231 -0.248979 ]
44 : [ 4.4 0.0926772 -0.824599 0.824924 ]
45 : [ 4.5 -1.64514 -0.313514 1.07257 ]
46 : [ 4.6 -0.281387 1.01541 -0.343512 ]
47 : [ 4.7 1.73504 -1.54906 -0.570394 ]
48 : [ 4.8 -0.634918 0.397189 -0.45656 ]
49 : [ 4.9 -0.853559 0.0403166 -0.299548 ]
50 : [ 5 0.450826 0.0339842 -0.428601 ]
51 : [ 5.1 0.877138 0.262852 0.269669 ]
52 : [ 5.2 -0.86813 -0.323261 -0.719086 ]
53 : [ 5.3 -1.67268 -0.0197131 -1.12035 ]
54 : [ 5.4 -0.597611 -0.330729 -0.0366483 ]
55 : [ 5.5 -0.0950169 0.912443 1.13421 ]
56 : [ 5.6 0.159206 -0.617572 1.47189 ]
57 : [ 5.7 1.41735 -0.150357 -0.463105 ]
58 : [ 5.8 -1.24986 -1.85139 1.21562 ]
59 : [ 5.9 -0.383809 -0.135983 -0.966214 ]
60 : [ 6 3.0788 -0.710827 -0.216468 ]
61 : [ 6.1 -0.226418 0.0258637 0.921209 ]
62 : [ 6.2 0.0419902 0.103587 0.249475 ]
63 : [ 6.3 -0.667934 0.12365 -0.14698 ]
64 : [ 6.4 -0.271669 0.485002 0.201355 ]
65 : [ 6.5 -0.259562 0.00503527 -1.99632 ]
66 : [ 6.6 -0.735897 0.244483 -1.93819 ]
67 : [ 6.7 0.553995 -0.879113 1.10496 ]
68 : [ 6.8 0.533898 0.043558 -0.593256 ]
69 : [ 6.9 0.955203 1.44755 -0.420213 ]
70 : [ 7 -1.02309 -0.567824 -0.632136 ]
71 : [ 7.1 -0.671702 -0.562391 0.0522118 ]
72 : [ 7.2 0.567886 -0.804104 -1.0274 ]
73 : [ 7.3 0.0207672 1.36971 0.726692 ]
74 : [ 7.4 1.2459 1.99545 -0.41103 ]
75 : [ 7.5 0.266026 1.93123 0.98723 ]
76 : [ 7.6 0.407197 0.223757 -0.806872 ]
77 : [ 7.7 -0.880356 -1.38558 1.47653 ]
78 : [ 7.8 0.487294 -0.616603 -0.270488 ]
79 : [ 7.9 2.22406 0.934046 1.08349 ]
80 : [ 8 0.412462 -1.05112 -0.246497 ]
81 : [ 8.1 0.236328 0.880083 -0.623114 ]
82 : [ 8.2 -1.05763 -0.325425 0.72565 ]
83 : [ 8.3 -1.21886 0.220351 2.01841 ]
84 : [ 8.4 0.759168 2.49498 0.803174 ]
85 : [ 8.5 -1.08808 -0.321161 2.63821 ]
86 : [ 8.6 -0.66262 -0.645395 -0.440328 ]
87 : [ 8.7 1.31516 0.381884 0.226424 ]
88 : [ 8.8 0.346054 0.743799 -1.39891 ]
89 : [ 8.9 0.124891 0.507658 -0.102776 ]
90 : [ 9 0.191792 -0.310295 0.0640703 ]
91 : [ 9.1 -1.09636 -0.302375 0.387491 ]
92 : [ 9.2 -1.44132 -0.573967 -0.098096 ]
93 : [ 9.3 0.230417 -1.3587 -0.984013 ]
94 : [ 9.4 1.16441 -0.634025 0.0382606 ]
95 : [ 9.5 0.67274 -0.22672 0.504295 ]
96 : [ 9.6 -1.35244 -0.0812011 0.472061 ]
97 : [ 9.7 1.42725 0.112269 -0.870752 ]
98 : [ 9.8 -0.247597 0.139603 1.04401 ]
99 : [ 9.9 0.0305908 -0.845472 -0.273887 ]
[4]:
# Case 2: Get a time series from a Process
myProcess = ot.WhiteNoise(ot.Normal(3), myTimeGrid)
myTimeSeries2 = myProcess.getRealization()
myTimeSeries2
[4]:
[ t X0 X1 X2 ]
0 : [ 0 1.73945 0.935939 1.27511 ]
1 : [ 0.1 -0.595004 -0.0230083 1.85337 ]
2 : [ 0.2 0.356188 -1.31765 -1.195 ]
3 : [ 0.3 -0.443684 -0.826204 -0.748816 ]
4 : [ 0.4 0.434034 -0.644208 -0.559902 ]
5 : [ 0.5 0.0546682 -0.0564792 0.757535 ]
6 : [ 0.6 -0.663158 0.683646 0.591043 ]
7 : [ 0.7 -2.20872 -0.779031 -0.703086 ]
8 : [ 0.8 -0.0566955 0.588101 0.738839 ]
9 : [ 0.9 0.727128 -1.1831 0.853199 ]
10 : [ 1 1.03198 0.104467 0.51551 ]
11 : [ 1.1 -1.73251 0.369231 0.667104 ]
12 : [ 1.2 -0.51281 -0.477754 1.26264 ]
13 : [ 1.3 1.57846 1.89005 0.390297 ]
14 : [ 1.4 0.235759 0.244948 0.57169 ]
15 : [ 1.5 -0.420542 0.553255 -2.19382 ]
16 : [ 1.6 0.421279 0.753758 -0.512419 ]
17 : [ 1.7 -0.306225 -1.21103 0.0174802 ]
18 : [ 1.8 -1.16501 0.0643894 0.717609 ]
19 : [ 1.9 -0.304268 -0.391375 0.258215 ]
20 : [ 2 -1.49078 1.06182 0.374969 ]
21 : [ 2.1 -0.165467 0.357871 0.885118 ]
22 : [ 2.2 1.7379 -0.713196 1.70642 ]
23 : [ 2.3 0.428636 -0.277595 0.411295 ]
24 : [ 2.4 0.370445 0.187854 1.43266 ]
25 : [ 2.5 -0.416128 -0.122868 0.950488 ]
26 : [ 2.6 -0.411128 -0.98049 0.564196 ]
27 : [ 2.7 -1.55666 0.624888 1.05797 ]
28 : [ 2.8 -0.769529 1.54237 0.374802 ]
29 : [ 2.9 0.506105 0.419768 1.58128 ]
30 : [ 3 0.00956375 -0.38302 0.163699 ]
31 : [ 3.1 1.2561 0.00620397 0.272004 ]
32 : [ 3.2 -0.153784 -0.404166 2.09224 ]
33 : [ 3.3 0.675043 -0.383208 -0.355239 ]
34 : [ 3.4 -1.3053 -1.51577 0.172158 ]
35 : [ 3.5 0.57768 -0.173049 0.662172 ]
36 : [ 3.6 0.697838 0.789578 0.479307 ]
37 : [ 3.7 -0.449433 1.7657 0.281658 ]
38 : [ 3.8 0.127981 -0.747966 0.512891 ]
39 : [ 3.9 1.08613 -0.551952 1.57891 ]
40 : [ 4 -0.222394 0.205503 1.33851 ]
41 : [ 4.1 0.045253 2.15649 1.00985 ]
42 : [ 4.2 1.18898 1.28765 -0.279847 ]
43 : [ 4.3 -0.567342 0.0789431 -0.374098 ]
44 : [ 4.4 0.36891 -0.216249 -0.964493 ]
45 : [ 4.5 1.14255 0.271953 -0.62676 ]
46 : [ 4.6 -1.24554 -0.793869 0.808652 ]
47 : [ 4.7 0.551898 1.26214 -0.398731 ]
48 : [ 4.8 -2.13505 0.420672 -0.164956 ]
49 : [ 4.9 -1.27789 -0.748335 0.447628 ]
50 : [ 5 1.6065 -1.23832 -0.857113 ]
51 : [ 5.1 -0.796012 2.55533 -1.40966 ]
52 : [ 5.2 0.161757 1.07153 -0.0756152 ]
53 : [ 5.3 -0.38581 -0.695899 -0.240171 ]
54 : [ 5.4 0.0140339 -1.32783 -0.421805 ]
55 : [ 5.5 -1.31457 -0.314242 0.870097 ]
56 : [ 5.6 -1.05029 -0.661749 -0.398957 ]
57 : [ 5.7 -0.306869 2.24097 -1.84225 ]
58 : [ 5.8 -1.12629 -0.343091 1.35707 ]
59 : [ 5.9 -1.14144 0.719911 0.691288 ]
60 : [ 6 0.0157415 0.897854 -0.0268917 ]
61 : [ 6.1 -1.17253 -1.22323 1.33729 ]
62 : [ 6.2 -0.412087 1.40508 0.839941 ]
63 : [ 6.3 -0.171826 -0.612136 0.43319 ]
64 : [ 6.4 1.25989 -2.15135 0.638385 ]
65 : [ 6.5 1.1986 0.576051 -2.72106 ]
66 : [ 6.6 -0.456634 0.834452 0.189723 ]
67 : [ 6.7 -1.5599 0.141706 -0.312877 ]
68 : [ 6.8 -1.09669 1.30628 -0.603543 ]
69 : [ 6.9 0.0844715 -1.01157 -0.45413 ]
70 : [ 7 0.184323 1.11103 0.0332655 ]
71 : [ 7.1 -0.402727 0.812135 0.137958 ]
72 : [ 7.2 -0.552396 0.712298 0.657798 ]
73 : [ 7.3 -0.2971 0.288142 -0.843501 ]
74 : [ 7.4 1.4814 0.904859 0.90054 ]
75 : [ 7.5 0.211378 0.408217 -0.290709 ]
76 : [ 7.6 -1.03768 0.403674 -0.0361247 ]
77 : [ 7.7 -0.0439957 1.57857 1.44503 ]
78 : [ 7.8 -0.908343 -1.329 -0.476214 ]
79 : [ 7.9 1.02238 -1.19788 2.59603 ]
80 : [ 8 0.149855 -0.390626 -0.311655 ]
81 : [ 8.1 -0.451449 0.237096 0.624386 ]
82 : [ 8.2 -0.5553 0.765645 0.509255 ]
83 : [ 8.3 0.416132 -1.42826 -0.155242 ]
84 : [ 8.4 1.19865 -0.189811 -1.09981 ]
85 : [ 8.5 0.727195 0.566469 -1.437 ]
86 : [ 8.6 -0.253593 0.898235 0.732674 ]
87 : [ 8.7 -0.109548 -0.485969 -0.35672 ]
88 : [ 8.8 -1.25939 0.0349764 -0.409638 ]
89 : [ 8.9 0.795517 -2.10814 -0.914884 ]
90 : [ 9 1.09502 -0.0632852 2.00363 ]
91 : [ 9.1 -0.124881 0.0604086 -0.449676 ]
92 : [ 9.2 0.15909 -2.04317 1.06144 ]
93 : [ 9.3 -1.44415 0.751101 0.844058 ]
94 : [ 9.4 -1.23908 0.743606 1.43464 ]
95 : [ 9.5 0.0770002 -2.18739 0.237613 ]
96 : [ 9.6 0.805032 0.784197 1.02133 ]
97 : [ 9.7 -0.35903 -1.16653 1.01787 ]
98 : [ 9.8 0.275839 0.907344 0.359928 ]
99 : [ 9.9 1.17828 1.57853 2.0749 ]
[5]:
# Get the number of values of the time series
myTimeSeries.getSize()
[5]:
100
[6]:
# Get the dimension of the values observed at each time
myTimeSeries.getMesh().getDimension()
[6]:
1
[7]:
# Get the value Xi at index i
i = 37
print('Xi = ', myTimeSeries.getValueAtIndex(i))
Xi = [-0.488205,-0.465482,0.332084]
[8]:
# Get the time series at index i : (ti, Xi)
i = 37
print('(ti, Xi) = ', myTimeSeries[i])
(ti, Xi) = [-0.488205,-0.465482,0.332084]
[9]:
# Get a the marginal value at index i of the time series
i = 37
# get the time stamp:
print('ti = ', myTimeSeries[i, 0])
# get the first component of the corresponding value :
print('Xi1 = ', myTimeSeries[i, 1])
ti = -0.4882047479037244
Xi1 = -0.46548206392049335
[10]:
# Get all the values (X1, .., Xn) of the time series
myTimeSeries.getValues()
[10]:
X0 | X1 | X2 | |
---|---|---|---|
0 | 0.6082016512187646 | -1.2661731022166567 | -0.43826561996041397 |
1 | 1.2054782008285756 | -2.1813852346165143 | 0.3500420865302907 |
2 | -0.3550070491856397 | 1.437249310140903 | 0.8106679824694837 |
3 | 0.79315601145977 | -0.4705255986325704 | 0.26101793529769673 |
4 | -2.2900619818700854 | -1.2828852904549808 | -1.311781115463341 |
5 | -0.09078382658049489 | 0.9957932259165571 | -0.13945281896393122 |
6 | -0.5602056000378475 | 0.4454896972990519 | 0.32292503034661274 |
7 | 0.44578529818450985 | -1.0380765948630941 | -0.8567122780208447 |
8 | 0.4736169171884015 | -0.12549774541256004 | 0.35141776801611424 |
9 | 1.7823586399387168 | 0.070207359297043 | -0.7813664602347197 |
10 | -0.7215334320191932 | -0.24122348956683715 | -1.7879641991225605 |
11 | 0.4013597437727011 | 1.367825523115209 | 1.0043431266393292 |
12 | 0.7415483840772669 | -0.043612337965035816 | 0.5393446780678338 |
13 | 0.2999504132732002 | 0.40771715787264406 | -0.48511195560000947 |
14 | -0.38299201615804984 | -0.7528165991274193 | 0.25792642458131404 |
15 | 1.9687596027732095 | -0.6712905330814658 | 1.8557922404430598 |
16 | 0.05215932560232187 | 0.790445824639787 | 0.7163525542248972 |
17 | -0.7436220201526069 | 0.18435600073165376 | -1.530734483444384 |
18 | 0.6550274609707977 | 0.5380714996799529 | 1.738212597715617 |
19 | -0.9587222803460276 | 0.3779221311281753 | -0.1810041948595859 |
20 | 1.6729651981107247 | -1.0389584433401373 | -0.3535524450885604 |
21 | 1.2138135523904243 | -0.7770331122619051 | -1.3685314491601195 |
22 | 0.1034744243555785 | -0.8918195408099401 | 0.905601680864366 |
23 | 0.33479445398050983 | -0.4836415989409058 | 0.6779582665237674 |
24 | 1.7093788628314481 | 1.07062031445028 | -0.506924729621585 |
25 | -1.6608639532729972 | 2.2462287040616475 | 0.7596015459405085 |
26 | -0.510763820034195 | -0.6330662015316597 | -0.9570721500415863 |
27 | 0.5440465956139983 | 0.8145606872090909 | -0.734708377960548 |
28 | -0.11146080893135524 | 0.9944818782078243 | -0.16062532311409344 |
29 | -0.9387705743377833 | -1.968691542444217 | -0.6576034692788746 |
30 | 0.3387510861377688 | 1.0155577081272245 | 0.6371672460165964 |
31 | -0.08990711915988879 | -0.8558864367182618 | 1.2712829469472742 |
32 | -0.2382525840159452 | 1.3262988324629563 | 2.119675592985771 |
33 | -0.9015813901152602 | -1.5169648184616176 | -1.2993795893316618 |
34 | 0.23037243903915736 | -3.097374220265686 | 0.013229996975342417 |
35 | -1.2574295730740137 | 1.0277604824132867 | -0.7664306747065759 |
36 | 0.21751211240280865 | 1.0453331498743215 | 0.3315688008777966 |
37 | -0.4882047479037244 | -0.46548206392049335 | 0.33208385645183086 |
38 | -0.16772582180258397 | 3.012626743702593 | 0.9420404910001581 |
39 | 0.6118901404192247 | 0.6117151564272073 | -1.5374973590145564 |
40 | -2.406701632898191 | 0.6629359832717704 | -0.6561602005666336 |
41 | -0.7516114592509675 | 0.4381769975654701 | -0.4553345919601327 |
42 | 1.8603777479857533 | 0.21972123726271536 | 1.725462994560952 |
43 | -0.5434054870737179 | -0.7367488371221942 | -0.5082064350443634 |
44 | -2.258672040864401 | -0.5963998333071584 | -0.3146800263075891 |
45 | -1.782738221104927 | -0.6847337811346507 | 0.061115707883683305 |
46 | 0.8737197289205034 | -1.4629534274978957 | -0.3187856285938157 |
47 | 1.263141994155593 | -0.42672559757717077 | -1.8923429557066818 |
48 | -0.5143905498504552 | 0.6472293778560264 | 0.0037024864831827547 |
49 | 0.7296877765012115 | -0.2472337723951604 | 0.4791910385893783 |
50 | -0.03360982636241379 | -0.03672706471415314 | 0.11025616355141858 |
51 | -0.3768703589700057 | -0.09558941438605024 | 0.10912241770686365 |
52 | -0.198754145656359 | 0.4736195376455026 | 0.16163728851497228 |
53 | 0.3844829079369488 | 0.11646763738621115 | -0.10008045244292718 |
54 | 1.491564303161293 | 1.2230054393315812 | 0.526646331161638 |
55 | -0.6569234219822457 | -0.13122822803845577 | -1.453470810242808 |
56 | 1.1741448211259624 | 0.9293947008749075 | -0.3371134579838932 |
57 | 0.5786882960372883 | -0.5824589478380001 | -1.388861072987769 |
58 | -0.4997483415305477 | -1.5551578034867246 | 0.48308301365700856 |
59 | 0.20500419543073403 | -0.09725248024468533 | 0.5925630820144326 |
60 | -0.6020444666415793 | -1.2100861975899475 | -0.8866978667261813 |
61 | -0.14111369030042462 | 0.4419834280530504 | 0.5191620132847282 |
62 | -1.5145511107546767 | -0.6769173970437052 | 0.6676776291109286 |
63 | -1.4058454178947792 | -0.02953347109931314 | -0.6318286705576991 |
64 | -0.34215693175874956 | 2.0533857387192125 | 1.1587027449019125 |
65 | -1.457170091897887 | -0.8443667458052184 | -0.28861027376722903 |
66 | 0.4192711129639974 | -0.8360643654354077 | 0.8582685706406981 |
67 | -0.9065659143816405 | -0.9168098650471502 | 1.1632207448691083 |
68 | 0.30191831259808755 | 0.49033126865016113 | 0.4754245747137407 |
69 | -0.7887042563466362 | -0.6694491869900636 | -0.1379279554775434 |
70 | -0.9715312223420239 | -1.1878377947381524 | 1.428203280692695 |
71 | -0.5892298619089735 | -1.7321759670632966 | 0.8249934440800486 |
72 | 3.0279901522056356 | 1.6947972773244453 | -1.6482672001700636 |
73 | -0.9964693298966726 | 0.7731214398905927 | -0.5194757998273593 |
74 | -0.03519734280661111 | -0.43986558279364807 | -0.259332190949003 |
75 | -0.8754189647636796 | -2.5398626160475524 | -0.056670934740152897 |
76 | -0.021727906394664178 | 0.5992195203104552 | 0.1468678550666206 |
77 | -0.7453603509332029 | -0.5215960035243518 | 0.5920202454509629 |
78 | -0.4700387195138181 | -2.172109456702745 | -0.43261732336113257 |
79 | 0.2677501726668674 | -0.367989726099373 | 1.1484168621665602 |
80 | -0.034328296438353126 | 0.46108184356376175 | -0.6224243706780185 |
81 | -1.6250556819326551 | -0.543099206860823 | -0.2695348828774449 |
82 | 0.02088179929670331 | 0.6238537218570768 | 0.7671373238839353 |
83 | 0.8887983653765579 | 1.4803058800425652 | 0.6610018495637071 |
84 | 1.408952186863387 | 0.5761247344316582 | 1.8932649144636948 |
85 | 0.8586112321424536 | -0.9073478946471832 | -0.5375026761534027 |
86 | -0.6384336188895413 | 1.348563813060152 | -2.2660834614598864 |
87 | 0.4232323917550925 | -0.9961412477351472 | -1.087506356723854 |
88 | 0.11107999528804083 | 0.677663085654946 | -1.0550178569789195 |
89 | -0.004096591671766315 | 0.56283328125277 | -0.029615966971302244 |
90 | 0.07020645731636742 | -0.235269945857301 | -1.2903078142197937 |
91 | -1.0186404579648396 | -1.7113094070040313 | 0.9433259509800255 |
92 | -0.5423194949602862 | -0.9991112989354737 | -1.4045695396487468 |
93 | 1.9460618018795466 | 0.7795719081491099 | 1.1384752363617938 |
94 | 0.711147617175394 | -0.4533857740632992 | 0.6183193642974254 |
95 | 0.7220435333040667 | 0.6600207498477129 | 0.4659188068304761 |
96 | -0.40772976610396877 | 1.4591902726984554 | -0.4115654109111582 |
97 | 0.5494386852470321 | 1.4501932963738728 | -0.327249469535079 |
98 | -1.3979567876733754 | 1.301150630693703 | -0.48525934352978206 |
99 | -0.2724066372034578 | -0.33882294820034387 | -0.7907566133559019 |
[11]:
# Compute the temporal Mean
# It corresponds to the mean of the values of the time series
myTimeSeries.getInputMean()
[11]:
[-0.025392,-0.027327,-0.0146978]
[12]:
# Draw the marginal i of the time series using linear interpolation
myTimeSeries.drawMarginal(0)
[12]:
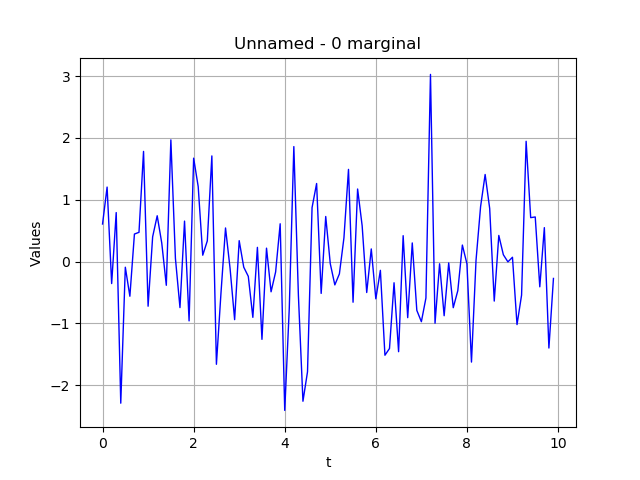
[13]:
# with no interpolation
myTimeSeries.drawMarginal(0, False)
[13]:
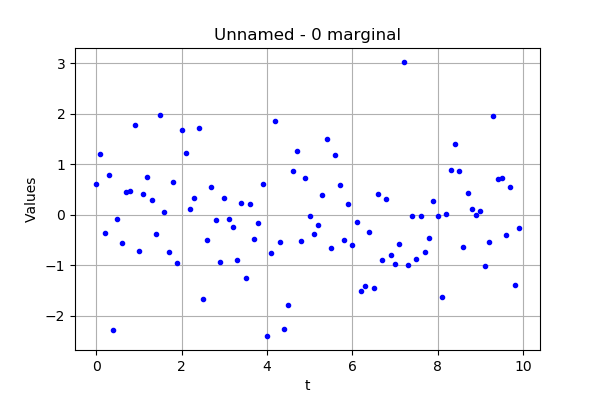