FisherSnedecorFactory¶
(Source code, png, hires.png, pdf)
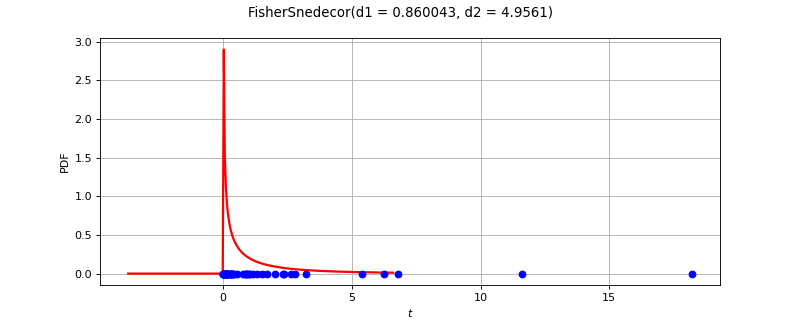
-
class
FisherSnedecorFactory
(*args)¶ Fisher-Snedecor factory.
- Available constructor:
FisherSnedecorFactory()
See also
Notes
Several estimators to build a FisherSnedecor distribution from a scalar sample are proposed.
Maximum likelihood estimator:
The parameters are estimated by numerical maximum likelihood estimation. The starting point of the optimization algorithm is based on the moment based estimator.
The optimization sets lower bounds for the
and
parameters in order to ensure that
and
. The default values for these lower bounds are from the
ResourceMap
keys FisherSnedecorFactory-D1LowerBound and FisherSnedecorFactory-D2LowerBound.Moment based estimator:
Lets denote:
the empirical mean of the sample,
its empirical variance,
We first compute
:
if
(otherwise, the moment based estimator fails).
Then we compute
:
if
(otherwise, the moment based estimator fails).
Examples
In the following example, the parameters of a
FisherSnedecor
are estimated from a sample.>>> import openturns as ot >>> ot.RandomGenerator.SetSeed(0) >>> size = 10000 >>> distribution = ot.FisherSnedecor(4.5, 8.4) >>> sample = distribution.getSample(size) >>> factory = ot.TruncatedNormalFactory() >>> estimated = factory.build(sample) >>> estimated = factory.buildMethodOfMoments(sample) >>> estimated = factory.buildMethodOfLikelihoodMaximization(sample)
Methods
build
(self, \*args)Estimate the distribution using the default strategy.
buildAsFisherSnedecor
(self, \*args)Estimate the distribution using the default strategy.
buildEstimator
(self, \*args)Build the distribution and the parameter distribution.
buildMethodOfLikelihoodMaximization
(self, sample)Method of likelihood maximization.
buildMethodOfMoments
(self, sample)Method of moments estimator.
getBootstrapSize
(self)Accessor to the bootstrap size.
getClassName
(self)Accessor to the object’s name.
getId
(self)Accessor to the object’s id.
getName
(self)Accessor to the object’s name.
getShadowedId
(self)Accessor to the object’s shadowed id.
getVisibility
(self)Accessor to the object’s visibility state.
hasName
(self)Test if the object is named.
hasVisibleName
(self)Test if the object has a distinguishable name.
setBootstrapSize
(self, bootstrapSize)Accessor to the bootstrap size.
setName
(self, name)Accessor to the object’s name.
setShadowedId
(self, id)Accessor to the object’s shadowed id.
setVisibility
(self, visible)Accessor to the object’s visibility state.
-
__init__
(self, \*args)¶ Initialize self. See help(type(self)) for accurate signature.
-
build
(self, \*args)¶ Estimate the distribution using the default strategy.
- Parameters
- sample
Sample
Data
- sample
- Returns
- distribution
Distribution
The estimated distribution
- distribution
Notes
The default strategy is using the maximum likelihood estimators.
-
buildAsFisherSnedecor
(self, \*args)¶ Estimate the distribution using the default strategy.
Available usages:
buildAsFisherSnedecor(sample)
buildAsFisherSnedecor(param)
- Parameters
- sample2-d sequence of float
Sample from which the distribution parameters are estimated.
- paramCollection of
PointWithDescription
A vector of parameters of the distribution.
- Returns
- dist
FisherSnedecor
The built distribution.
- dist
Notes
The default strategy is using the maximum likelihood estimators.
-
buildEstimator
(self, \*args)¶ Build the distribution and the parameter distribution.
- Parameters
- sample2-d sequence of float
Sample from which the distribution parameters are estimated.
- parameters
DistributionParameters
Optional, the parametrization.
- Returns
- resDist
DistributionFactoryResult
The results.
- resDist
Notes
According to the way the native parameters of the distribution are estimated, the parameters distribution differs:
Moments method: the asymptotic parameters distribution is normal and estimated by Bootstrap on the initial data;
Maximum likelihood method with a regular model: the asymptotic parameters distribution is normal and its covariance matrix is the inverse Fisher information matrix;
Other methods: the asymptotic parameters distribution is estimated by Bootstrap on the initial data and kernel fitting (see
KernelSmoothing
).
If another set of parameters is specified, the native parameters distribution is first estimated and the new distribution is determined from it:
if the native parameters distribution is normal and the transformation regular at the estimated parameters values: the asymptotic parameters distribution is normal and its covariance matrix determined from the inverse Fisher information matrix of the native parameters and the transformation;
in the other cases, the asymptotic parameters distribution is estimated by Bootstrap on the initial data and kernel fitting.
-
buildMethodOfLikelihoodMaximization
(self, sample)¶ Method of likelihood maximization.
Refer to
MaximumLikelihoodFactory
.- Parameters
- sample
Sample
Data
- sample
- Returns
- distribution
FisherSnedecor
The estimated distribution
- distribution
-
buildMethodOfMoments
(self, sample)¶ Method of moments estimator.
- Parameters
- sample
Sample
Data
- sample
- Returns
- distribution
FisherSnedecor
The estimated distribution
- distribution
-
getBootstrapSize
(self)¶ Accessor to the bootstrap size.
- Returns
- sizeinteger
Size of the bootstrap.
-
getClassName
(self)¶ Accessor to the object’s name.
- Returns
- class_namestr
The object class name (object.__class__.__name__).
-
getId
(self)¶ Accessor to the object’s id.
- Returns
- idint
Internal unique identifier.
-
getName
(self)¶ Accessor to the object’s name.
- Returns
- namestr
The name of the object.
-
getShadowedId
(self)¶ Accessor to the object’s shadowed id.
- Returns
- idint
Internal unique identifier.
-
getVisibility
(self)¶ Accessor to the object’s visibility state.
- Returns
- visiblebool
Visibility flag.
-
hasName
(self)¶ Test if the object is named.
- Returns
- hasNamebool
True if the name is not empty.
-
hasVisibleName
(self)¶ Test if the object has a distinguishable name.
- Returns
- hasVisibleNamebool
True if the name is not empty and not the default one.
-
setBootstrapSize
(self, bootstrapSize)¶ Accessor to the bootstrap size.
- Parameters
- sizeinteger
Size of the bootstrap.
-
setName
(self, name)¶ Accessor to the object’s name.
- Parameters
- namestr
The name of the object.
-
setShadowedId
(self, id)¶ Accessor to the object’s shadowed id.
- Parameters
- idint
Internal unique identifier.
-
setVisibility
(self, visible)¶ Accessor to the object’s visibility state.
- Parameters
- visiblebool
Visibility flag.