EnumerateFunction¶
- class EnumerateFunction(*args)¶
Enumerate function.
Methods
getBasisSizeFromTotalDegree
(maximumDegree)Get the basis size corresponding to a total degree.
Accessor to the object's name.
Return the dimension of the EnumerateFunction.
getId
()Accessor to the object's id.
Accessor to the underlying implementation.
getMarginal
(*args)Get the marginal enumerate function.
getMaximumDegreeCardinal
(maximumDegree)Get the number of multi-indices of total degree lower or equal to a threshold.
getMaximumDegreeStrataIndex
(maximumDegree)Get the largest index of the strata containing multi-indices lower or equal to the given maximum degree.
getName
()Accessor to the object's name.
getStrataCardinal
(strataIndex)Get the number of multi-indices in the basis inside a given strata.
getStrataCumulatedCardinal
(strataIndex)Get the number of multi-indices in the basis inside a range of stratas.
Accessor to the upper bound.
inverse
(indices)Get the antecedent of a indices list in the EnumerateFunction.
setDimension
(dimension)Set the dimension of the EnumerateFunction.
setName
(name)Accessor to the object's name.
setUpperBound
(upperBound)Accessor to the upper bound.
Notes
EnumerateFunction is a bijection
from
to
. This bijection is based on a particular enumeration rule of the set of multi-indices. For any integer
, the value of the enumerate function is:
The first multi-index is:
Let
be any pair of indices. If
then:
where
is the k-th component of the multi-index
. This provides a necessary but insufficient condition for the construction of the bijection: a supplementary hypothesis must be made.
For example, consider the dimension
. The following mapping is an enumerate function:
For the functional expansion (respectively polynomial chaos expansion), the multi-index
is the collection of marginal degrees of the selected orthogonal functions (respectively orthogonal polynomials). More precisely, after the selection of the type of orthogonal functions (respectively orthogonal polynomials) for the construction of the orthogonal basis, the EnumerateFunction defines the marginal degrees of the univariate functions (respectively univariate polynomials). The multivariate tensor product function is based on the product of marginal univariate functions:
for any point
where
is the polynomial of degree
for the i-th marginal. The total degree of the polynomial is equal to the sum of the integers in the multi-index:
For example, consider the multi-index
. This multi-index defines a multivariate polynomial which has a input vector of dimension 3. The marginal degree of the polynomial of the first dimension is equal to 2, the marginal degree of the polynomial of the second dimension is equal to 0 and the marginal degree of the polynomial of the third dimension is equal to 3.
Examples
>>> import openturns as ot >>> dim = 2 >>> enum_func = ot.LinearEnumerateFunction(dim) >>> for i in range(6): ... print(enum_func(i)) [0,0] [1,0] [0,1] [2,0] [1,1] [0,2]
- __init__(*args)¶
- getBasisSizeFromTotalDegree(maximumDegree)¶
Get the basis size corresponding to a total degree.
- Parameters:
- max_degint
Maximum total degree.
- Returns:
- sizeint
Number of multi-indices in the basis of total degree
.
Notes
In the specific context of a linear enumeration (
LinearEnumerateFunction
) this is also the cumulated cardinal of stratas up to max_deg.Examples
>>> import openturns as ot >>> dim = 2 >>> enum_func = ot.LinearEnumerateFunction(dim) >>> enum_func.getBasisSizeFromTotalDegree(3) 10 >>> enum_func.getStrataCumulatedCardinal(3) 10
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getDimension()¶
Return the dimension of the EnumerateFunction.
- Returns:
- dimint,
Dimension of the EnumerateFunction.
- dimint,
- getId()¶
Accessor to the object’s id.
- Returns:
- idint
Internal unique identifier.
- getImplementation()¶
Accessor to the underlying implementation.
- Returns:
- implImplementation
A copy of the underlying implementation object.
- getMarginal(*args)¶
Get the marginal enumerate function.
- Parameters:
- indicesint or sequence of int,
List of marginal indices.
- indicesint or sequence of int,
- Returns:
- enumerateFunction
EnumerateFunction
The marginal enumerate function.
- enumerateFunction
- getMaximumDegreeCardinal(maximumDegree)¶
Get the number of multi-indices of total degree lower or equal to a threshold.
- Parameters:
- max_degint
Maximum total degree.
- Returns:
- cardinalint
Number of multi-indices in the basis of total degree
.
Notes
In the specific context of a linear enumeration (
LinearEnumerateFunction
) this is also the cumulated cardinal of stratas of index.
Examples
>>> import openturns as ot >>> dim = 2 >>> enum_func = ot.LinearEnumerateFunction(dim) >>> enum_func.getMaximumDegreeCardinal(2) 6
- getMaximumDegreeStrataIndex(maximumDegree)¶
Get the largest index of the strata containing multi-indices lower or equal to the given maximum degree.
- Parameters:
- max_degint
Maximum total degree.
- Returns:
- indexint
Index of the last strata that contains multi-indices of total degree
.
Notes
In the specific context of a linear enumeration (
LinearEnumerateFunction
) this is the strata of index max_deg.Examples
>>> import openturns as ot >>> dim = 2 >>> enum_func = ot.LinearEnumerateFunction(dim) >>> enum_func.getMaximumDegreeStrataIndex(2) 2
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getStrataCardinal(strataIndex)¶
Get the number of multi-indices in the basis inside a given strata.
- Parameters:
- strataIndexint
Index of the strata of the tensorized basis.
- Returns:
- cardinalint
Number of multi-indices in the basis inside the strata strataIndex.
Notes
In the specific context of a linear enumeration (
LinearEnumerateFunction
) the strata strataIndex consists of a hyperplane of all the multi-indices of total degree strataIndex, and its cardinal is strataIndex + 1.Examples
>>> import openturns as ot >>> dim = 2 >>> enum_func = ot.LinearEnumerateFunction(dim) >>> enum_func.getStrataCardinal(2) 3
- getStrataCumulatedCardinal(strataIndex)¶
Get the number of multi-indices in the basis inside a range of stratas.
- Parameters:
- strataIndexint
Index of the strata of the tensorized basis.
- Returns:
- cardinalint
Number of multi-indices in the basis inside the stratas of index lower or equal to strataIndex.
Notes
The number of multi-indices is the total of multi-indices inside the stratas. In the specific context of a linear enumeration (
LinearEnumerateFunction
) this returns the number of multi-indices of maximal total degree strataIndex.Examples
>>> import openturns as ot >>> dim = 2 >>> enum_func = ot.LinearEnumerateFunction(dim) >>> enum_func.getStrataCumulatedCardinal(2) 6 >>> sum([enum_func.getStrataCardinal(i) for i in range(3)]) 6
- getUpperBound()¶
Accessor to the upper bound.
- Returns:
- ubsequence of int
Upper bound of the indices (inclusive).
- inverse(indices)¶
Get the antecedent of a indices list in the EnumerateFunction.
- Parameters:
- multiIndexsequence of int
List of indices.
- Returns:
- antecedentint
Represents the antecedent of the multiIndex in the EnumerateFunction.
Examples
>>> import openturns as ot >>> dim = 2 >>> enum_func = ot.LinearEnumerateFunction(dim) >>> for i in range(6): ... print(str(i)+' '+str(enum_func(i))) 0 [0,0] 1 [1,0] 2 [0,1] 3 [2,0] 4 [1,1] 5 [0,2] >>> print(enum_func.inverse([1,1])) 4
- setDimension(dimension)¶
Set the dimension of the EnumerateFunction.
- Parameters:
- dimint,
Dimension of the EnumerateFunction.
- dimint,
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
- setUpperBound(upperBound)¶
Accessor to the upper bound.
- Parameters:
- ubsequence of int
Upper bound of the indices (inclusive).
Examples using the class¶
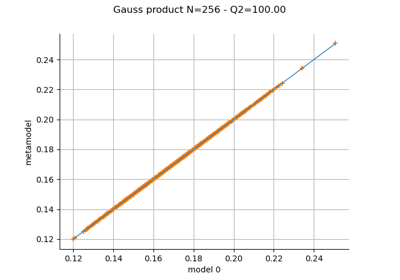
Create a polynomial chaos metamodel by integration on the cantilever beam
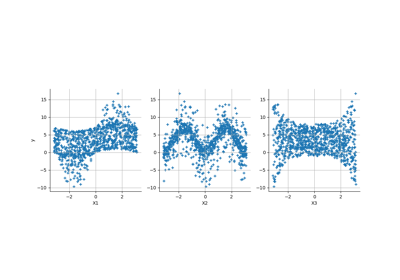
Create a polynomial chaos for the Ishigami function: a quick start guide to polynomial chaos
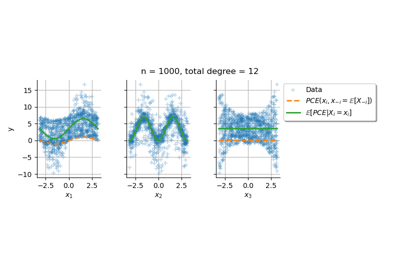
Conditional expectation of a polynomial chaos expansion
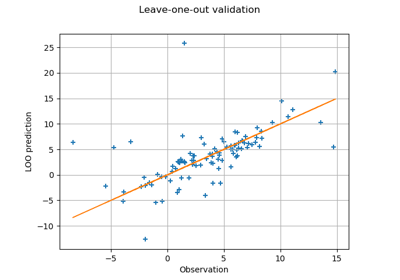
Compute leave-one-out error of a polynomial chaos expansion