LeastSquaresMetaModelSelectionFactory¶
- class LeastSquaresMetaModelSelectionFactory(*args)¶
Least squares metamodel selection factory.
- Parameters:
- basisSeqFac
BasisSequenceFactory
A basis sequence factory.
- fittingAlgo
FittingAlgorithm
, optional A fitting algorithm.
- basisSeqFac
Notes
Implementation of an approximation algorithm implementation factory which builds an
ApproximationAlgorithm
.This class is not usable because it is operational only within the
FunctionalChaosAlgorithm
.Examples
>>> import openturns as ot >>> basisSequenceFactory = ot.LARS() >>> fittingAlgorithm = ot.CorrectedLeaveOneOut() >>> approximationAlgorithm = ot.LeastSquaresMetaModelSelectionFactory( ... basisSequenceFactory, fittingAlgorithm)
Methods
build
(x, y, weight, psi, indices)Build the approximation.
Accessor to the basis sequence factory.
Accessor to the object's name.
Accessor to the fitting algorithm.
getName
()Accessor to the object's name.
hasName
()Test if the object is named.
Get the model selection flag.
setName
(name)Accessor to the object's name.
- __init__(*args)¶
- build(x, y, weight, psi, indices)¶
Build the approximation.
- Parameters:
- x2-d sequence of float
The input random observations
where
is the input of the physical model,
is the input dimension and
is the sample size.
- y2-d sequence of float
The output random observations
where
is the output of the physical model,
is the output dimension and
is the sample size.
- weightsequence of float
Weights associated to the input sample points such that the corresponding weighted experiment is a good approximation of
, where
is the distribution of the standard random vector
associated with the physical input random vector
. If unspecified, all weights are equal to
, where
is the size of the sample.
- psisequence of
Function
The functional basis.
- indicessequence of int
Indices in the basis.
- Returns:
- algorithm:
ApproximationAlgorithm
The estimation algorithm.
- algorithm:
- getBasisSequenceFactory()¶
Accessor to the basis sequence factory.
- Returns:
- basis
BasisSequenceFactory
Basis sequence factory.
- basis
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getFittingAlgorithm()¶
Accessor to the fitting algorithm.
- Returns:
- algo
FittingAlgorithm
Fitting algorithm.
- algo
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- hasName()¶
Test if the object is named.
- Returns:
- hasNamebool
True if the name is not empty.
- involvesModelSelection()¶
Get the model selection flag.
A model selection method can be used to select the coefficients of the decomposition which enable to best predict the output. Model selection leads to a sparse functional chaos expansion.
- Returns:
- involvesModelSelection: bool
True if the method involves a model selection method.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
Examples using the class¶
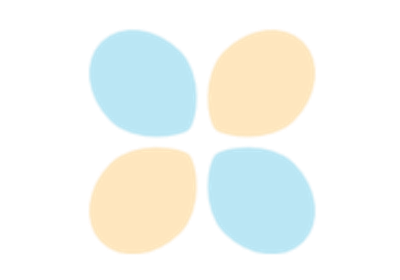
Create a full or sparse polynomial chaos expansion
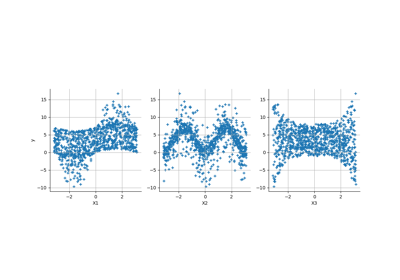
Create a polynomial chaos for the Ishigami function: a quick start guide to polynomial chaos
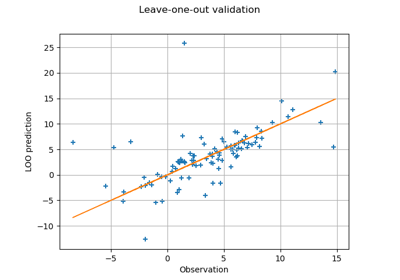
Compute leave-one-out error of a polynomial chaos expansion