RandomVector¶
- class RandomVector(*args)¶
Random vectors.
- Parameters:
- distribution
Distribution
Distribution of the
UsualRandomVector
to define.
- distribution
See also
Notes
A
RandomVector
provides at least a way to generate realizations.Methods
Accessor to the antecedent RandomVector in case of a composite RandomVector.
Accessor to the object's name.
Accessor to the covariance of the RandomVector.
Accessor to the description of the RandomVector.
Accessor to the dimension of the RandomVector.
Accessor to the distribution of the RandomVector.
Accessor to the domain of the Event.
getFrozenRealization
(fixedPoint)Compute realizations of the RandomVector.
getFrozenSample
(fixedSample)Compute realizations of the RandomVector.
Accessor to the Function in case of a composite RandomVector.
getId
()Accessor to the object's id.
Accessor to the underlying implementation.
getMarginal
(*args)Get the random vector corresponding to the
marginal component(s).
getMean
()Accessor to the mean of the RandomVector.
getName
()Accessor to the object's name.
Accessor to the comparaison operator of the Event.
Accessor to the parameter of the distribution.
Accessor to the parameter description of the distribution.
Compute one realization of the RandomVector.
getSample
(size)Compute realizations of the RandomVector.
Accessor to the threshold of the Event.
intersect
(other)Intersection of two events.
Accessor to know if the RandomVector is a composite one.
isEvent
()Whether the random vector is an event.
join
(other)Union of two events.
setDescription
(description)Accessor to the description of the RandomVector.
setName
(name)Accessor to the object's name.
setParameter
(parameters)Accessor to the parameter of the distribution.
- __init__(*args)¶
- getAntecedent()¶
Accessor to the antecedent RandomVector in case of a composite RandomVector.
- Returns:
- antecedent
RandomVector
Antecedent RandomVector
in case of a
CompositeRandomVector
such as:.
- antecedent
- getClassName()¶
Accessor to the object’s name.
- Returns:
- class_namestr
The object class name (object.__class__.__name__).
- getCovariance()¶
Accessor to the covariance of the RandomVector.
- Returns:
- covariance
CovarianceMatrix
Covariance of the considered
UsualRandomVector
.
- covariance
Examples
>>> import openturns as ot >>> distribution = ot.Normal([0.0, 0.5], [1.0, 1.5], ot.CorrelationMatrix(2)) >>> randomVector = ot.RandomVector(distribution) >>> ot.RandomGenerator.SetSeed(0) >>> print(randomVector.getCovariance()) [[ 1 0 ] [ 0 2.25 ]]
- getDescription()¶
Accessor to the description of the RandomVector.
- Returns:
- description
Description
Describes the components of the RandomVector.
- description
- getDimension()¶
Accessor to the dimension of the RandomVector.
- Returns:
- dimensionpositive int
Dimension of the RandomVector.
- getDistribution()¶
Accessor to the distribution of the RandomVector.
- Returns:
- distribution
Distribution
Distribution of the considered
UsualRandomVector
.
- distribution
Examples
>>> import openturns as ot >>> distribution = ot.Normal([0.0, 0.0], [1.0, 1.0], ot.CorrelationMatrix(2)) >>> randomVector = ot.RandomVector(distribution) >>> ot.RandomGenerator.SetSeed(0) >>> print(randomVector.getDistribution()) Normal(mu = [0,0], sigma = [1,1], R = [[ 1 0 ] [ 0 1 ]])
- getDomain()¶
Accessor to the domain of the Event.
- Returns:
- domain
Domain
Describes the domain of an event.
- domain
- getFrozenRealization(fixedPoint)¶
Compute realizations of the RandomVector.
In the case of a
CompositeRandomVector
or an event of some kind, this method returns the value taken by the random vector if the root cause takes the value given as argument.- Parameters:
- fixedPoint
Point
Point chosen as the root cause of the random vector.
- fixedPoint
- Returns:
- realization
Point
The realization corresponding to the chosen root cause.
- realization
Examples
>>> import openturns as ot >>> distribution = ot.Normal() >>> randomVector = ot.RandomVector(distribution) >>> f = ot.SymbolicFunction('x', 'x') >>> compositeRandomVector = ot.CompositeRandomVector(f, randomVector) >>> event = ot.ThresholdEvent(compositeRandomVector, ot.Less(), 0.0) >>> print(event.getFrozenRealization([0.2])) [0] >>> print(event.getFrozenRealization([-0.1])) [1]
- getFrozenSample(fixedSample)¶
Compute realizations of the RandomVector.
In the case of a
CompositeRandomVector
or an event of some kind, this method returns the different values taken by the random vector when the root cause takes the values given as argument.- Parameters:
- fixedSample
Sample
Sample of root causes of the random vector.
- fixedSample
- Returns:
- sample
Sample
Sample of the realizations corresponding to the chosen root causes.
- sample
Examples
>>> import openturns as ot >>> distribution = ot.Normal() >>> randomVector = ot.RandomVector(distribution) >>> f = ot.SymbolicFunction('x', 'x') >>> compositeRandomVector = ot.CompositeRandomVector(f, randomVector) >>> event = ot.ThresholdEvent(compositeRandomVector, ot.Less(), 0.0) >>> print(event.getFrozenSample([[0.2], [-0.1]])) [ y0 ] 0 : [ 0 ] 1 : [ 1 ]
- getFunction()¶
Accessor to the Function in case of a composite RandomVector.
- Returns:
- function
Function
Function used to define a
CompositeRandomVector
as the image through this function of the antecedent:
.
- function
- getId()¶
Accessor to the object’s id.
- Returns:
- idint
Internal unique identifier.
- getImplementation()¶
Accessor to the underlying implementation.
- Returns:
- implImplementation
A copy of the underlying implementation object.
- getMarginal(*args)¶
Get the random vector corresponding to the
marginal component(s).
- Parameters:
- iint or list of ints,
Indicates the component(s) concerned.
is the dimension of the RandomVector.
- iint or list of ints,
- Returns:
- vector
RandomVector
RandomVector restricted to the concerned components.
- vector
Notes
Let’s note
a random vector and
a set of indices. If
is a
UsualRandomVector
, the subvector is defined by. If
is a
CompositeRandomVector
, defined bywith
,
some scalar functions, the subvector is
.
Examples
>>> import openturns as ot >>> distribution = ot.Normal([0.0, 0.0], [1.0, 1.0], ot.CorrelationMatrix(2)) >>> randomVector = ot.RandomVector(distribution) >>> ot.RandomGenerator.SetSeed(0) >>> print(randomVector.getMarginal(1).getRealization()) [0.608202] >>> print(randomVector.getMarginal(1).getDistribution()) Normal(mu = 0, sigma = 1)
- getMean()¶
Accessor to the mean of the RandomVector.
- Returns:
- mean
Point
Mean of the considered
UsualRandomVector
.
- mean
Examples
>>> import openturns as ot >>> distribution = ot.Normal([0.0, 0.5], [1.0, 1.5], ot.CorrelationMatrix(2)) >>> randomVector = ot.RandomVector(distribution) >>> ot.RandomGenerator.SetSeed(0) >>> print(randomVector.getMean()) [0,0.5]
- getName()¶
Accessor to the object’s name.
- Returns:
- namestr
The name of the object.
- getOperator()¶
Accessor to the comparaison operator of the Event.
- Returns:
- operator
ComparisonOperator
Comparaison operator used to define the
RandomVector
.
- operator
- getParameter()¶
Accessor to the parameter of the distribution.
- Returns:
- parameter
Point
Parameter values.
- parameter
- getParameterDescription()¶
Accessor to the parameter description of the distribution.
- Returns:
- description
Description
Parameter names.
- description
- getRealization()¶
Compute one realization of the RandomVector.
- Returns:
- realization
Point
Sequence of values randomly determined from the RandomVector definition. In the case of an event: one realization of the event (considered as a Bernoulli variable) which is a boolean value (1 for the realization of the event and 0 else).
- realization
See also
Examples
>>> import openturns as ot >>> distribution = ot.Normal([0.0, 0.0], [1.0, 1.0], ot.CorrelationMatrix(2)) >>> randomVector = ot.RandomVector(distribution) >>> ot.RandomGenerator.SetSeed(0) >>> print(randomVector.getRealization()) [0.608202,-1.26617] >>> print(randomVector.getRealization()) [-0.438266,1.20548]
- getSample(size)¶
Compute realizations of the RandomVector.
- Parameters:
- nint,
Number of realizations needed.
- nint,
- Returns:
- realizations
Sample
n sequences of values randomly determined from the RandomVector definition. In the case of an event: n realizations of the event (considered as a Bernoulli variable) which are boolean values (1 for the realization of the event and 0 else).
- realizations
Examples
>>> import openturns as ot >>> distribution = ot.Normal([0.0, 0.0], [1.0, 1.0], ot.CorrelationMatrix(2)) >>> randomVector = ot.RandomVector(distribution) >>> ot.RandomGenerator.SetSeed(0) >>> print(randomVector.getSample(3)) [ X0 X1 ] 0 : [ 0.608202 -1.26617 ] 1 : [ -0.438266 1.20548 ] 2 : [ -2.18139 0.350042 ]
- getThreshold()¶
Accessor to the threshold of the Event.
- Returns:
- thresholdfloat
Threshold of the
RandomVector
.
- intersect(other)¶
Intersection of two events.
- Parameters:
- event
RandomVector
A composite event
- event
- Returns:
- event
RandomVector
Intersection event
- event
- isComposite()¶
Accessor to know if the RandomVector is a composite one.
- Returns:
- isCompositebool
Indicates if the RandomVector is of type Composite or not.
- isEvent()¶
Whether the random vector is an event.
- Returns:
- isEventbool
Whether it takes it values in {0, 1}.
- join(other)¶
Union of two events.
- Parameters:
- event
RandomVector
A composite event
- event
- Returns:
- event
RandomVector
Union event
- event
- setDescription(description)¶
Accessor to the description of the RandomVector.
- Parameters:
- descriptionstr or sequence of str
Describes the components of the RandomVector.
- setName(name)¶
Accessor to the object’s name.
- Parameters:
- namestr
The name of the object.
- setParameter(parameters)¶
Accessor to the parameter of the distribution.
- Parameters:
- parametersequence of float
Parameter values.
Examples using the class¶
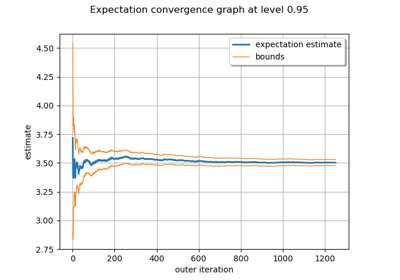
Evaluate the mean of a random vector by simulations
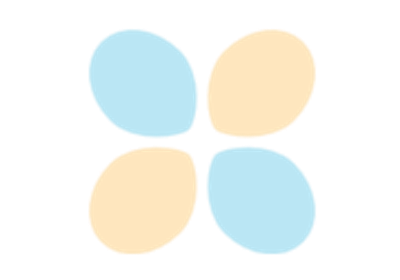
Use the Adaptive Directional Stratification Algorithm
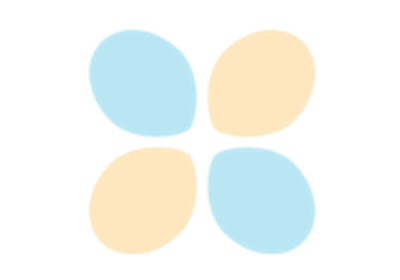
Use the post-analytical importance sampling algorithm
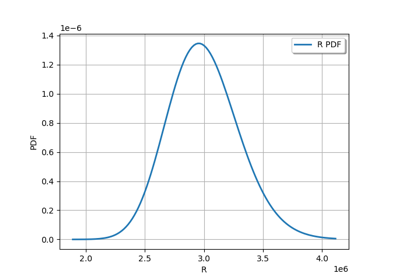
Estimate a probability with Monte-Carlo on axial stressed beam: a quick start guide to reliability
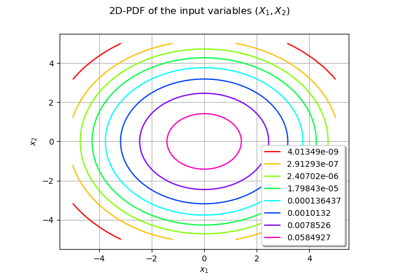
Use the FORM algorithm in case of several design points
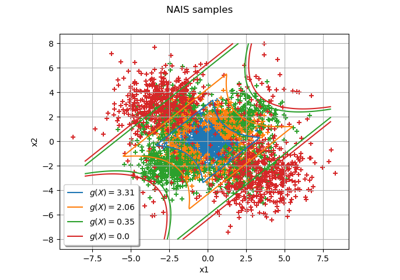
Non parametric Adaptive Importance Sampling (NAIS)
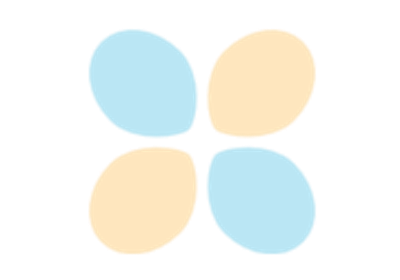
Test the design point with the Strong Maximum Test
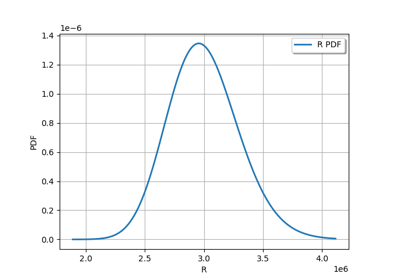
Axial stressed beam : comparing different methods to estimate a probability
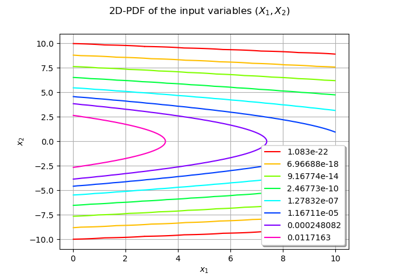
An illustrated example of a FORM probability estimate
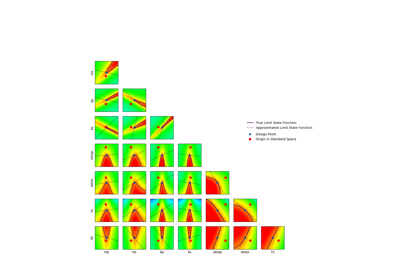
Using the FORM - SORM algorithms on a nonlinear function
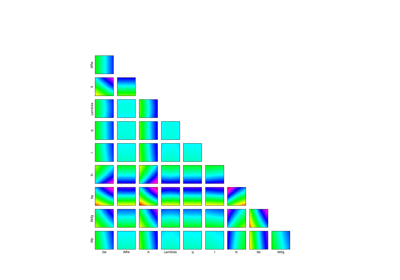
Example of sensitivity analyses on the wing weight model
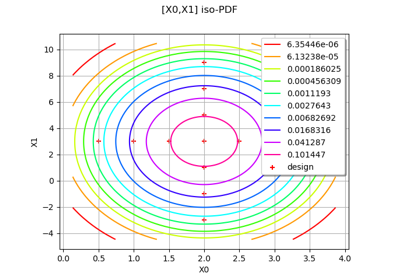
Create mixed deterministic and probabilistic designs of experiments
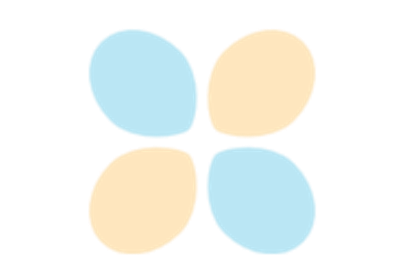
Defining Python and symbolic functions: a quick start introduction to functions
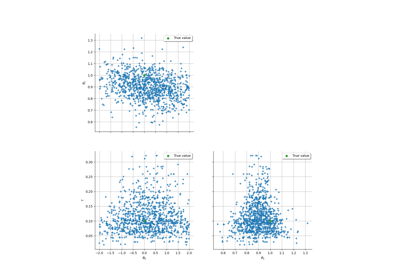
Linear Regression with interval-censored observations